RegEx to extract all matches from string using RegExp.exec
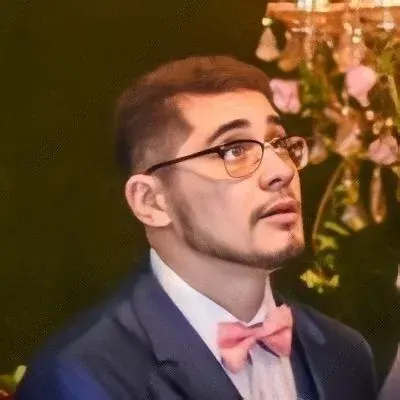
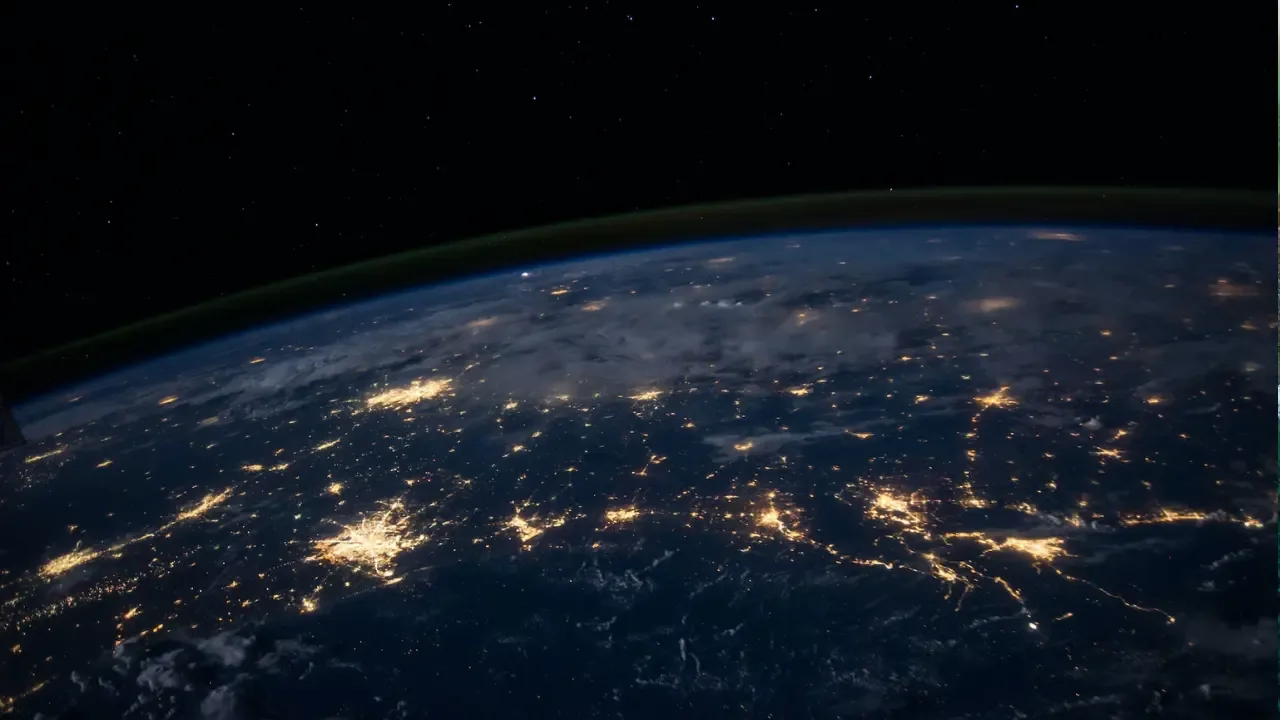
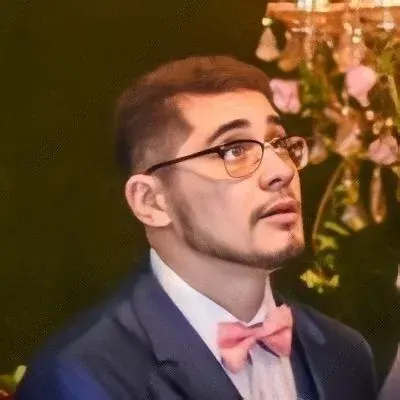
Extracting All Matches from a String using RegExp.exec
š So you want to extract all matches from a string using the RegExp.exec
method? Well, you've come to the right place! š In this blog post, we'll explain how to solve the common issue of only getting the first match, and provide you with an easy solution. Let's dive in! šŖ
The Problem šµļøāāļø
The issue at hand is that the RegExp.exec
method only returns the first match of a regular expression pattern within a string. In the provided code snippet, the desired result is to extract both the key name and its corresponding value from the string, but only the first match is returned.
The Solution š”
To extract all matches from a string, we can modify our approach slightly by using a loop along with the RegExp.exec
method. Here's an updated code snippet:
const inputString = '[description:"aoeu" uuid:"123sth"]';
const regex = /(\w+?):"(.*?)"/g;
let match;
const matches = [];
while ((match = regex.exec(inputString)) !== null) {
matches.push({
key: match[1],
value: match[2]
});
}
console.log(matches);
šÆ The crucial change here is the use of a while loop that continues to call regex.exec
until all matches have been found. Each match is then pushed into an array named matches
as an object with key
and value
properties.
š” In our regular expression pattern, (\w+?)
captures the key name, and "(.*?)"
captures the value. The g
flag at the end enables global matching.
Let's Break it Down š
We define the input string
'[description:"aoeu" uuid:"123sth"]'
on line 1 and the regular expression pattern/(\w+?):"(.*?)"/g
on line 2.After initializing the
match
variable on line 3 and creating an emptymatches
array on line 4, we begin the while loop on line 6.Within the loop, we call
regex.exec
on line 7 to search for the next match in the string. If a match is found, it is assigned to thematch
variable.On lines 8-9, we push the key-value pair into the
matches
array as an object.The loop continues until no more matches are found, at which point
regex.exec
returnsnull
and the loop breaks.Finally, we log the
matches
array to the console on line 13.
Test it Yourself! ā
Feel free to use the provided code snippet and try it out with different input strings. You'll see that now you get an array of all the key-value pairs found in the string, not just the first match. š
Conclusion and Call-to-Action š
š Congratulations! You've learned how to extract all matches from a string using RegExp.exec
and a while loop. Now, you can confidently tackle similar problems and extract the information you need from strings in your JavaScript projects! š
Remember, practice makes perfect, so give it a go with different scenarios and test cases. If you have any questions or suggestions, we'd love to hear from you in the comments below. Happy coding! šš»