Remove CSS class from element with JavaScript (no jQuery)
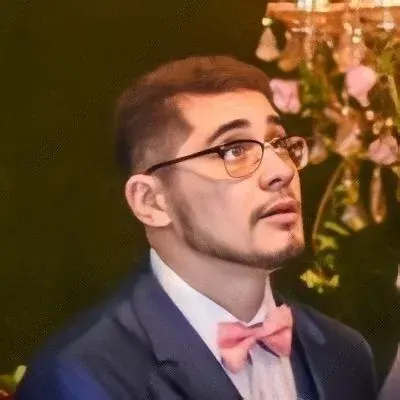
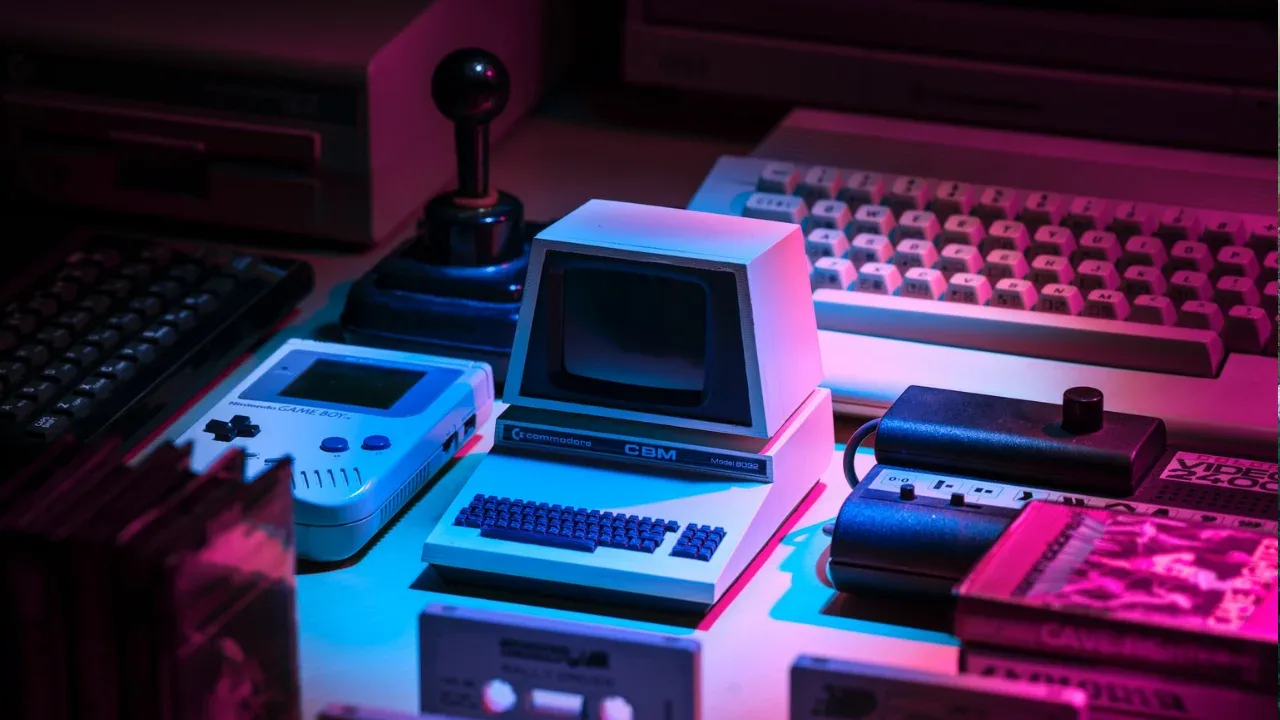
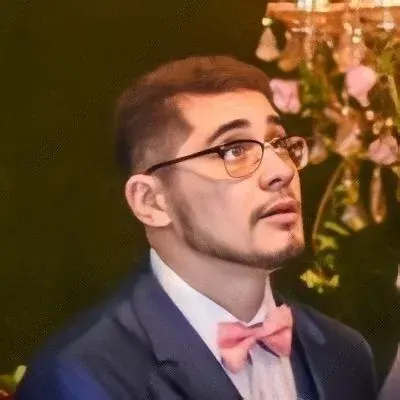
Removing CSS Class from Element with JavaScript (No jQuery)
So you want to remove a CSS class from an element using pure JavaScript. No worries! We've got you covered. In this blog post, we'll walk you through the process step-by-step, provide easy-to-understand solutions, and answer any questions you may have. Let's dive in! 🚀
The Problem
The first thing we need to tackle is understanding the problem at hand. Removing a CSS class from an element is a common task for web developers, but it can be confusing if you're new to JavaScript. Luckily, with a little bit of know-how, we can achieve this without relying on jQuery.
The Solution
There are a few different approaches we can take to remove a CSS class from an element using JavaScript. Let's explore each one in detail:
1. Using the classList
Property
The classList
property is a powerful tool that allows us to add, remove, or toggle CSS classes on an element. To remove a class, you can use the classList.remove()
method. Here's an example:
const element = document.getElementById('yourElementId');
element.classList.remove('yourClassName');
In the above code snippet, replace 'yourElementId'
with the ID of the element you want to target, and 'yourClassName'
with the name of the class you want to remove.
2. Using the className
Property
If you're working with older browsers that don't support the classList
property, don't worry! You can still achieve the desired result using the className
property. Here's how you can do it:
const element = document.getElementById('yourElementId');
const classToRemove = 'yourClassName';
if (element.classList) {
element.classList.remove(classToRemove);
} else {
let classes = element.className.split(' ');
let index = classes.indexOf(classToRemove);
if (index > -1) {
classes.splice(index, 1);
element.className = classes.join(' ');
}
}
The above code snippet provides a fallback solution for browsers that don't support classList
. It splits the className
into an array, finds the index of the class to be removed, removes it using splice()
, and then joins the modified array back into a string.
Summary
Removing a CSS class from an element with JavaScript is totally doable, even without jQuery! By using either the classList
or className
property, you can easily manipulate CSS classes and achieve the desired result.
Your Turn!
We hope this guide has helped you understand how to remove a CSS class from an element with JavaScript. Now it's your turn to try it out and apply it to your own projects. If you have any questions or need further assistance, feel free to leave a comment below.
Happy coding! 🎉