ReactJS: Maximum update depth exceeded error
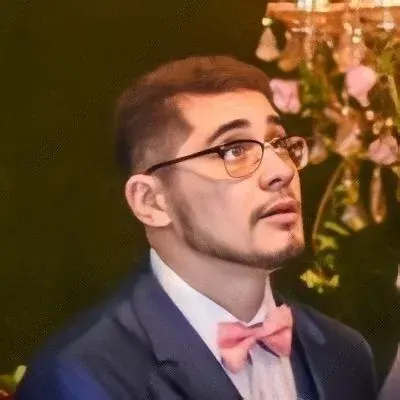
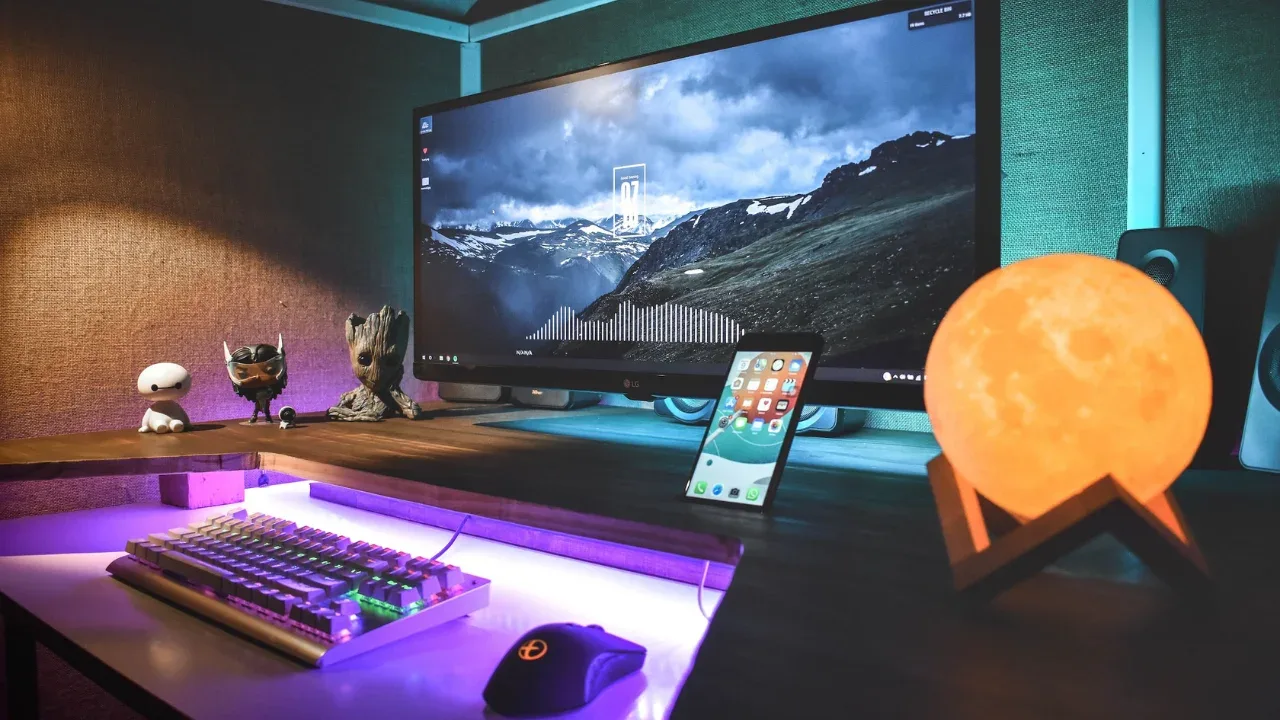
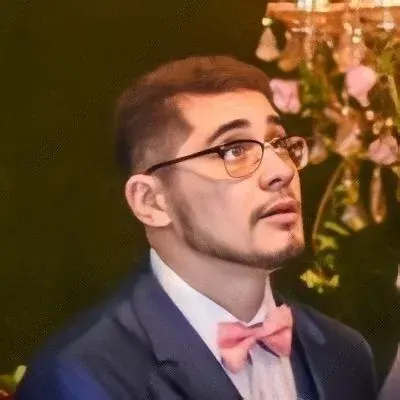
ReactJS: Maximum update depth exceeded error 😱😱
Are you seeing the dreaded "Maximum update depth exceeded" error in your ReactJS application? Don't worry, you're not alone! This error commonly occurs when a component repeatedly calls setState
inside componentWillUpdate
or componentDidUpdate
, causing React to limit the number of nested updates to prevent infinite loops. 🔄🔁
Let's take a look at your code snippet:
import React, { Component } from 'react';
import styled from 'styled-components';
class Item extends React.Component {
constructor(props) {
super(props);
this.toggle = this.toggle.bind(this);
this.state = {
details: false
}
}
toggle() {
const currentState = this.state.details;
this.setState({ details: !currentState });
}
render() {
return (
<tr className="Item">
<td>{this.props.config.server}</td>
<td>{this.props.config.verbose}</td>
<td>{this.props.config.type}</td>
<td className={this.state.details ? "visible" : "hidden"}>PLACEHOLDER MORE INFO</td>
{<td><span onClick={this.toggle()}>Details</span></td>}
</tr>
)
}
}
export default Item;
Upon initial inspection, there doesn't appear to be an obvious infinite loop in your code. However, the issue lies within the onClick
event in your render method: {<td><span onClick={this.toggle()}>Details</span></td>}
.
❌ Problem:
By invoking this.toggle()
immediately, you're repeatedly calling setState
every time the component renders. This results in the maximum update depth being exceeded error, as React detects a loop. 😫
✅ Solution:
To fix this error, you need to pass the toggle
function reference to onClick
without invoking it immediately. Simply remove the parentheses after this.toggle
.
Replace this line: {<td><span onClick={this.toggle()}>Details</span></td>}
with: {<td><span onClick={this.toggle}>Details</span></td>}
By doing this, the toggle
function will be called only when the Details
span is clicked, preventing any infinite loops and resolving the update depth error. 🎉🚀
Now, go ahead and try it out! Your component should work as expected without triggering any update depth errors.
If you found this guide helpful, don't forget to share it with your fellow ReactJS developers! Let's help each other code without frustration. 🤝📚
Got any more ReactJS questions or need help with another coding dilemma? Leave a comment below and let's dive into the world of coding together! 🌟💻💬