regex.test V.S. string.match to know if a string matches a regular expression
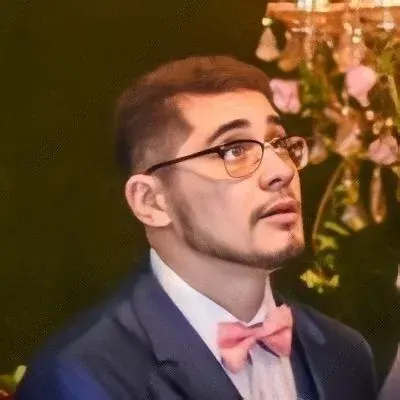
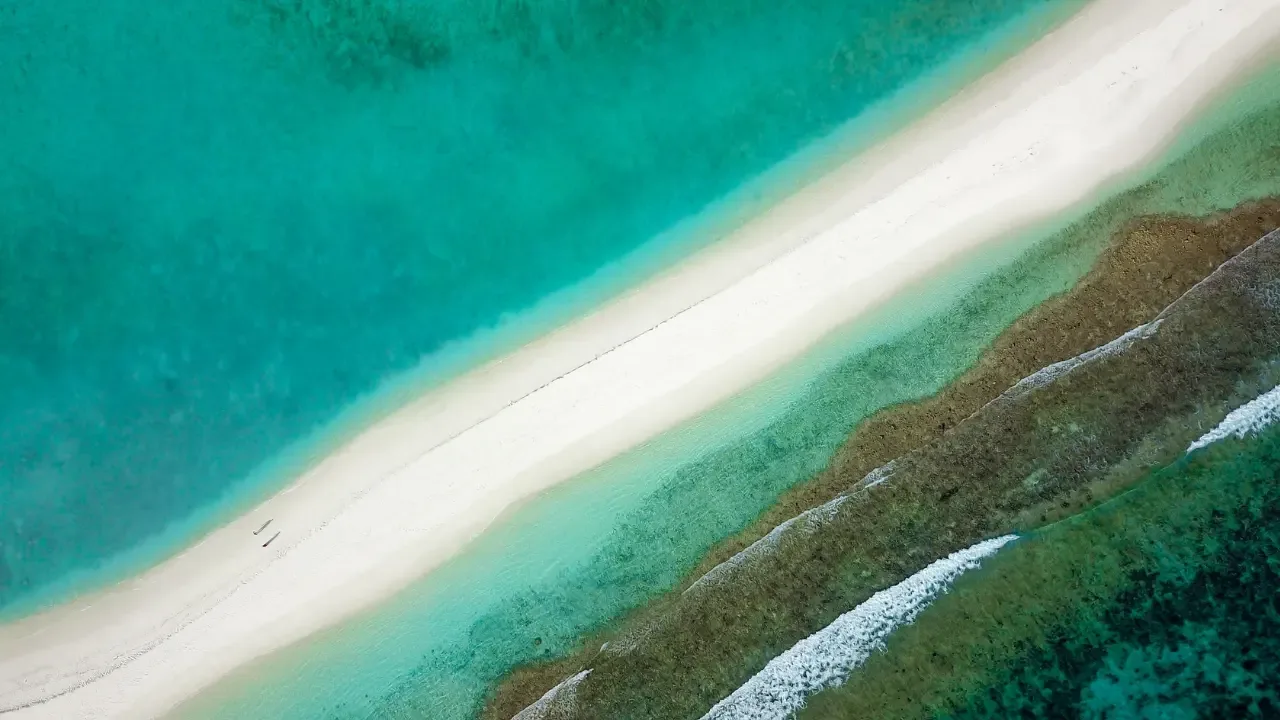
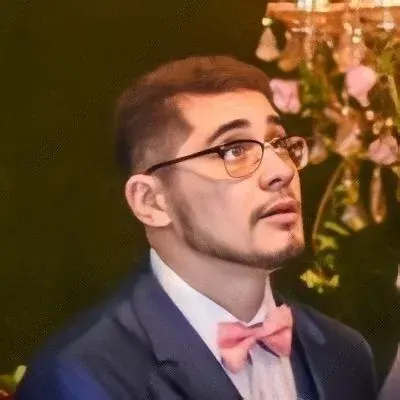
Is there a difference between regex.test
and string.match
?
š Understanding Regular Expression Matching Methods in JavaScript
Regular expressions are powerful tools used for pattern matching in strings. In JavaScript, there are two commonly used methods to determine if a string matches a regular expression: regex.test()
and string.match()
.
š¤ Common Question: What's the difference?
So, what's the difference between regex.test()
and string.match()
? At first glance, they might seem to give the same result, but there is a subtle difference in how they work.
regex.test(string)
The test()
method is a function that belongs to the RegExp
object. It returns a boolean value of true
if the string matches the regular expression and false
otherwise. Here's an example:
const str = "Hello, World!";
const regex = /World/;
if (regex.test(str)) {
console.log("The string matches the regular expression!");
} else {
console.log("The string does not match the regular expression.");
}
string.match(regex)
On the other hand, the match()
method is a function attached to a string object. It returns an array of all matched occurrences if the string matches the regular expression, and null
if there are no matches. Consider this example:
const str = "Hello, World!";
const regex = /World/;
const matchedArray = str.match(regex);
if (matchedArray) {
console.log("The string matches the regular expression!");
} else {
console.log("The string does not match the regular expression.");
}
š” Understanding the Results:
The key difference lies in the return values. While test()
returns a boolean value (true
or false
), match()
returns either an array of matched occurrences or null
.
So, if you only need to check if a string matches a regular expression, and you don't need the matched occurrences, you can use regex.test()
as it provides a simpler and more concise result.
However, if you need to extract the matched occurrences for further processing, string.match()
is a better choice, as it returns an array of matches.
š The Best Way to Use Them:
To summarize, here's when you should use each method:
Use
regex.test(string)
if you only need to check for a match and don't require the matched occurrences.Use
string.match(regex)
if you need to extract the matched occurrences for further processing.
Knowing the difference between regex.test()
and string.match()
allows you to choose the right method based on your specific requirements.
š Share Your Experience:
Have you encountered any issues while using these methods? Do you have any tips or tricks related to regular expressions in JavaScript? Share your thoughts and experiences in the comments below!
š¢ Call-to-Action: Engage with Us!
Join our community and stay up-to-date with the latest tech trends and informative guides! Subscribe to our newsletter to receive regular updates straight to your inbox. Don't miss out on the opportunity to enhance your programming skills and stay ahead of the curve. Let's connect and continue the conversation!
Now that you have a clear understanding of the difference between regex.test()
and string.match()
, you can confidently choose the best method for your needs. Use this knowledge to tackle your next regex challenge like a pro! šŖ