ReactJS - Does render get called any time "setState" is called?
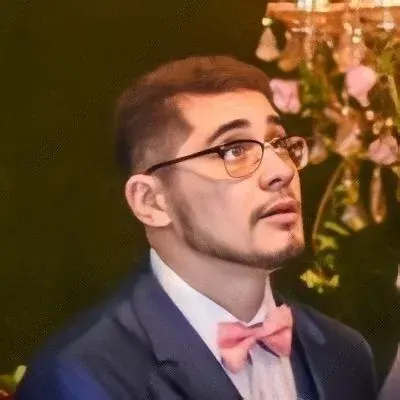
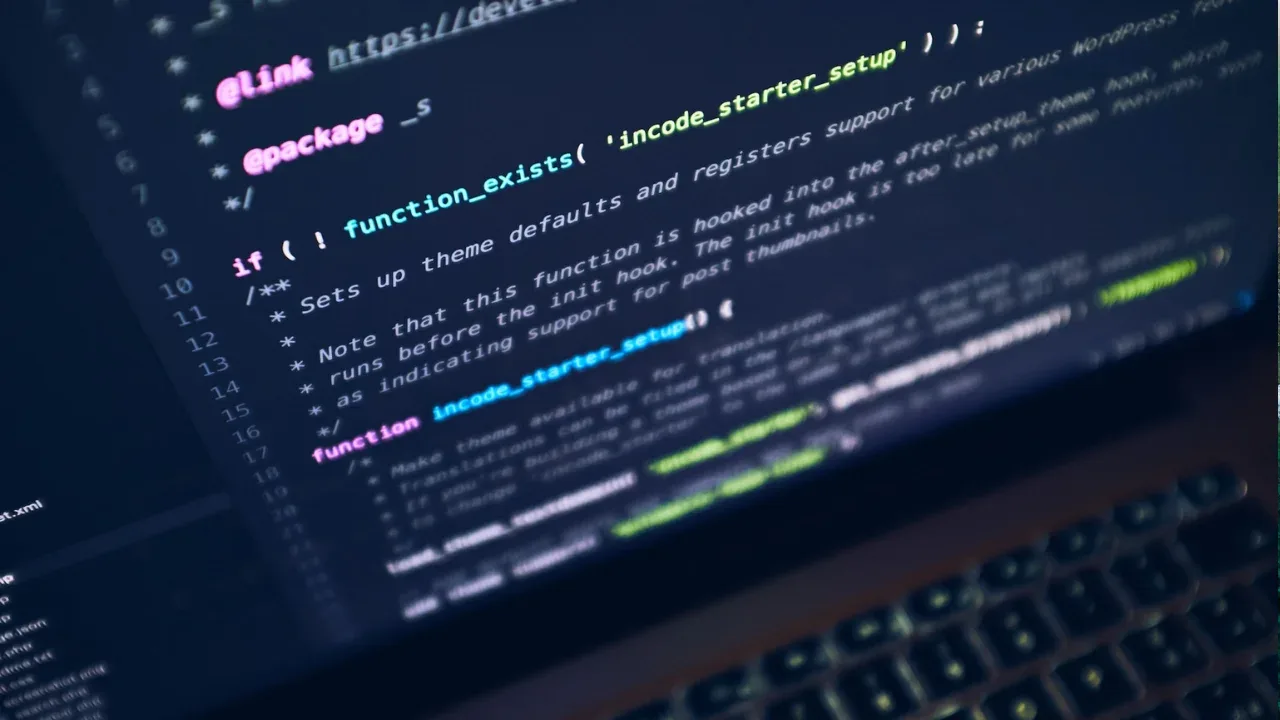
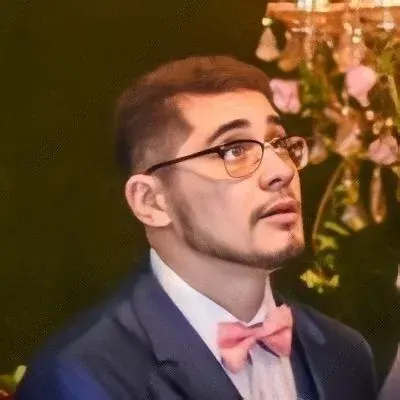
ReactJS - Does render get called any time "setState" is called? 🔄
If you're relatively new to React, you may be wondering whether the render
method gets called every time setState
is called. And if so, why? After all, isn't React all about rendering only the necessary components when the state changes?
Let's dive into this issue and shed some light on the matter. 🕵️♂️
Understanding the Problem 😕
In React, components are re-rendered whenever their state or props change. However, keep in mind that the setState
method is asynchronous and batched for performance reasons. This means that calling setState
multiple times in quick succession won't immediately trigger re-renders.
Now, in the example you provided, it may seem counterintuitive that both the Main
and TimeInChild
components are re-rendered every time the text is clicked, even though the state doesn't change. Let's take a closer look at the code snippet:
var TimeInChild = React.createClass({
render: function() {
var t = new Date().getTime();
return (
<p>Time in child: {t}</p>
);
}
});
var Main = React.createClass({
onTest: function() {
this.setState({'test':'me'});
},
render: function() {
var currentTime = new Date().getTime();
return (
<div onClick={this.onTest}>
<p>Time in main: {currentTime}</p>
<p>Click me to update time</p>
<TimeInChild/>
</div>
);
}
});
ReactDOM.render(<Main/>, document.body);
Initially, you might have expected that re-renders only happen when the state
data changes. However, since the onClick
handler always sets the state to the same value ('me'), it seems redundant for the components to re-render.
Unveiling the Solution ✨
The key to understanding this behavior lies in the React reconciliation process. When a component's state changes, React performs a virtual DOM diffing algorithm to determine which parts of the actual DOM need to be updated.
In our example, even though the state doesn't change, React still goes through the reconciliation process. However, thanks to its efficient diffing algorithm, it realizes that there are no changes to propagate to the actual DOM. Consequently, it doesn't perform any unnecessary updates, resulting in an optimized rendering process.
Therefore, while the render
method is indeed called, React cleverly avoids rendering or updating the rendered components when their state remains the same.
Engaging with the React Community 🌍
React is a vibrant and supportive community that thrives on the exchange of knowledge and ideas. If you still have questions or want to explore this topic further, why not join the conversation?
Share your React experiences in the comments section below. 🗣️
Connect with fellow React enthusiasts on social media using the hashtag #ReactJS. 🔍
Check out the official React documentation for more in-depth insights. 📖
Remember, learning is a journey, and collaborating with others can make it even more enjoyable. So let's keep exploring and evolving together! 🚀