React: why child component doesn"t update when prop changes
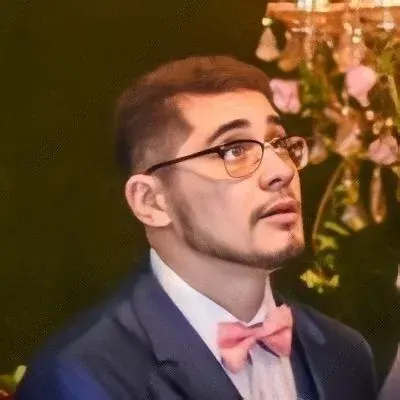
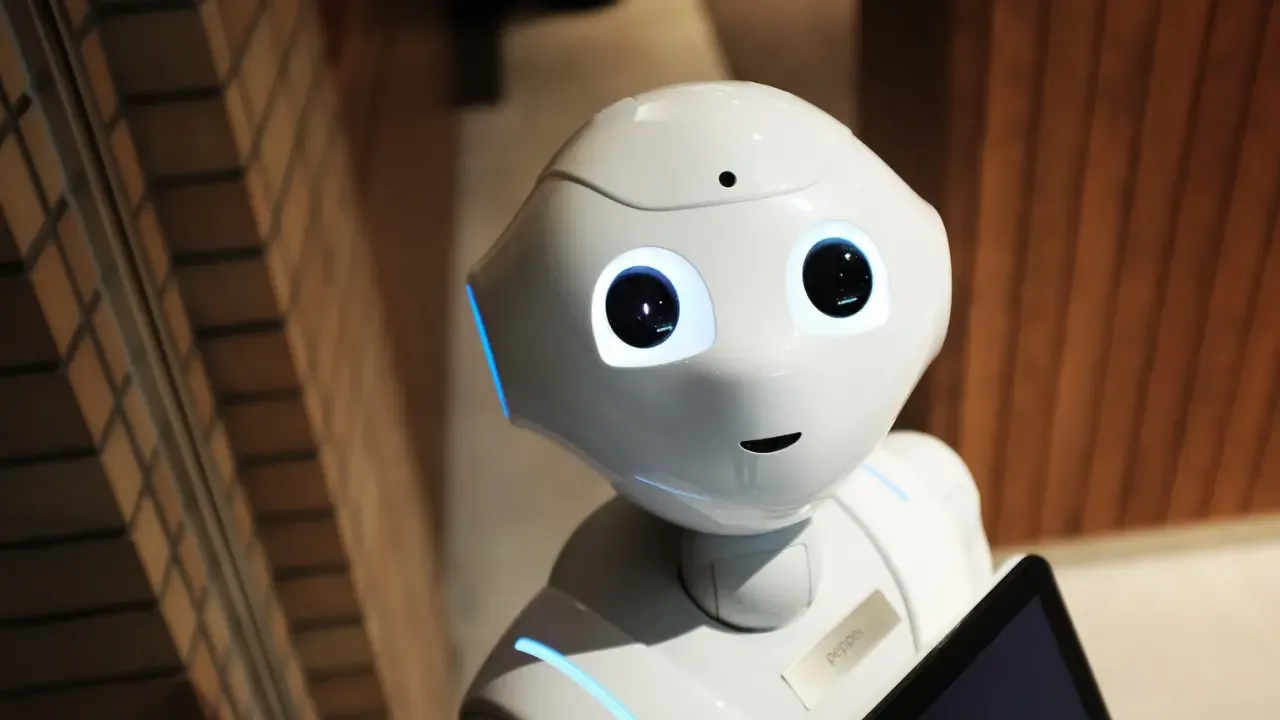
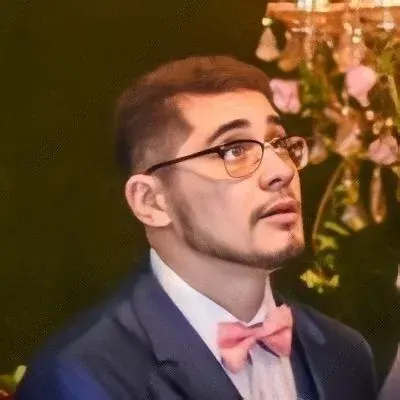
React: Why child component doesn't update when prop changes? 🤔
You're coding away in React, feeling pretty confident, when suddenly you hit a roadblock. You have a parent component, let's call it Container
, and it has a child component called Child
. 🏠👶
In the code provided, you notice that Child
is not re-rendering when the prop bar
in Container
changes. What in the world is going on here? 😮
Understanding the problem 🧐
React is all about efficient rendering and re-rendering when necessary. It uses a reconciliation algorithm to determine whether a component should be re-rendered based on changes in its props and state. But sometimes, this doesn't happen as expected. 😕
In our case, the problem lies in the way you are updating the prop bar
in the Container
component. React follows a unidirectional data flow, meaning that props should be treated as read-only and not directly modified. However, in the provided code, you are mutating the prop directly using this.props.foo.bar = 123
inside the handleEvent()
function. 🛡️💥
Because of this mutation, React doesn't detect the change in the prop and doesn't trigger a re-render of the Child
component. That's why you are seeing the old value of bar
even after calling forceUpdate()
. 😢
The solution 💡
To fix this issue, we should follow the React way of updating props by using state. Here's how we can do it:
Container {
// Use state to manage the value of foo.bar
state = {
bar: this.props.foo.bar
};
handleEvent() {
// Instead of modifying foo.bar directly, update it using setState()
this.setState({ bar: 123 });
},
render() {
return <Child bar={this.state.bar} />;
}
}
Child {
render() {
return <div>{this.props.bar}</div>;
}
}
By storing the value of bar
in the state of the Container
component and using setState()
to update it, React can properly detect the change and trigger a re-render of the Child
component. 🔄✨
The compelling call-to-action 📣
Now that you know the solution to why the child component wasn't updating, it's time to put your new knowledge into practice! Go ahead and apply these changes to your code and see the magical re-rendering happen. 🧙♂️💫
If you have any more questions or want to share your experience with React, leave a comment below! Let's dive deeper into the wonderful world of React together! 💬✨