Remove last item from array
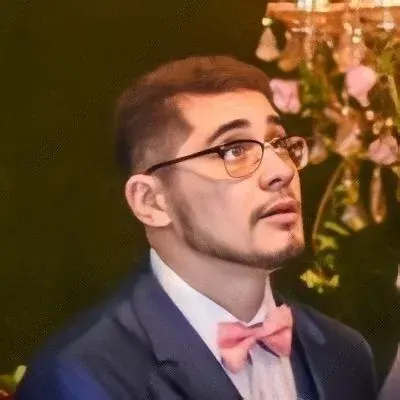
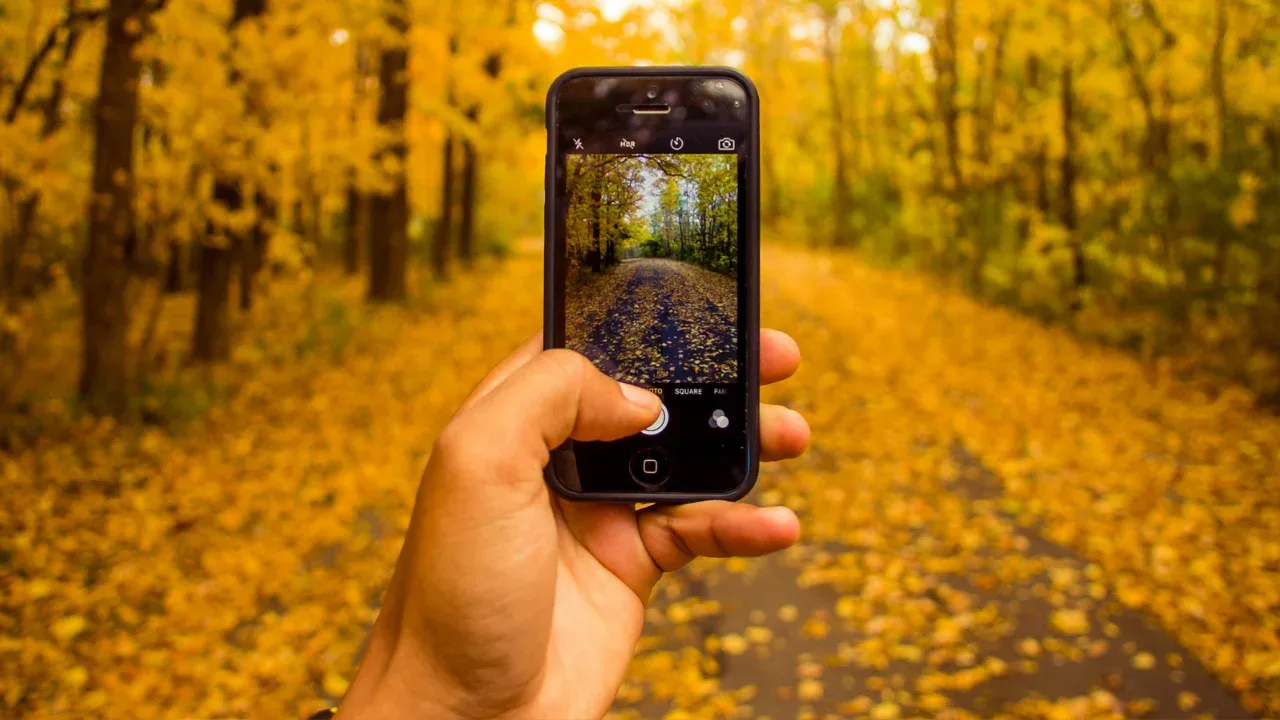
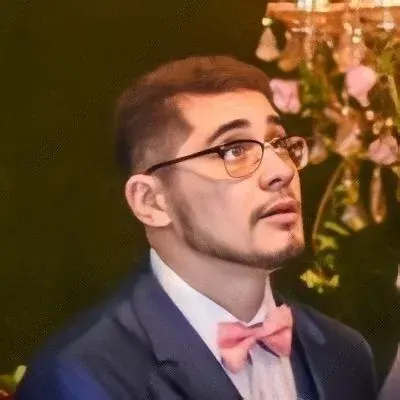
How to Remove the Last Item from an Array: Easy Solutions for an Annoying Problem š¤
š Hello there, fellow coders! Have you ever found yourself scratching your head š¤ over how to remove the last item from an array in your JavaScript code? Maybe you tried a solution that seemed promising, like using arr.slice(-1)
, only to be left wondering why it didn't work. Fear not, for today we will tackle this annoying problem head-on and provide you with some easy solutions! šŖ
The Situation
So, you have an array arr
filled with numbers: [1, 0, 2]
. You want to remove the last item from this array, which in this case is 2
. Simple, right? Well, not so fast! šļø
The Failed Attempt: arr.slice(-1)
You gave the arr.slice(-1)
method a try, thinking it would do the trick. But, much to your disappointment, it didn't actually remove the last element from the array. What gives? š«
Understanding arr.slice(-1)
Let's take a moment to understand what arr.slice(-1)
does. This method returns the last item of the array without modifying the original array. In other words, it creates a new array containing just the last element. This can be useful if you want to extract the last value without altering the original array, but it won't remove the item from the array itself.
Solution 1: arr.pop()
One way to remove the last item from an array is by using the arr.pop()
method. This method removes the last element from the array and returns that element. Here's how you can use it to solve your problem:
var lastItem = arr.pop();
console.log(lastItem); // Output: 2
console.log(arr); // Output: [1, 0]
By assigning the result of arr.pop()
to a variable (lastItem
in this case), you can retrieve the value of the last item before it was removed. And voila! The element is successfully removed, and your array is updated without the last item. š
Solution 2: arr.splice()
Another option to remove the last item from an array is by using the arr.splice()
method. This method allows you to remove elements from an array by specifying the starting index and the number of elements to remove. Here's how you can do it:
arr.splice(-1);
console.log(arr); // Output: [1, 0]
By passing -1
as the starting index to arr.splice()
, we tell it to start removing elements from the last position. Since we don't specify the number of elements to remove, it will remove everything from the starting index to the end of the array. As a result, the last item is eliminated, and your array is left with just [1, 0]
. š
š¢ Time for Action!
Now that you know how to remove the last item from an array, it's time to put your newfound knowledge into practice! Head over to your code editor and give it a try. Remember, the journey to becoming a great coder is paved with hands-on experience. š»
Conclusion
Removing the last item from an array may seem like a tricky task at first, but with the right knowledge, it becomes a piece of cake! In this guide, we explored two easy solutions: using arr.pop()
and arr.splice(-1)
. These methods provide different approaches, allowing you to choose the one that fits your specific needs. So go ahead and unleash your coding powers, and never let a stubborn array stand in your way again! š„
We hope you found this blog post helpful! If you have any further questions or want to share your thoughts, we'd love to hear from you in the comments section below. And don't forget to hit that share button to help your fellow coders tackle this irritating array problem with ease! šāØ