React this.setState is not a function
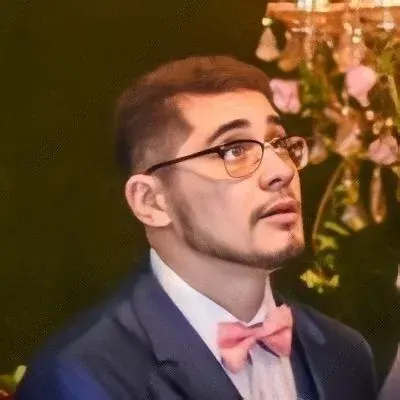
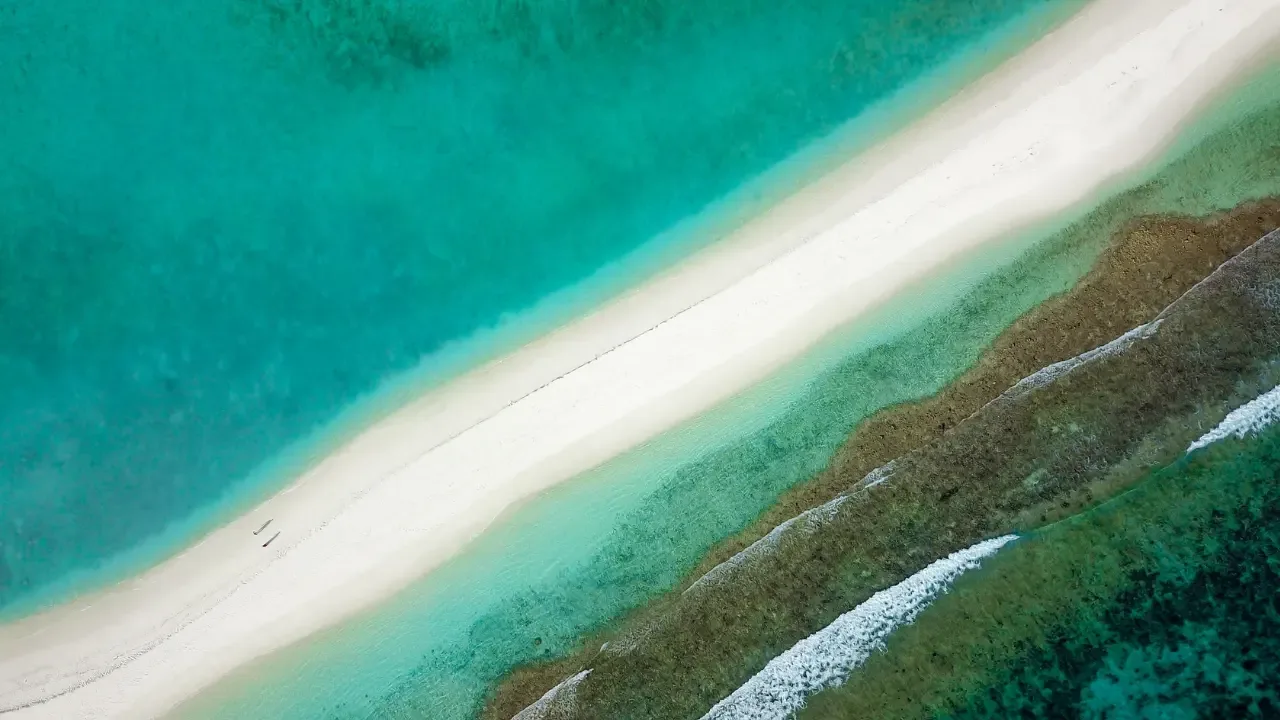
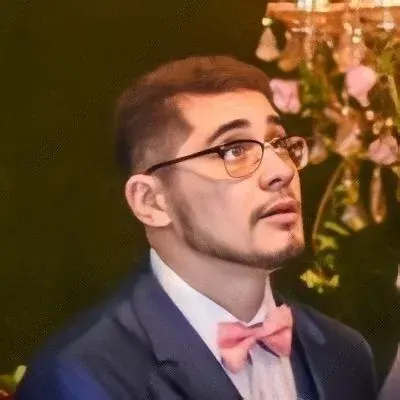
Fixing the "this.setState is not a function" error in React
If you're working with React and have encountered the error "TypeError: this.setState is not a function", you're not alone! This common issue often occurs when there is a problem with the binding of this
in your component. Luckily, there are a few easy solutions to fix this error and get your app working smoothly with a 3rd party API.
Understanding the problem
In the provided code snippet, the error arises when trying to handle the API response inside the VK.api()
callback function. The this
keyword refers to the context within the callback function rather than the React component itself. As a result, calling this.setState
throws an error because setState
is not a function within the callback's context.
Solution 1: Binding this
using arrow functions
One way to solve this issue is by using arrow functions when defining your callback functions. Arrow functions automatically bind the this
context of the parent scope, which means that you can access the component's methods and state without any issues.
VK.api('users.get',{fields: 'photo_50'}, (data) => {
if(data.response){
this.setState({
FirstName: data.response[0].first_name
});
console.info(this.state.FirstName);
}
});
By using an arrow function as the callback for VK.api()
, the this
inside the function now correctly refers to the React component, allowing you to call this.setState
without any errors.
Solution 2: Create a reference to this
If using arrow functions is not an option for you, another approach is to create a reference to this
outside of the callback function.
componentDidMount: function() {
var self = this;
VK.api('users.get',{fields: 'photo_50'}, function(data){
if(data.response){
self.setState({
FirstName: data.response[0].first_name
});
console.info(self.state.FirstName);
}
});
},
In this solution, we assign this
to a variable called self
before entering the callback function. By referencing self
, we can access the component's methods and state properly, resolving the "this.setState is not a function" error.
Solution 3: Using bind
to set the context explicitly
If you prefer a more traditional approach, you can use the bind
method to explicitly set the context of the callback function to the component.
componentDidMount: function() {
VK.api('users.get',{fields: 'photo_50'}, function(data){
if(data.response){
this.setState({
FirstName: data.response[0].first_name
});
console.info(this.state.FirstName);
}
}.bind(this));
},
By calling bind(this)
on the callback function, we ensure that this
within the callback refers to the React component. This allows us to access this.setState
without any issues.
Call-to-Action
Don't let the "this.setState is not a function" error hold you back! With these easy solutions, you can quickly fix the problem and get back to building your React app. Give these solutions a try and let us know in the comments which one worked best for you. Have you encountered any other React errors? We'd love to help you out! 👍🚀