Regular Expression to get a string between parentheses in Javascript
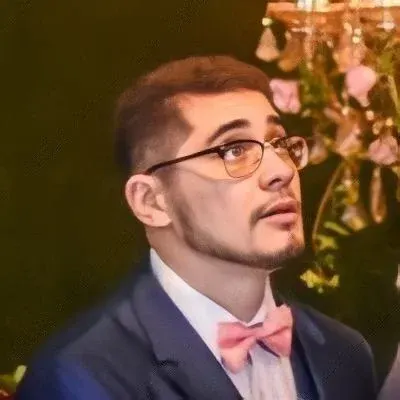
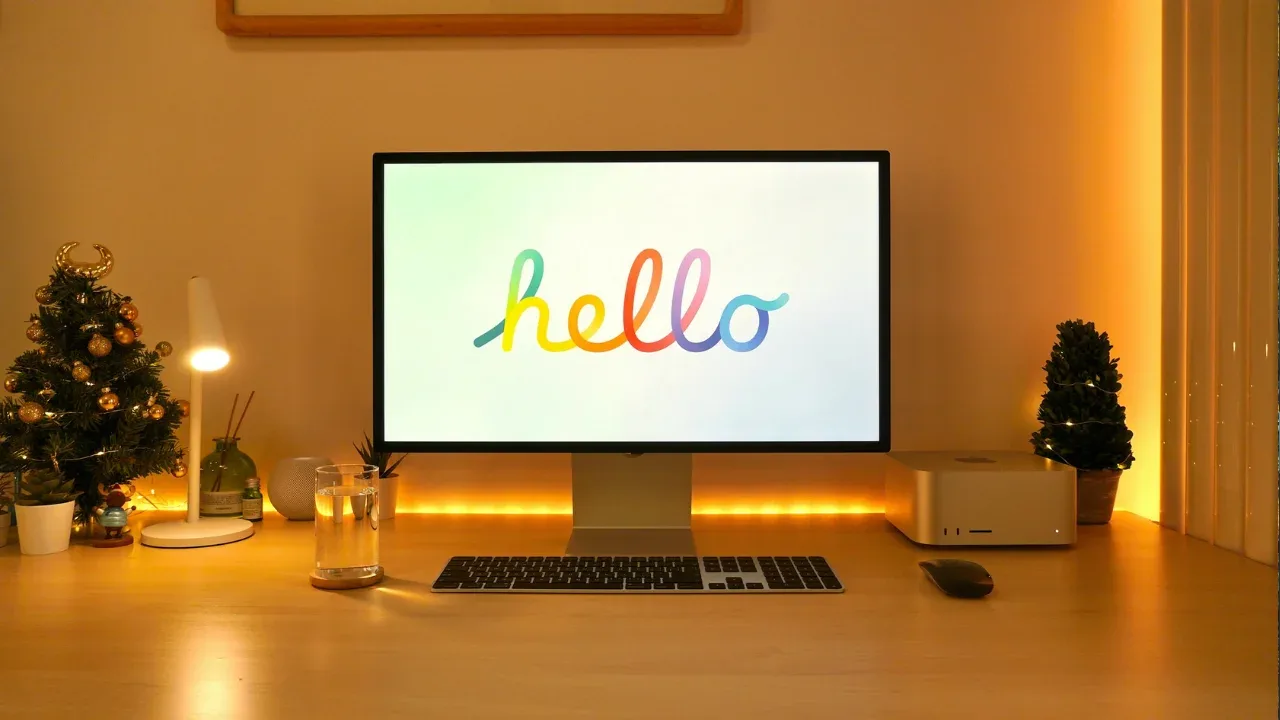
Extracting a String between Parentheses in JavaScript 📜
Have you ever encountered a situation where you wanted to extract the string between parentheses in JavaScript? 🤔 Fear not! In this article, we'll dive deep into this topic and provide you with easy solutions that will make your life easier. 😄
🎯 The Problem
Let's say you have a string and you only want to retrieve the content that resides between the opening "(" and closing ")" parentheses. For example, given the following string:
I expect five hundred dollars ($500).
You want to extract the text "$500" which is between the parentheses.
💡 The Solution
To achieve this, we can leverage one of JavaScript's powerful tools called regular expressions (regex). A regex is a sequence of characters that forms a search pattern, and it's perfect for extracting specific patterns or substrings from a text.
Here's how you can write a regular expression to get the string between parentheses:
const regex = /\(([^)]+)\)/;
const str = "I expect five hundred dollars ($500).";
const matches = str.match(regex);
const result = matches ? matches[1] : null;
Let's break down the regex pattern /\(([^)]+)\)/
:
\(
: Matches the opening parenthesis "(".([^)]+)
: Captures one or more characters that are not a closing parenthesis ")".\)
: Matches the closing parenthesis ")".
By using the match
function on the string str
with our regex pattern, we store the result in the matches
array. The captured string between the parentheses will be stored in matches[1]
. If no match is found, we set the result
variable to null
.
For example, running the code above will give you the result:
$500
⚠️ Common Pitfalls
When working with regular expressions, there are a few things you should keep in mind:
Escape Characters: When using characters with special meaning in regex (like parentheses), you need to escape them with a backslash "\". This is why we used
/\(
and\)
in our regex pattern.Single Match: The given solution only works when there is a single instance of parentheses in the string. If there are multiple occurrences, you might need to modify the regex pattern or use a different approach.
🚀 Call-to-Action
Now that you know how to extract strings between parentheses in JavaScript, try applying it to your own projects! Experiment with different strings and see how it can simplify your code. If you have any questions or need further help, leave a comment below or visit our friendly community at Stack Overflow.
Keep exploring, coding, and never stop learning! 🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
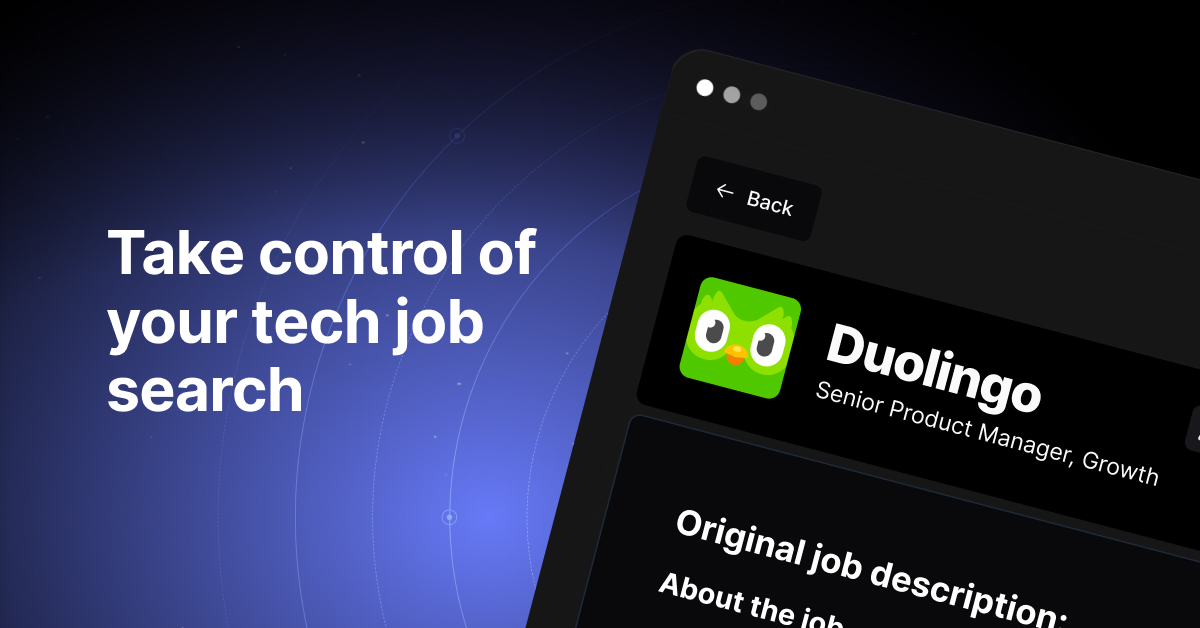