Remove all special characters with RegExp
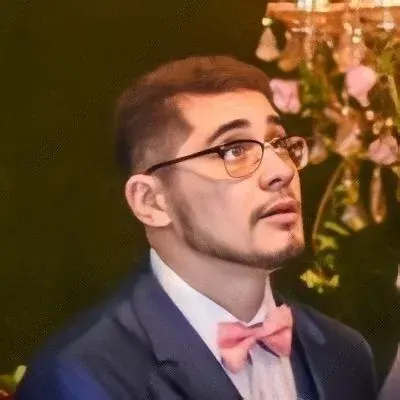
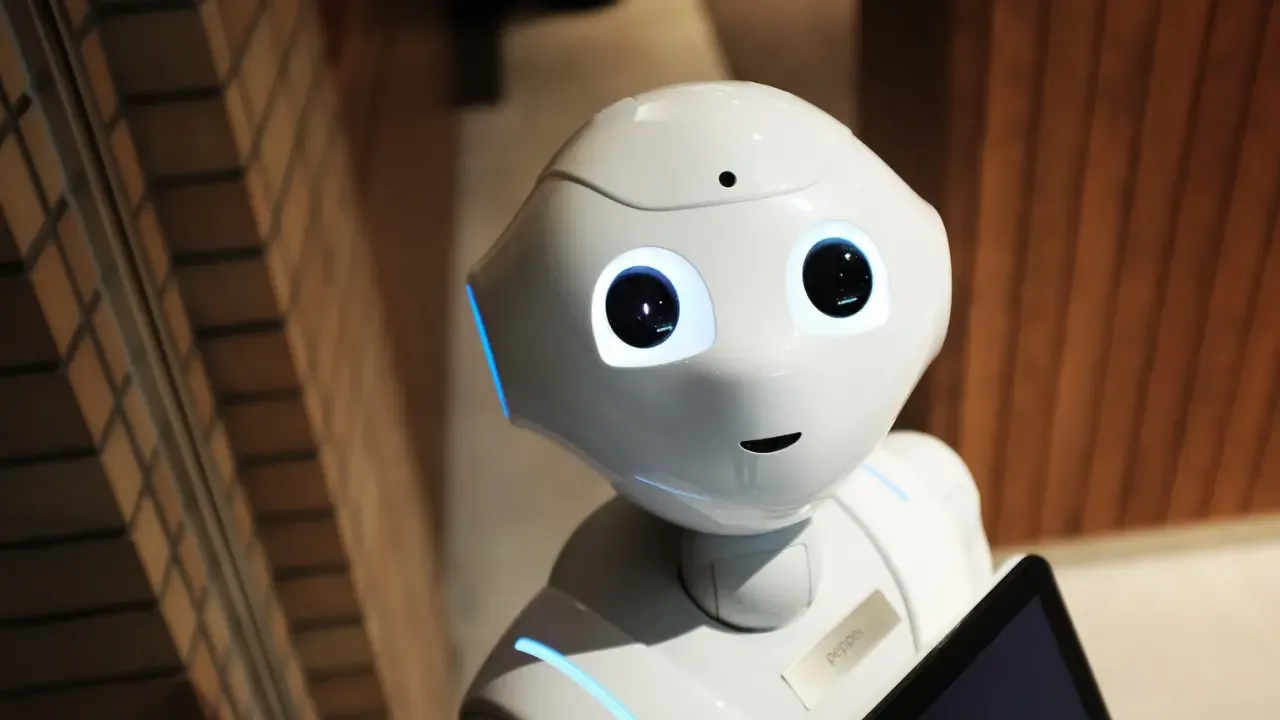
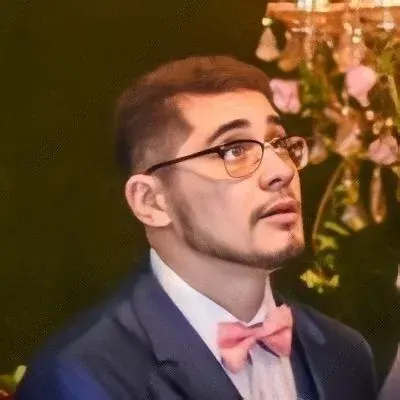
Removing Special Characters with RegExp: The Ultimate Guide 🧩
Have you ever encountered a situation where you needed to remove special characters from a string? If you've been scratching your head trying to find a solution, worry no more! In this blog post, we'll explore the common issues surrounding removing special characters with RegExp and provide easy, cross-browser compatible solutions. Let's dive in! 💪
The Problem: Incompatibility in IE7 😱
The context for this question revolves around a specific scenario where a RegExp is used to remove special characters from a string. The provided code works perfectly fine in Firefox, but it fails to do the job in IE7. So, what's the catch? 🤔
<pre><code>var specialChars = "!@#$^&%*()+=-[]\/{}|:<>?,."; for (var i = 0; i < specialChars.length; i++) { stringToReplace = stringToReplace.replace(new RegExp("\\" + specialChars[i], "gi"), ""); } </code></pre>
Solution: Overcoming Compatibility Issues in IE7 🛠️
To ensure cross-browser compatibility, we need to modify our approach. Rather than using the backslash (\
) to escape special characters, we'll rely on character classes to match the special characters. This change guarantees consistent behavior across browsers, including IE7. 🌐
Here's an updated version of the code snippet:
var specialChars = "!@#$^&%*()+=-[]\/{}|:<>?,.";
var regexPattern = new RegExp("[" + specialChars.replace(/([.*+?^=!:${}()|\[\]\/\\])/g, '\\$1') + "]", "gi");
stringToReplace = stringToReplace.replace(regexPattern, "");
By enclosing the special characters in square brackets ([]
), we create a character class. Additionally, we use the .replace()
method with a regular expression to escape any special characters within the specialChars
string.
Understanding the RegExp 🤓
To demystify the RegExp used in our solution, let's break it down:
[" + specialChars.replace(/([.*+?^=!:${}()|\[\]\/\\])/g, '\\$1') + "]
: This part dynamically creates a character class by surrounding thespecialChars
string with square brackets. Thereplace()
method ensures that any special characters withinspecialChars
are correctly escaped."gi"
: These are the flags passed to the RegExp constructor. 'g' stands for global (replaces all occurrences), and 'i' stands for case-insensitive (matches regardless of case).
Conclusion: Embrace the Power of RegExp! ✨
Removing special characters from a string may seem tricky at first, especially with the compatibility issues in older browsers. However, armed with our easy-to-understand solution, you can tackle this problem head-on! Remember to use character classes for consistent cross-browser behavior and escape any special characters.
If you found this guide helpful or have any additional tips/tricks, 🎉 let's continue the conversation in the comments section! Your engagement fuels our motivation to create more useful content. Happy coding! 🚀🔥