Remove not alphanumeric characters from string
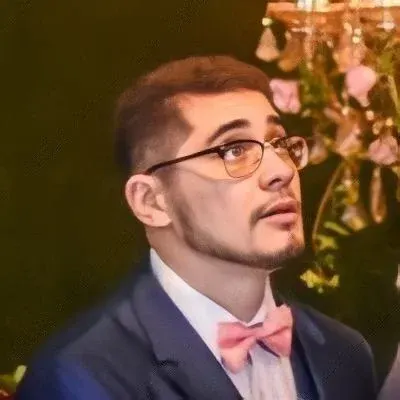
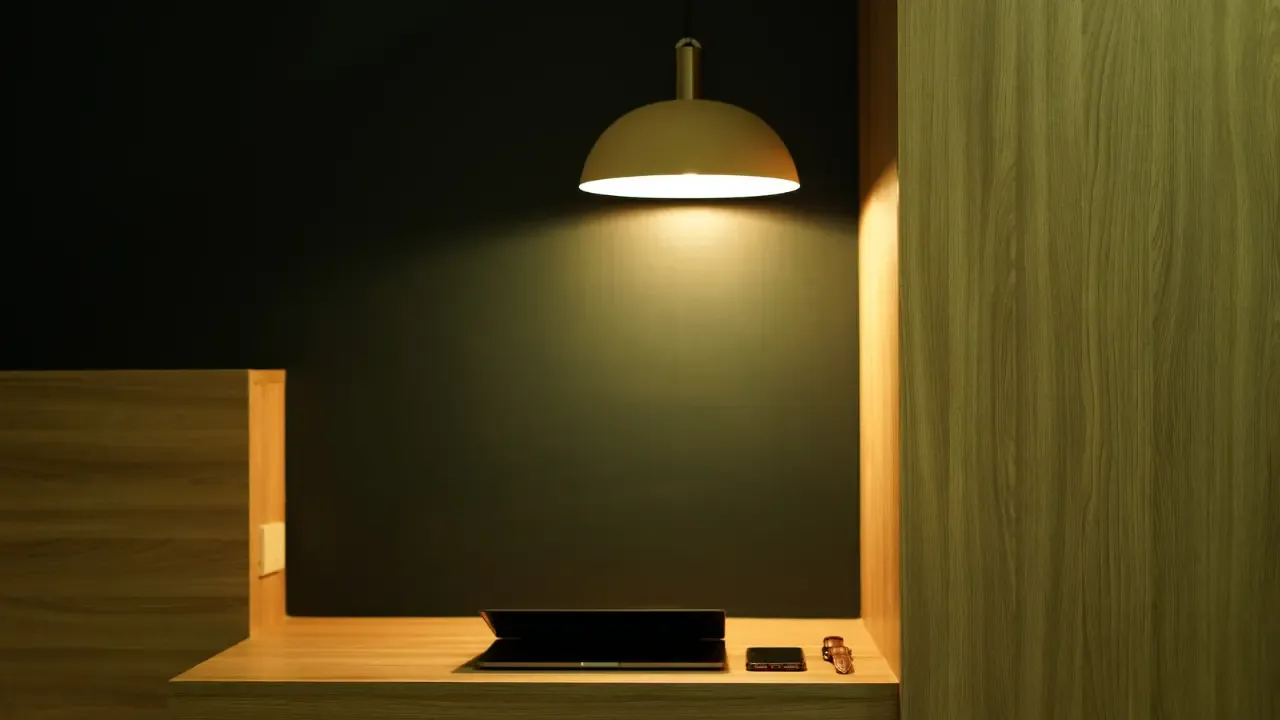
Removing Non-Alphanumeric Characters from a String: A Complete Guide
Are you tired of dealing with those pesky non-alphanumeric characters in your strings? 🤔 Don't worry, because we've got you covered! In this guide, we'll address the common issues faced when removing not alphanumeric characters from a string and provide easy solutions to help you achieve the desired output. 💪
The Challenge
Let's consider the following scenario:
Input: "\\test\red\bob\fred\new"
Output: "testredbobfrednew"
You may have noticed that simply using the replace
function with regular expressions isn't giving us the desired result. 😕
Attempted Solutions
Here are some attempts that have been made to solve this problem:
Attempt 1:
"\\test\red\bob\fred\new".replace(/[_\W]+/g, "");
Output: "testedobredew"
Attempt 2:
"\\test\red\bob\fred\new".replace(/['`~!@#$%^&*()_|+-=?;:'",.<>{}[\]\\/]/gi, "");
Output: "testedobred [newline] ew"
Attempt 3:
"\\test\red\bob\fred\new".replace(/[^a-zA-Z0-9]/, "");
Output: "testedobred [newline] ew"
Attempt 4:
"\\test\red\bob\fred\new".replace(/[^a-z0-9\s]/gi, '');
Output: "testedobred [newline] ew"
Multiple Steps Solution:
Another attempt involved multiple steps using a custom function:
function cleanID(id) {
id = id.toUpperCase();
id = id.replace( /\t/ , "T");
id = id.replace( /\n/ , "N");
id = id.replace( /\r/ , "R");
id = id.replace( /\b/ , "B");
id = id.replace( /\f/ , "F");
return id.replace( /[^a-zA-Z0-9]/ , "");
}
Output: "BTESTREDOBFREDNEW"
The Ultimate Solution
After going through several attempts, we finally found a working solution that meets all our requirements! 🎉
Final Attempt: JSON.stringify("\\test\red\bob\fred\new").replace(/\W/g, '');
Output: "testredbobfrednew"
By using JSON.stringify
to convert the string into a stringified format, we can then remove all non-alphanumeric characters using the replace
function with the regular expression /\\W/g
. This approach ensures that all special characters, including \r
, \n
, \b
, etc., are successfully removed from the string, giving us the desired output.
Wrap-Up
Removing not alphanumeric characters from a string can sometimes be a challenging task. However, armed with the right knowledge and solutions, you can easily overcome this problem. 💪
Now that you know the most effective solution, go ahead and try it out in your own projects. Don't hesitate to share your experience or any other alternative methods in the comments below! Let's conquer the world of alphanumeric strings together! 🌍💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
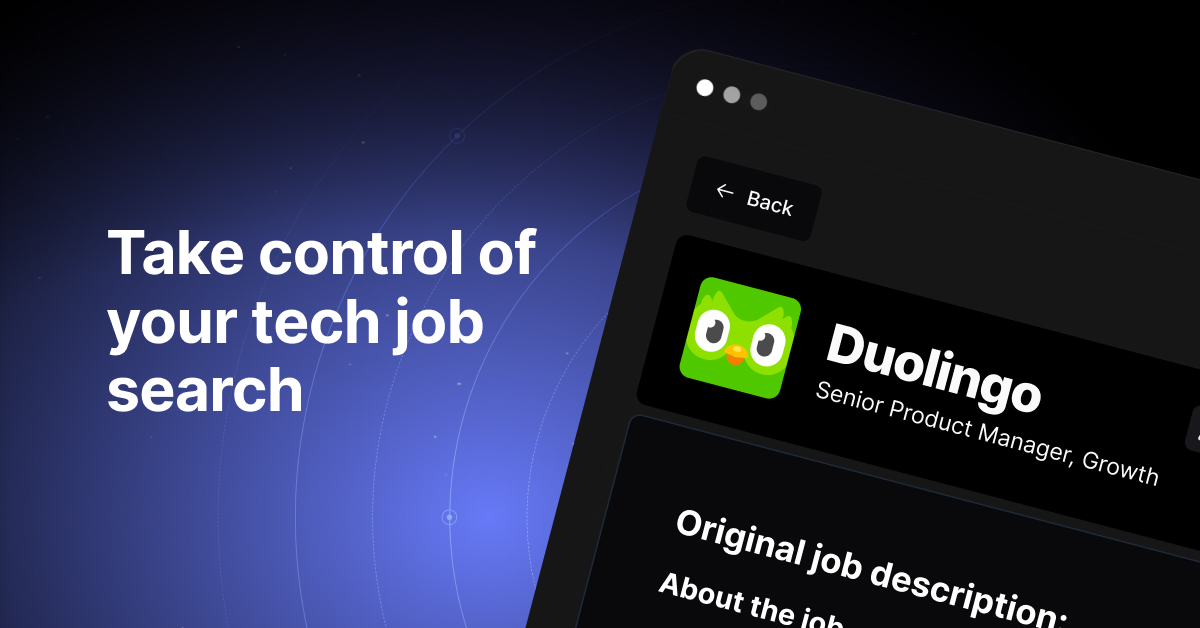