React - uncaught TypeError: Cannot read property "setState" of undefined
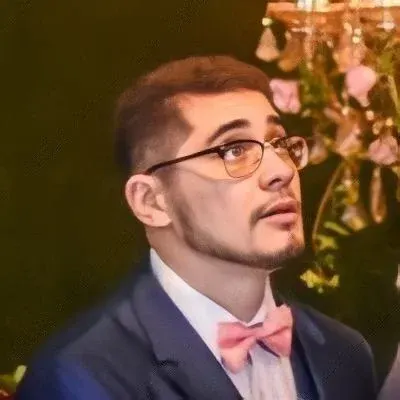
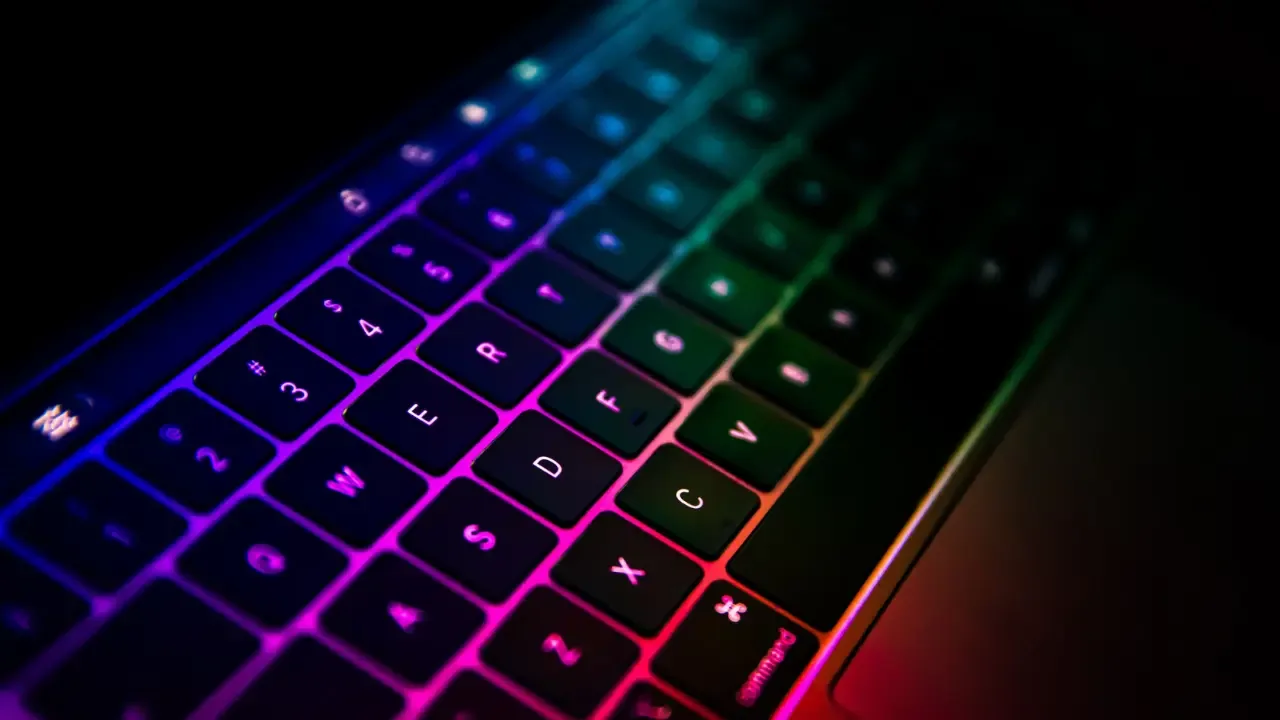
📝 React - uncaught TypeError: Cannot read property 'setState' of undefined
Are you encountering the frustrating error message "Uncaught TypeError: Cannot read property 'setState' of undefined" in your React application? Don't worry, I've got you covered! In this blog post, I will explain why this error occurs and provide easy solutions to fix it. So let's dive in and squash this bug together! 💪
Understanding the Problem 🤔
This error usually occurs when you try to access the setState
method of a component, but the component itself is undefined. In the provided code snippet, the error is happening in the delta
method. Let's break down the code to understand the issue better.
class Counter extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 1
};
this.delta.bind(this);
}
delta() {
this.setState({
count: this.state.count++
});
}
render() {
return (
<div>
<h1>{this.state.count}</h1>
<button onClick={this.delta}>+</button>
</div>
);
}
}
In the constructor, you're attempting to bind the delta
method to the component instance using this.delta.bind(this)
. However, this is not the correct way to bind a method in React. This is why you're encountering the "Cannot read property 'setState' of undefined" error.
Simple Solutions 🛠️
Fixing this issue is actually quite easy. Here are a couple of solutions that you can apply to get rid of the error:
Solution 1: Use an Arrow Function 🏹
In ES6, you can leverage arrow functions to automatically bind the this
keyword. Modify the delta
method like this:
delta = () => {
this.setState({
count: this.state.count++
});
}
By using an arrow function, you no longer have to bind the method explicitly in the constructor. The this
context will be automatically set to the component instance.
Solution 2: Bind the Method in the Constructor 👥
If you prefer not to use arrow functions, you can use the bind
method in the constructor to bind the delta
method explicitly.
constructor(props) {
super(props);
this.state = {
count: 1
};
this.delta = this.delta.bind(this);
}
Now, the this.delta = this.delta.bind(this)
line binds the delta
method to the correct this
context.
Time to Celebrate! 🥳
By applying one of these simple solutions, you should be able to fix the dreaded "Cannot read property 'setState' of undefined" error. Remember, React is all about staying flexible and embracing its powerful features, like arrow functions or explicit method binding.
If you found this blog post helpful, don't forget to share it with your fellow React developers. Have any other React issues or questions? Let's connect in the comments section below! Happy coding! 👨💻👩💻
🚀 [Call-to-Action:] Do you want to level up your React skills? Don't miss out on our upcoming React Masterclass! Sign up now and join our community of passionate developers. 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
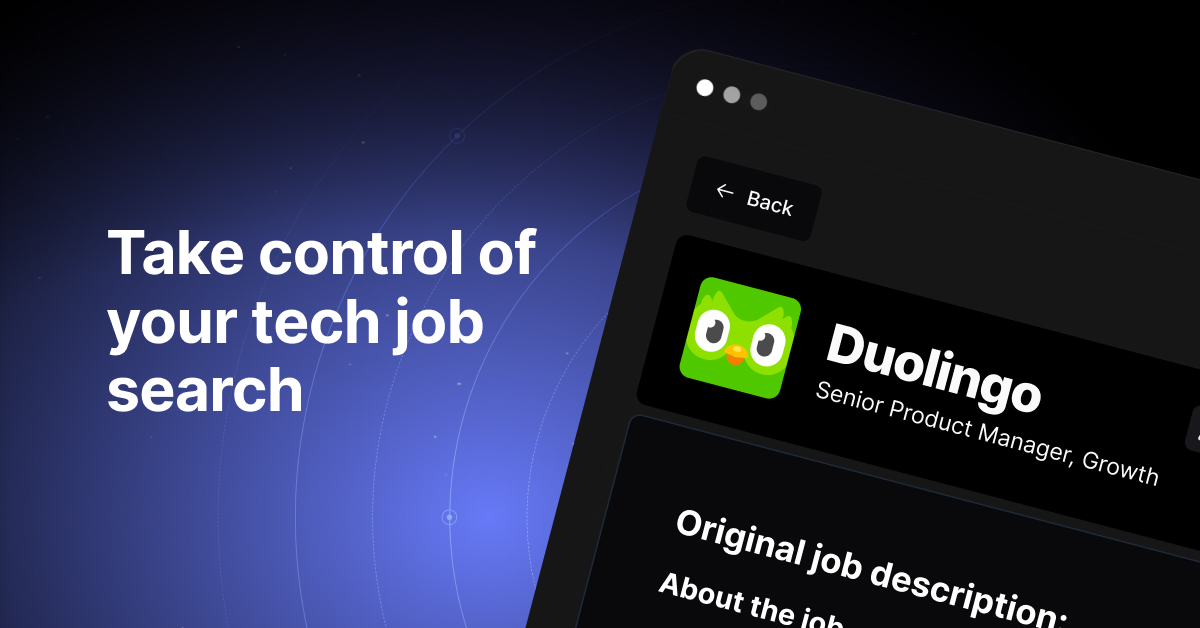