react-router - pass props to handler component
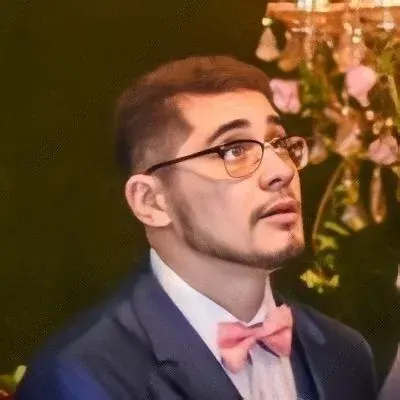
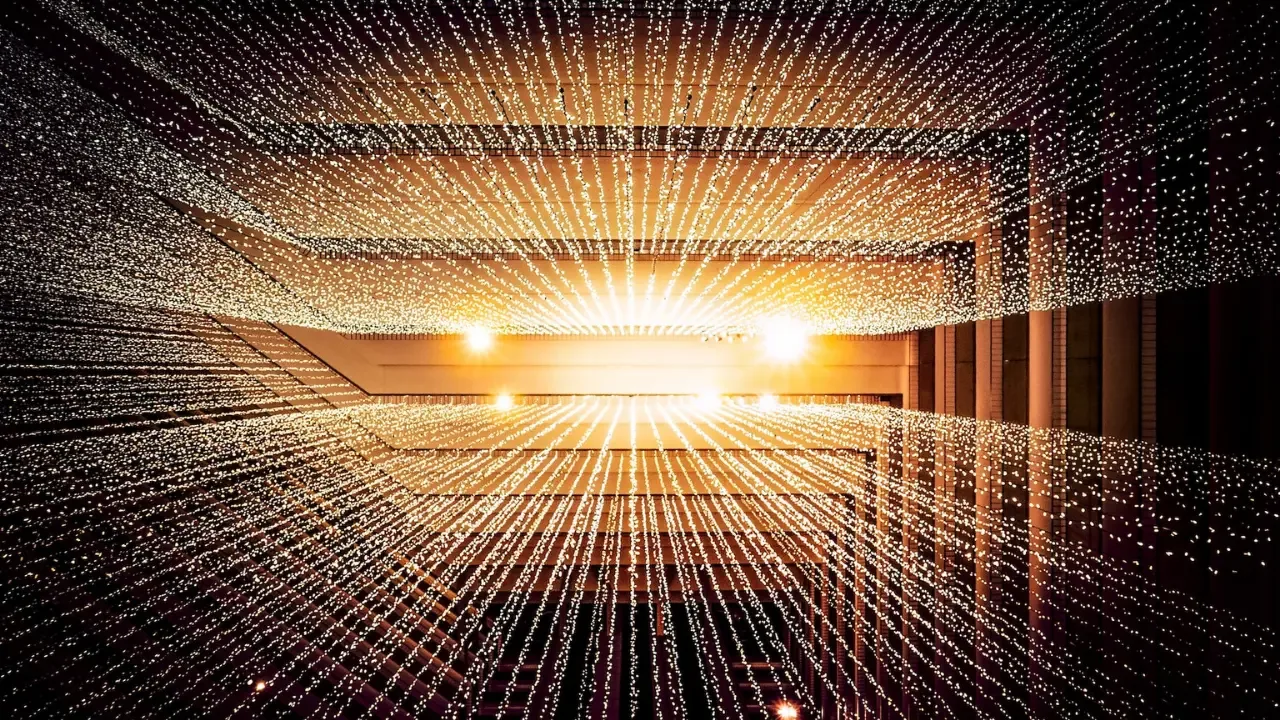
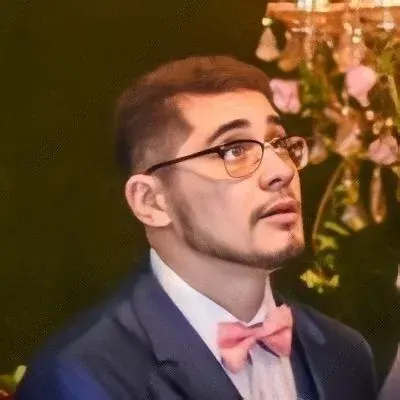
How to Pass Props to a Handler Component in React Router
Are you stuck trying to pass props to a handler component in React Router? 🤔 Don't worry, you're not alone! Many developers struggle with this common issue. In this blog post, we will explore different solutions and provide you with easy steps to pass props successfully! So, let's dive in! 💪
Understanding the Problem
To give you some context, let's take a look at the code snippet you provided:
var Dashboard = require('./Dashboard');
var Comments = require('./Comments');
var Index = React.createClass({
render: function () {
return (
<div>
<header>Some header</header>
<RouteHandler />
</div>
);
}
});
var routes = (
<Route path="/" handler={Index}>
<Route path="comments" handler={Comments}/>
<DefaultRoute handler={Dashboard}/>
</Route>
);
ReactRouter.run(routes, function (Handler) {
React.render(<Handler/>, document.body);
});
You want to pass properties (props
) to the Comments
component. However, the typical way of passing props through JSX (<Component prop1="value" />
) doesn't seem to work in this scenario. 🤔
Solution 1: Using Dynamic Segments
One solution is to use dynamic segments in your route configuration. Dynamic segments allow you to define variables in your route path and pass them as props to the corresponding handler component. Here's how you can implement this solution:
Update your
routes
configuration to include a dynamic segment:
var routes = (
<Route path="/" handler={Index}>
<Route path="comments/:myprop" handler={Comments}/>
<DefaultRoute handler={Dashboard}/>
</Route>
);
Update the
Comments
component to access the dynamic segment value:
var Comments = React.createClass({
render: function () {
var myprop = this.props.params.myprop; // Access the dynamic segment value
// Rest of the component code
}
});
Now, when you visit the /comments/value
URL, the myprop
value will be available as a prop in the Comments
component. 🎉
Solution 2: Using the createElement
Function
Another solution is to use the createElement
function provided by React Router to pass props explicitly. Here's how you can do it:
Update your
Index
component'srender
method to pass props explicitly:
var Index = React.createClass({
render: function () {
var propsToPass = {
myprop: "value" // Add your desired props here
};
return (
<div>
<header>Some header</header>
{React.createElement(RouteHandler, propsToPass)}
</div>
);
}
});
Update your
Comments
component to access the passed props:
var Comments = React.createClass({
render: function () {
var myprop = this.props.myprop; // Access the passed prop
// Rest of the component code
}
});
By using the createElement
function, you can explicitly pass props to the RouteHandler
and have them accessible in the corresponding handler component. 🙌
Conclusion and Call-to-Action
Passing props to a handler component in React Router can be a bit tricky, but with the solutions provided above, you can do it easily! Remember, you can either use dynamic segments or the createElement
function to achieve your goal.
Now it's your turn to apply these solutions to your React application and pass props like a pro! Share your experience and any other tips or tricks you discover along the way. We would love to hear from you! 😊
If you found this article helpful, make sure to share it with your fellow developers who might be struggling with the same issue. Sharing is caring! ❤️