Remove duplicate values from JS array
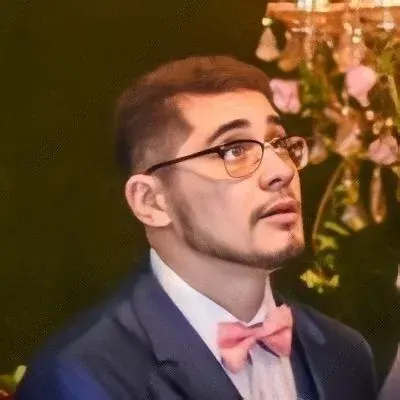
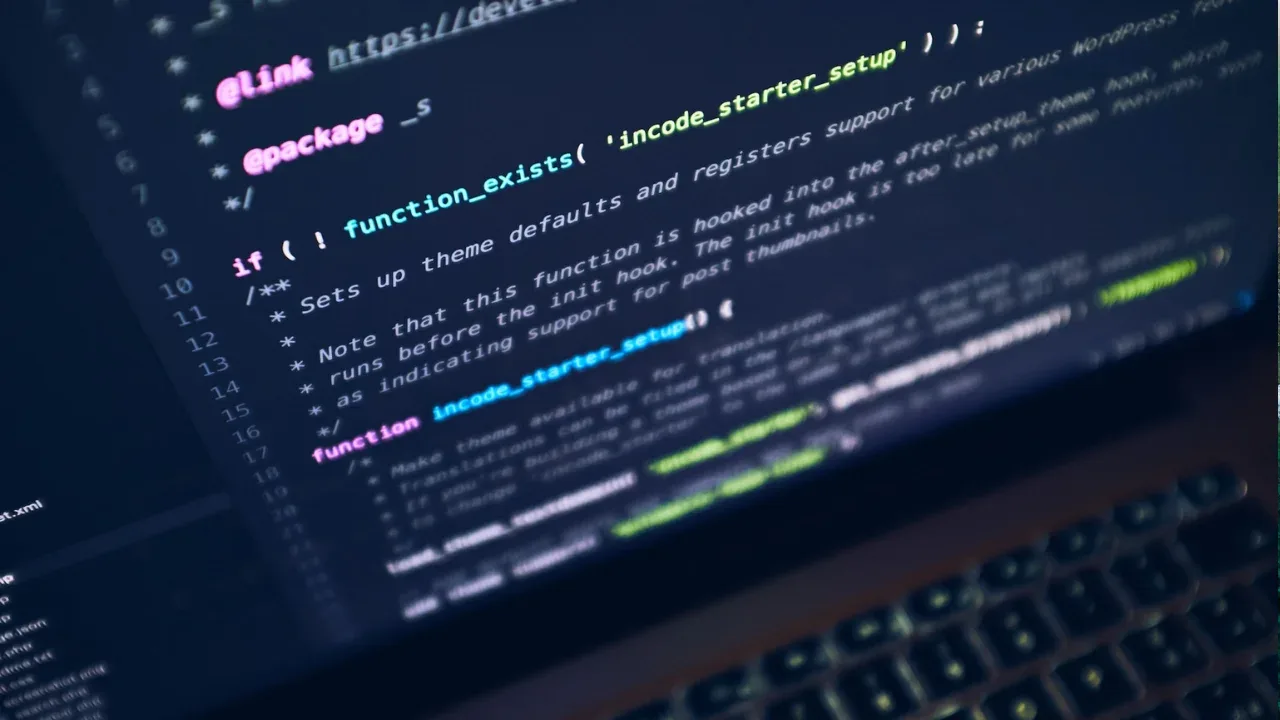
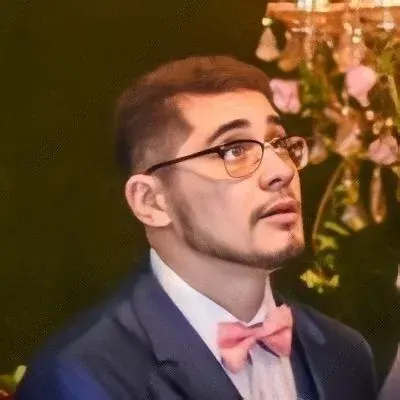
Removing Duplicate Values from JavaScript Array: A Quick Guide
Are you struggling to remove duplicate values from a JavaScript array? Look no further! In this blog post, we'll address this common issue and provide you with easy solutions. Whether you're a beginner or an experienced developer, we've got you covered. So, let's dive in and get those duplicates out of your array! 😎
The Problem: Removing Duplicates from an Array
Imagine you have a simple JavaScript array like this:
var names = ["Mike", "Matt", "Nancy", "Adam", "Jenny", "Nancy", "Carl"];
You want to remove the duplicate values and store only the unique ones in a new array. However, your initial attempts at solving this problem may not have been successful. Frustrating, right? 😤
Easy Solutions: Removing Duplicates Made Simple
Worry not, fellow developer! We've got a few easy solutions for you. Let's explore each of them, step by step.
Solution 1: Using Set
JavaScript provides a built-in data structure called the Set, which allows us to store unique values. We can leverage this feature to remove duplicates. Here's how you can do it:
var uniqueNames = [...new Set(names)];
That's it! By spreading the set into an array, you'll get only the unique values. How cool is that? 😃
Solution 2: Using Filter and Index Of
Another approach involves using the filter
method along with the indexOf
method. This solution checks for the index of each element and keeps only the first occurrence. Here's how you can implement it:
var uniqueNames = names.filter((name, index) => {
return names.indexOf(name) === index;
});
Voila! The filter
method creates a new array containing only the elements that pass the provided condition. In this case, we compare the current element's index with the first occurrence of that element. This removes any duplicates and gives us a unique array.
Solution 3: Using jQuery (optional)
If you prefer using jQuery, no problemo! You can achieve the same result with its help. Here's how:
var uniqueNames = $.unique(names);
By invoking the $.unique
function, you'll get an array with only the unique values. Simple and effective! 😄
Your Turn: Let's Engage!
Now that you've learned these easy solutions, it's time to put your skills to the test. Try them out and let us know your thoughts!
Have you encountered any other methods for removing duplicates from a JavaScript array? We'd love to hear about them! Share your experiences, thoughts, and suggestions in the comments section below. Let's learn from each other and grow together as a tech community! 💪🌟
Similar Question and Community Resources
Still hungry for more knowledge? Here's a similar question on Stack Overflow that you can explore:
Don't forget to check out the answers and solutions provided by the community. The more resources, the merrier! 🎉
That's a wrap for today! We hope you found this guide helpful and engaging. Stay tuned for more exciting tech tips, tricks, and tutorials on our blog. Until then, happy coding! 🚀✨