Referencing another schema in Mongoose
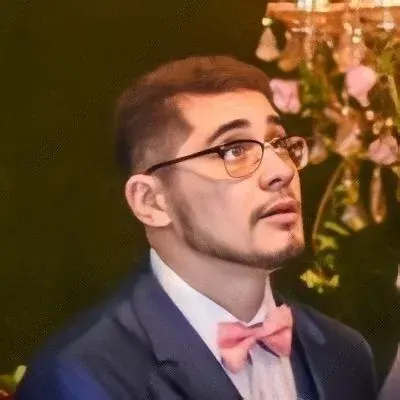
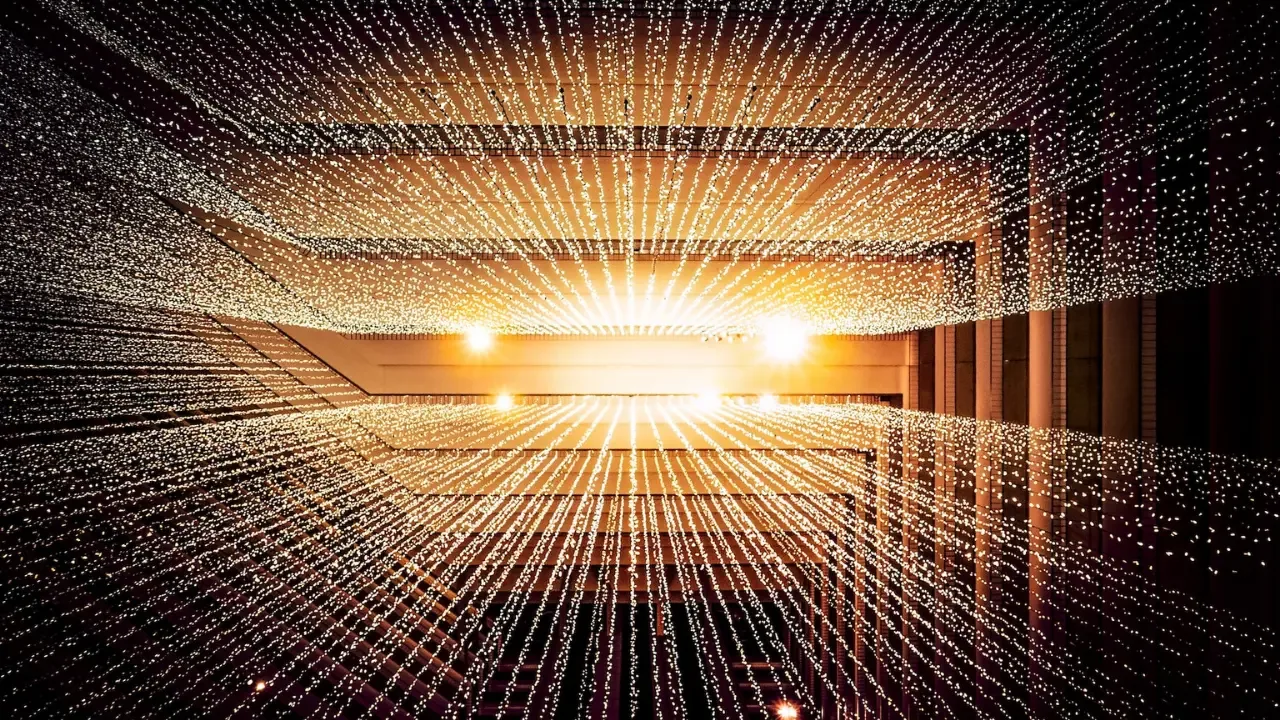
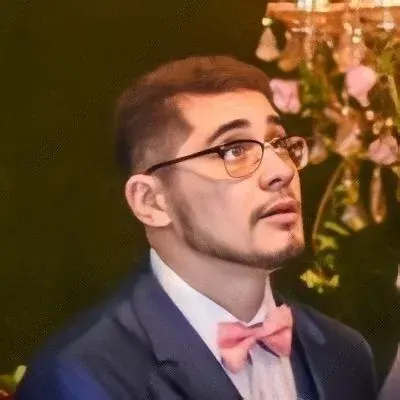
🌐 Guide to Referencing another Schema in Mongoose 🧩
Are you struggling to connect two schemas together in Mongoose? Don't worry, I've got your back! In this guide, I will walk you through the process of referencing another schema and provide you with easy solutions to address common issues. By the end, you'll be able to easily connect your schemas and make your life a whole lot easier! Let's dive right in! 💪
The Problem 😩
So, you have two schemas: userSchema
and postSchema
. You want to reference the User
model in the postedBy
field of postSchema
. Here's an example of what you're aiming for:
var userSchema = new Schema({
twittername: String,
twitterID: Number,
displayName: String,
profilePic: String,
});
var User = mongoose.model('User');
var postSchema = new Schema({
name: String,
postedBy: User, // User Model Type
dateCreated: Date,
comments: [{ body: "string", by: mongoose.Schema.Types.ObjectId }],
});
Great! But, how do you actually connect the two schemas together? 🤔
The Solution 💡
To reference another schema in Mongoose, you can make use of the ref
property. Let's modify the postSchema
to reference the User
schema:
var postSchema = new Schema({
name: String,
postedBy: { type: mongoose.Schema.Types.ObjectId, ref: 'User' },
dateCreated: Date,
comments: [{ body: "string", by: mongoose.Schema.Types.ObjectId }],
});
By setting postedBy
to { type: mongoose.Schema.Types.ObjectId, ref: 'User' }
, you are telling Mongoose that the postedBy
field should store the _id
value of a document from the 'User'
collection.
Putting It into Action 🚀
Let's say you want to retrieve the profilePic
of a post's postedBy
user. Here's how you can do it:
Post.findById(postId)
.populate('postedBy', 'profilePic')
.exec(function (err, post) {
if (err) {
// Handle error
} else {
var profilePic = post.postedBy.profilePic;
// Do something with profilePic
}
});
In the above example, we use the populate()
method to populate the postedBy
field with the actual User
document, including only the profilePic
field. This allows us to access the profilePic
directly from the postedBy
field.
Call to Action 📣
Congratulations! You've now learned how to reference another schema in Mongoose. But it doesn't stop here! Keep exploring and experimenting with Mongoose to unlock its full potential. If you found this guide helpful, share it with your fellow developers and let's spread the knowledge! 🎉
Got any questions or additional tips? Share them in the comments below and let's keep the conversation going! 👇
Happy coding! 💻🔥