Remove a JSON attribute
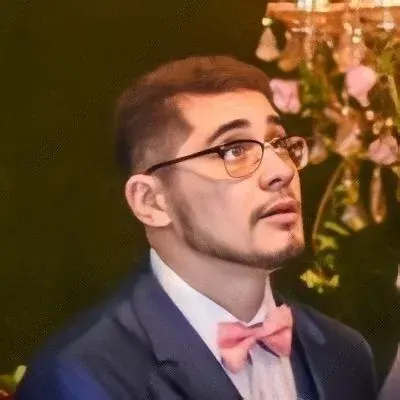
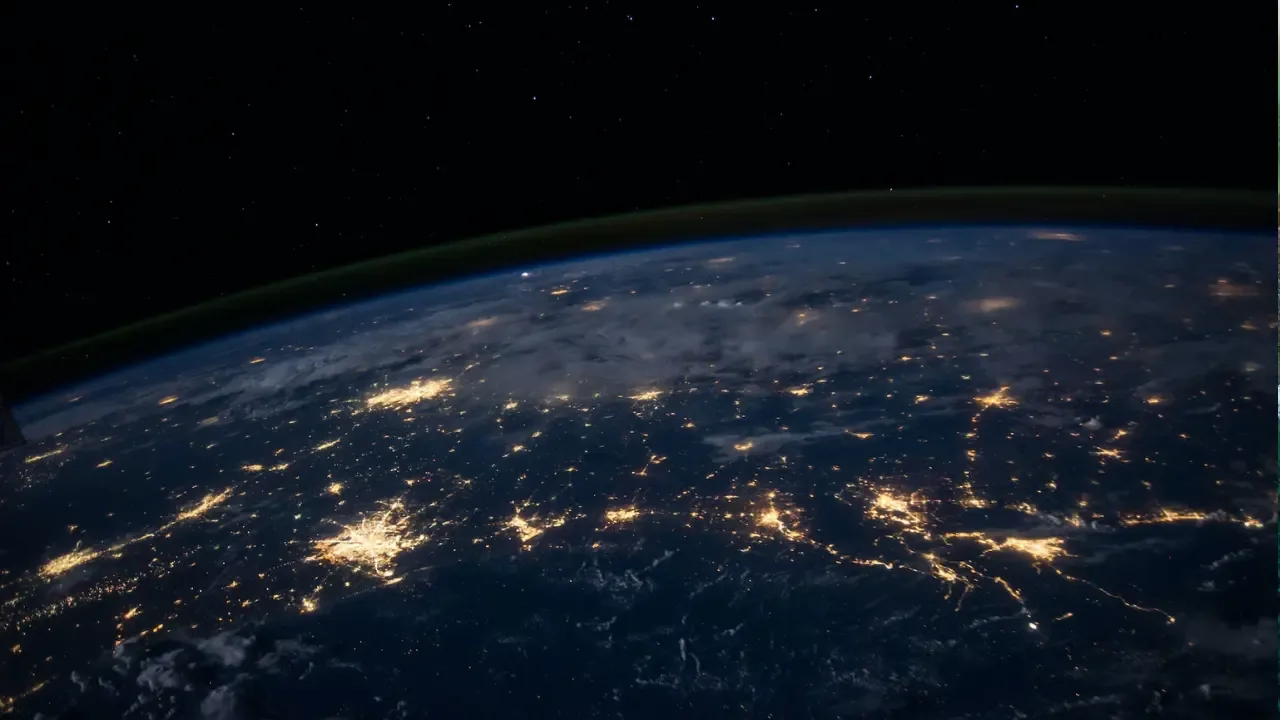
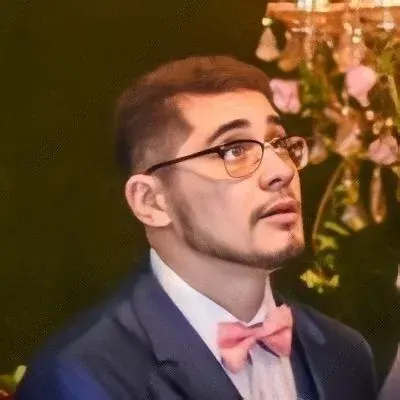
📝 Tech Blog: Removing a JSON Attribute Made Easy
Welcome, tech enthusiasts! 👋 In today's blog post, we'll tackle a common and sometimes puzzling question: how to remove a JSON attribute. Whether you're a seasoned developer or just starting your coding journey, we've got your back. We'll provide easy-to-understand solutions and examples to help you effortlessly remove that unwanted attribute. Let's dive in! 💪
Understanding the Challenge
So, you have a JSON object, and you want to remove a specific attribute. Let's take an example to make things crystal clear. Consider the following JSON object:
var myObj = {'test' : {'key1' : 'value', 'key2': 'value'}}
You want to remove the attribute 'key1'
, resulting in the following JSON object:
{'test': {'key2': 'value'}}
Solution 1: Using JavaScript's delete
Keyword
The delete
keyword in JavaScript can be used to remove properties from an object, including attributes of a JSON object. Here's how you can use it to remove the 'key1'
attribute from myObj
:
delete myObj.test.key1;
In this approach, we directly access the attribute we want to remove using dot notation and delete
it. Simple and clean, right? 😊
Solution 2: Using lodash.omit
If you're using the fantastic JavaScript utility library called Lodash, removing a JSON attribute becomes even easier with the omit
function. First, make sure you have Lodash installed. Then, you can remove 'key1'
from myObj
like this:
const _ = require('lodash');
const modifiedObj = _.omit(myObj, ['test.key1']);
By passing the path of the attribute to exclude, omit
creates a new object without the specified attribute. It's a superb option if you're already using Lodash in your project. 🙌
Solution 3: Using the Spread Operator
If you prefer to use modern JavaScript syntax, the spread operator (...
) offers an elegant solution. Here's how you can use it to remove the 'key1'
attribute:
const { ['key1']: _, ...modifiedObj } = myObj.test;
myObj.test = modifiedObj;
By using destructuring assignment and the spread operator, we create a new object modifiedObj
without the 'key1'
attribute. Finally, we update myObj
with the modified object. Fancy, isn't it? 😎
Share Your Thoughts!
Removing a JSON attribute may seem like a small task, but it can cause headaches if you're unfamiliar with the solutions. We hope our guide has shed some light on this topic and empowered you to tackle this challenge with confidence. Share your favorite solution or ask questions in the comments below! Let's learn and grow together. 🌱✨
Conclusion
We've explored three straightforward solutions to remove a JSON attribute:
Using JavaScript's
delete
keywordLeveraging the
omit
function from the Lodash libraryUtilizing the spread operator with destructuring assignment
Remember to choose the solution that best suits your project and coding style.
If you found this blog post helpful, give it a big thumbs up 👍 and share it with your fellow developers. Stay tuned for more engaging content and useful tips! Happy coding! 💻🚀