React-router URLs don"t work when refreshing or writing manually
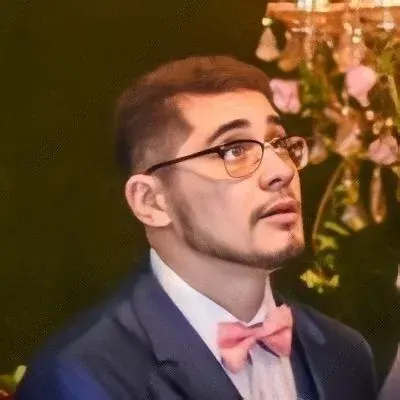
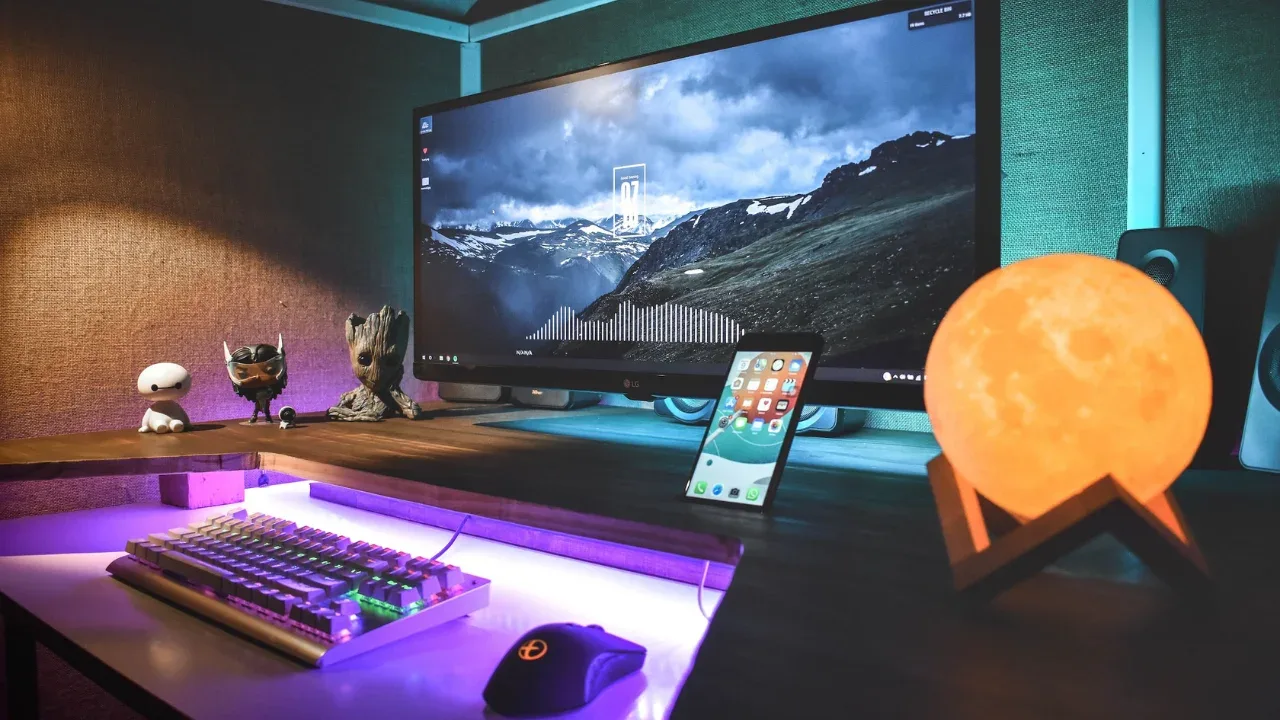
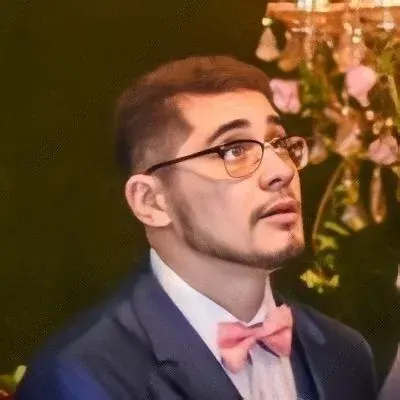
🚀📝 The React-router URL Refresh Problem: Easy Solutions for Easy Navigation
So, you're happily navigating through your React-router app, clicking on links and getting to the right pages like a breeze. But then, disaster strikes. 😱 You decide to refresh the page or manually enter a URL, and suddenly... nothing works! 😩
Don't worry, my friend. You're not alone in this struggle. Many React-router users face this issue, but fear not, for I am here to guide you through this problem and offer easy solutions that will make your URLs work like magic! ✨
🔎 Understanding the Issue
Let's start by understanding the problem you encountered. When you refresh your webpage or manually enter a URL, you may see the dreaded message: "Cannot GET /joblist". This is because React-router, by default, uses hash-based URLs (e.g., localhost/#/joblist) to handle client-side routing.
However, you don't quite like those hash URLs. Who can blame you? They just don't look as cool as simple, clean URLs. So, you tried to remove the hash from your URLs by using the Router.HistoryLocation
option. But unfortunately, this led to the issue you're facing.
🛠️ Easy Solutions
Luckily, there are a few easy solutions to get your React-router URLs working even when you refresh or manually enter them. Let's explore them one by one:
1. Use a Server-Side Solution Since React-router is a client-side routing library, it relies on the server's configuration to deliver the correct content when refreshing or manually entering URLs. Ensure that your server is set up to properly handle the routing.
For example, if you're using Express.js, you can add a catch-all route that serves your app's main HTML file for all routes. This ensures that the correct content is delivered even for routes not handled by the server.
app.get('*', function(req, res) {
res.sendFile(path.resolve(__dirname, 'path_to_your_index.html'));
});
Remember to adjust the 'path_to_your_index.html'
part to match the correct path to your app's main HTML file.
2. Configure Your Web Server If you're using a web server like Nginx or Apache, you can configure it to handle URL routing for your React-router app. This way, the server will correctly serve your app's content for any URL, including those that are refreshed or manually entered.
For Nginx, you can add a location block to handle the routing:
location / {
try_files $uri /index.html;
}
For Apache, you can use an .htaccess file with the following rule:
<IfModule mod_rewrite.c>
RewriteEngine On
RewriteBase /
RewriteRule ^index\.html$ - [L]
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule . /index.html [L]
</IfModule>
3. Switch to BrowserRouter React-router offers another router component called BrowserRouter, which uses the HTML5 History API for clean URLs. By using BrowserRouter instead of Router, you can achieve clean URLs without the need for hash routing.
Simply replace Router
with BrowserRouter
in your code, like this:
import { BrowserRouter } from 'react-router-dom';
// ...
Router.run(routes, BrowserRouter, function(Handler) {
React.render(<Handler/>, document.body);
});
Remember to also update your import statement to import from 'react-router-dom'
.
✨ The Call to Action: Engage and Share Your Success!
Now that you have easy solutions at hand, go forth and conquer those refreshing and manual URL woes! Implement the appropriate solution for your setup, and enjoy the seamless navigation experience you've always dreamed of in your React-router app.
If you found this guide helpful, why not share it with your fellow developers? Help them overcome this common React-router issue with ease. Spread the knowledge and make their lives a little bit easier too. Sharing is caring, after all! 🤝
Got any questions or want to share your success story? Leave a comment below and let's start a conversation. Happy routing! 🚀💻