ReferenceError: fetch is not defined
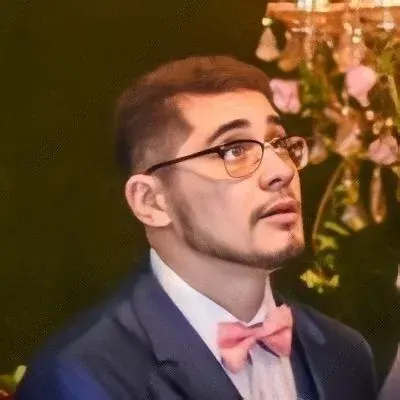
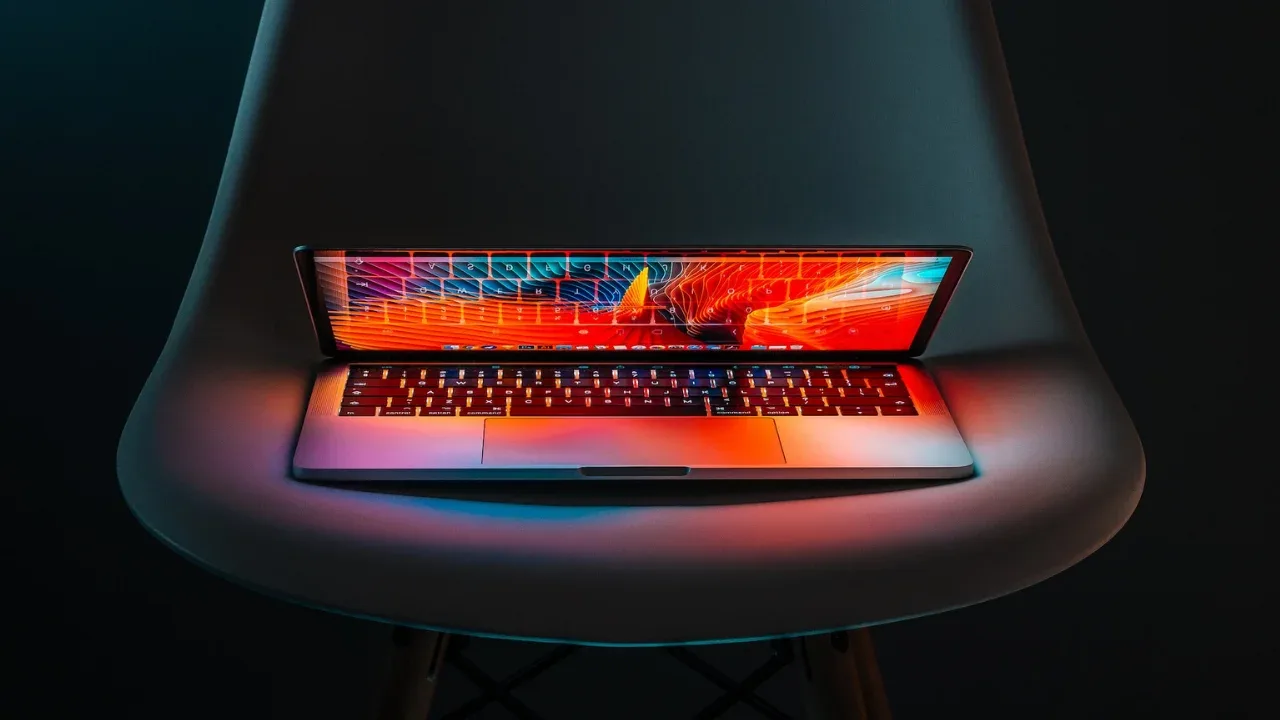
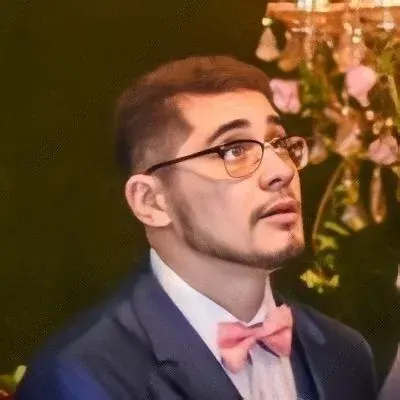
🎉📝🚀 Welcome to my tech blog! Today, we are going to tackle a common issue that many developers encounter: the dreaded ReferenceError: fetch is not defined
error in node.js. 😱
So, you've hit a roadblock while compiling your code in node.js and you're wondering how to fix it? Don't worry, I've got you covered! Let's dive in and find a solution to this problem.
The error message points out that the fetch
function is not defined. This is because the fetch
function is primarily used in the browser, and node.js doesn't have it built-in by default. But fret not, we can easily fix this by using a package called node-fetch
. 🎉
To get started, follow these steps:
Open your terminal and navigate to your project directory.
Install
node-fetch
by running the following command:npm install node-fetch
Now, in your code, require
node-fetch
at the beginning of your file:const fetch = require('node-fetch');
That's it! You've successfully fixed the
ReferenceError: fetch is not defined
issue in node.js. 🎉
Here's an updated version of your code with the necessary changes:
const fetch = require('node-fetch');
function getMovieTitles(substr){
let pageNumber=1;
let url = 'https://jsonmock.hackerrank.com/api/movies/search/?Title=' + substr + "&page=" + pageNumber;
fetch(url).then((resp) => resp.json()).then(function(data) {
let movies = data.data;
let totPages = data.total_pages;
let sortArray = [];
for(let i=0; i<movies.length;i++){
sortArray.push(data.data[i].Title);
}
for(let i=2; i<=totPages; i++){
let newPage = i;
let url1 = 'https://jsonmock.hackerrank.com/api/movies/search/?Title=' + substr + "&page=" + newPage;
fetch(url1).then(function(response) {
let contentType = response.headers.get("content-type");
if(contentType && contentType.indexOf("application/json") !== -1) {
return response.json().then(function(json) {
//console.log(json); //uncomment this console.log to see the JSON data.
for(let i=0; i<json.data.length;i++){
sortArray.push(json.data[i].Title);
}
if(i==totPages)console.log(sortArray.sort());
});
} else {
console.log("Oops, we haven't got JSON!");
}
});
}
})
.catch(function(error) {
console.log(error);
});
}
With these changes, the code will work perfectly in node.js and you won't encounter the ReferenceError: fetch is not defined
error anymore.
If you want to learn more about node-fetch
and its capabilities, check out the official npm page for this package: node-fetch - npm.
Remember, as a developer, you'll encounter many hurdles along your coding journey, but with the right knowledge and the willingness to learn, you'll overcome them all! 💪
If you found this blog post helpful, please share it with your fellow developers who might be facing the same issue. And don't forget to leave a comment below sharing your thoughts and experiences.
Happy coding! 🚀✨