Regex to replace multiple spaces with a single space
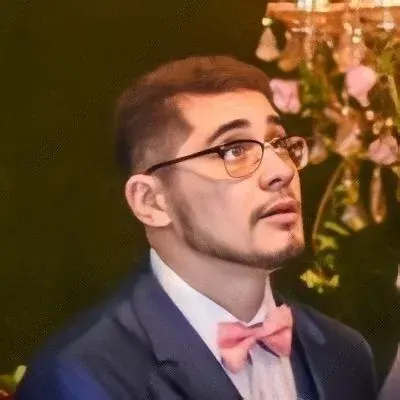
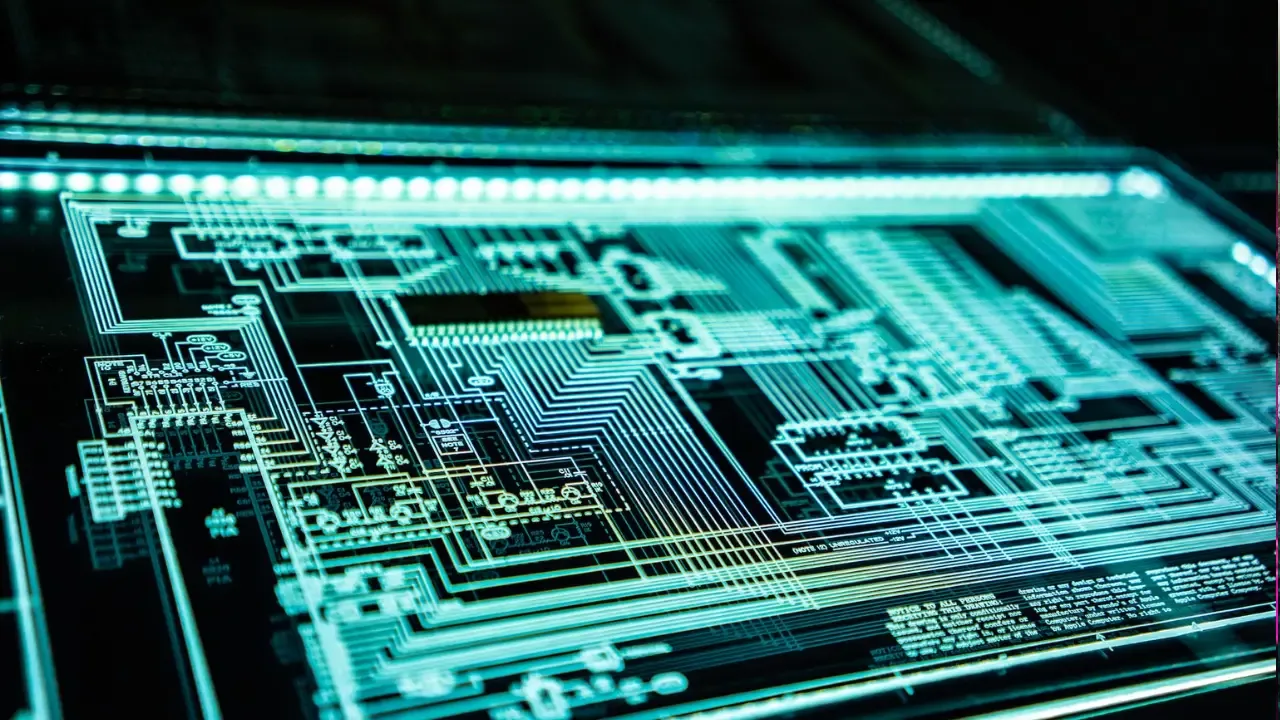
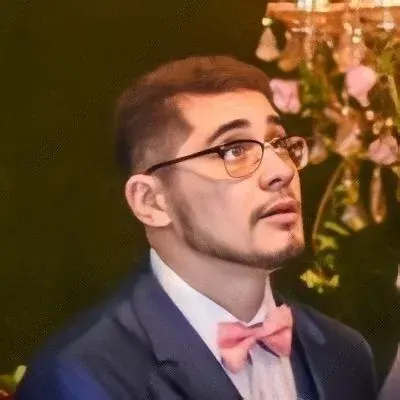
Easy Peasy Way to Replace Multiple Spaces with a Single Space using Regex in JavaScript ππ
Are you tired of dealing with pesky extra spaces cluttering your text? π Whether you're working on a form validation, data manipulation, or simply trying to make your text look neat and tidy, the struggle is real! But worry no more, because we've got the perfect jQuery or JavaScript magic to help you out! π©β¨
The Problem: Extra Spaces Everywhere! π«
Imagine you have a string with multiple spaces, like this:
"The dog has a long tail, and it is RED!"
All those unnecessary spaces make the text look messy and unprofessional! You want to transform it into a clean and polished string with only a single space between each word, like this:
"The dog has a long tail, and it is RED!"
The Solution: Regex to the Rescue! π¦ΈββοΈ
We can use a regular expression (regex) combined with JavaScript's replace()
method to effortlessly eliminate those surplus spaces! πͺπ
const text = "The dog has a long tail, and it is RED!";
const updatedText = text.replace(/\s+/g, " ");
console.log(updatedText);
By using the regex /\s+/g
, we're targeting any consecutive whitespace characters \s+
in the text. The g
flag ensures that all occurrences are replaced, not just the first one. The second argument to replace()
is " "
, which represents the single space we want to replace the multiple spaces with.
Test Drive: Let's See It in Action! ππ¨
const text = "The dog has a long tail, and it is RED!";
const updatedText = text.replace(/\s+/g, " ");
console.log(updatedText);
Output:
"The dog has a long tail, and it is RED!"
So satisfying, right? Those excessive spaces are now gone, leaving you with a sleek and streamlined string! πβ¨
Go Beyond: Customizing Your Regex! π
The great thing about using regex is its flexibility! π§ You can tweak it to fit your specific needs and address a variety of space-related scenarios.
For example, if you only want to remove extra spaces at the beginning or end of the string, you can modify the regex pattern like this:
const text = " Only trim leading and trailing spaces ";
const updatedText = text.replace(/^\s+|\s+$/g, "");
console.log(updatedText);
Output:
"Only trim leading and trailing spaces"
In this regex, ^\s+
matches any whitespaces at the beginning of the string, and \s+$
matches any whitespaces at the end. Combining them with the |
operator allows us to remove only the leading and trailing spaces.
Feel free to explore and experiment with different patterns to suit your specific use case! π§ͺπ‘
It's Your Turn! ππ
Now that you know the secret sauce for replacing multiple spaces with a single space using regex in JavaScript, it's time to put your newfound knowledge into action! Use it to clean up your text, improve user experiences, or conquer any other space-related challenges you encounter! β¨π
But hey, don't keep it to yourself! Share this guide with your friends and colleagues who might find it helpful too! Let's spread the word and make the web a tidier place, one space at a time! ππ»
Markdown Version:
# Easy Peasy Way to Replace Multiple Spaces with a Single Space using Regex in JavaScript ππ
Are you tired of dealing with pesky extra spaces cluttering your text? π Whether you're working on a form validation, data manipulation, or simply trying to make your text look neat and tidy, the struggle is real! But worry no more, because we've got the perfect jQuery or JavaScript magic to help you out! π©β¨
## The Problem: Extra Spaces Everywhere! π«
Imagine you have a string with multiple spaces, like this:
\`\`\`javascript
"The dog has a long tail, and it is RED!"
\`\`\`
All those unnecessary spaces make the text look messy and unprofessional! You want to transform it into a clean and polished string with only a single space between each word, like this:
\`\`\`javascript
"The dog has a long tail, and it is RED!"
\`\`\`
## The Solution: Regex to the Rescue! π¦ΈββοΈ
We can use a regular expression (regex) combined with JavaScript's \`replace()\` method to effortlessly eliminate those surplus spaces! πͺπ
\`\`\`javascript
const text = "The dog has a long tail, and it is RED!";
const updatedText = text.replace(/\\s+/g, " ");
console.log(updatedText);
\`\`\`
By using the regex \`/\\s+/g\`, we're targeting any consecutive whitespace characters \`\\s+\` in the text. The \`g\` flag ensures that all occurrences are replaced, not just the first one. The second argument to \`replace()\` is \`" "\`, which represents the single space we want to replace the multiple spaces with.
## Test Drive: Let's See It in Action! ππ¨
\`\`\`javascript
const text = "The dog has a long tail, and it is RED!";
const updatedText = text.replace(/\\s+/g, " ");
console.log(updatedText);
\`\`\`
Output:
\`\`\`
"The dog has a long tail, and it is RED!"
\`\`\`
So satisfying, right? Those excessive spaces are now gone, leaving you with a sleek and streamlined string! πβ¨
## Go Beyond: Customizing Your Regex! π
The great thing about using regex is its flexibility! π§ You can tweak it to fit your specific needs and address a variety of space-related scenarios.
For example, if you only want to remove extra spaces at the beginning or end of the string, you can modify the regex pattern like this:
\`\`\`javascript
const text = " Only trim leading and trailing spaces ";
const updatedText = text.replace(/^\\s+|\\s+$/g, "");
console.log(updatedText);
\`\`\`
Output:
\`\`\`
"Only trim leading and trailing spaces"
\`\`\`
In this regex, \`^\\s+\` matches any whitespaces at the beginning of the string, and \`\\s+$\` matches any whitespaces at the end. Combining them with the \`|\` operator allows us to remove only the leading and trailing spaces.
Feel free to explore and experiment with different patterns to suit your specific use case! π§ͺπ‘
## It's Your Turn! ππ
Now that you know the secret sauce for replacing multiple spaces with a single space using regex in JavaScript, it's time to put your newfound knowledge into action! Use it to clean up your text, improve user experiences, or conquer any other space-related challenges you encounter! β¨π
But hey, don't keep it to yourself! Share this guide with your friends and colleagues who might find it helpful too! Let's spread the word and make the web a tidier place, one space at a time! ππ»
Make sure you bookmark and share this blog post so you'll always have this handy regex trick up your sleeve! Happy coding! ππ»