Read a file one line at a time in node.js?
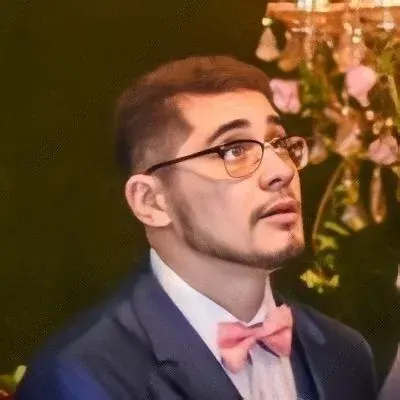
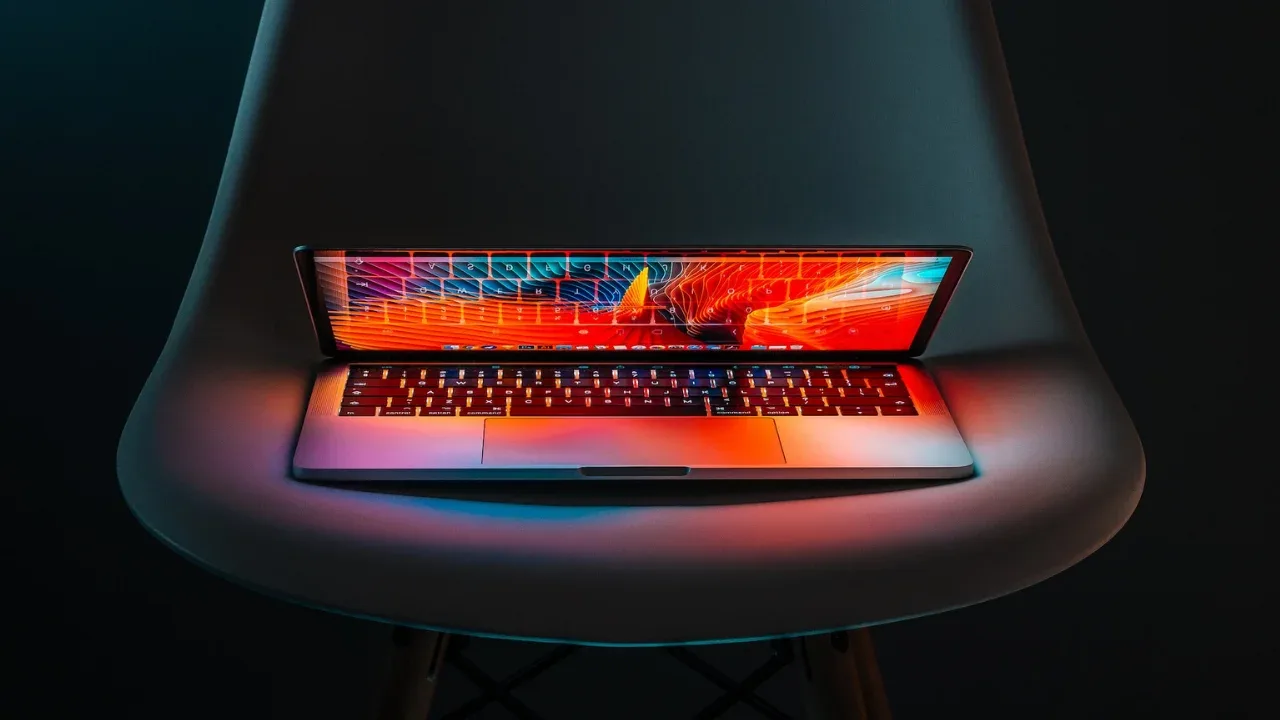
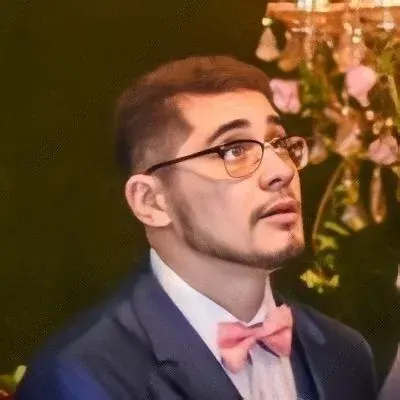
Reading a File One Line at a Time in Node.js: Easy Solutions
Have you ever encountered the need to read a large file one line at a time in Node.js? It can be a tricky task, but worry not - we're here to help! In this blog post, we'll address common issues and provide you with easy solutions to read a file line by line. So let's dive in! 💻📃
The Challenge
Recently, we stumbled upon a question on Quora that described a similar problem. The user wanted to read a file one line at a time but was having trouble making all the connections fit together. Let's take a look at the code snippet they shared:
var Lazy = require("lazy");
new Lazy(process.stdin)
.lines
.forEach(function(line) {
console.log(line.toString());
});
process.stdin.resume();
As you can see, the code reads from the standard input (STDIN) and prints each line. However, our challenge is to adapt this code to read from a file instead. Let's explore the solutions together! 🚀
Solution 1: Using the fs
Module
One straightforward solution is to use the built-in fs
module in Node.js. Here's the code snippet you tried:
fs.open('./VeryBigFile.csv', 'r', '0666', Process);
function Process(err, fd) {
if (err) throw err;
// DO lazy read
}
However, you mentioned that it's not working as expected. No worries, we've got you covered! The fs.open
method is used to open a file and obtain a file descriptor, but it doesn't provide a way to read the file one line at a time.
Solution 2: Using Line-By-Line Module
To achieve our goal, we can make use of the line-by-line
module in Node.js. This module simplifies reading a file one line at a time, even if the file size exceeds the server's memory capacity.
First, let's install the line-by-line
module via npm:
npm install line-by-line
Once installed, you can use the following code to read a file line by line:
const LineByLineReader = require('line-by-line');
const lr = new LineByLineReader('./VeryBigFile.csv');
lr.on('error', function(err) {
// Handle error
});
lr.on('line', function(line) {
console.log(line);
});
lr.on('end', function() {
// File reading is complete
});
With the line-by-line
module, you can easily read each line of your file without worrying about memory limitations.
Your Contribution
We hope these solutions have helped you overcome the challenge of reading a file one line at a time in Node.js. Now, we want to hear from you! Have you encountered any similar challenges while working with Node.js? How did you solve them? Let us know in the comments below! 👇
By sharing your experiences, you can help other developers facing similar obstacles, and together, we can create a supportive and collaborative community.
Happy coding! 💙💻
Remember to credit the original Quora post for the context of this problem, which can be found here.