React JSX: selecting "selected" on selected <select> option
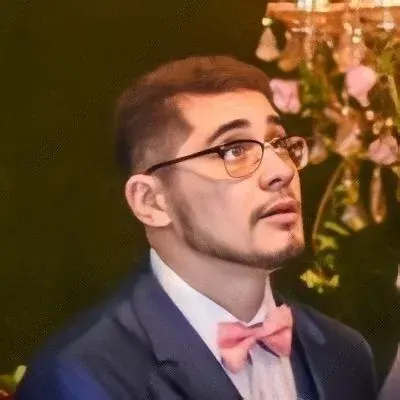
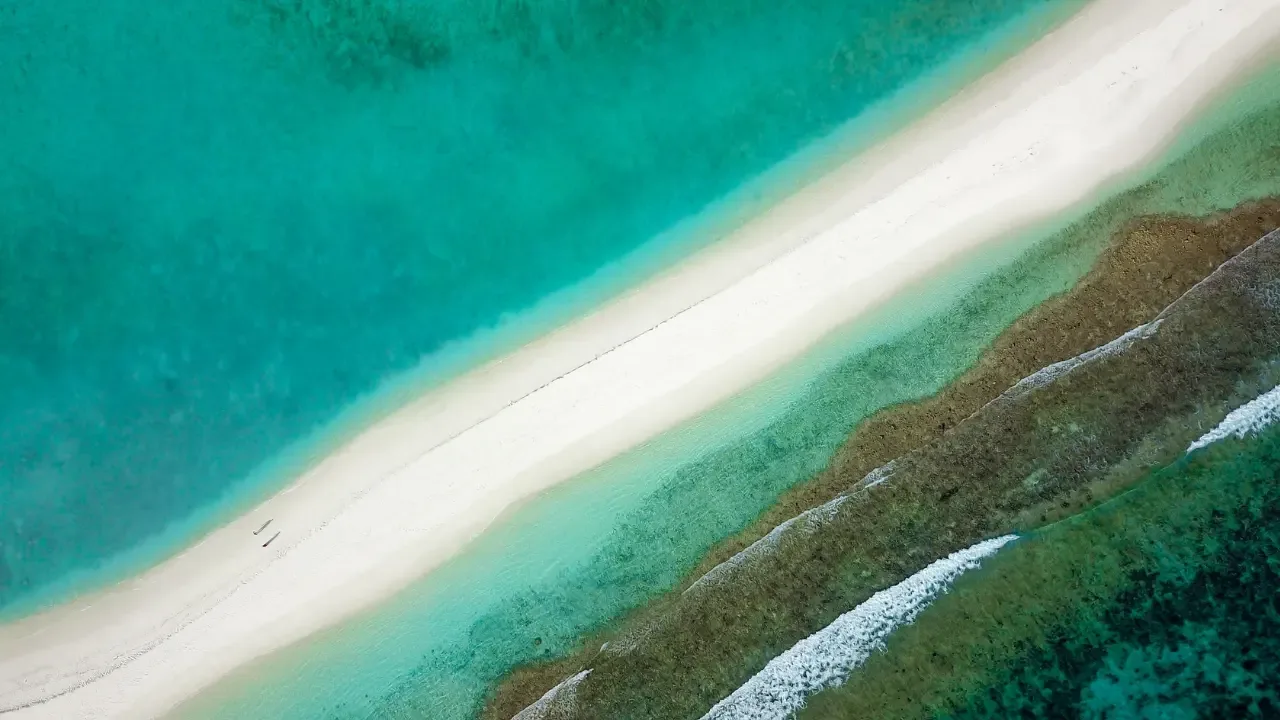
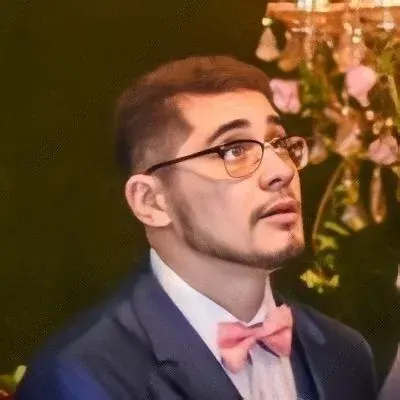
👋 Welcome to my tech blog! Today, we're going to tackle a common issue when working with React JSX: how to set the "selected" attribute on a selected <select>
option. 😊
In this scenario, we have a React component for a <select>
menu, and we need to dynamically set the "selected" attribute on the option that reflects the application state. Let's dive right into the code and explore some possible solutions! 💻
🔍 Understanding the problem
The code you provided attempts to check whether optionState
matches the value of each option and conditionally set the "selected" attribute. However, it triggers a syntax error on JSX compilation. 😮
🔄 Solution 1: Using a ternary operator You can solve this problem by using a ternary operator to conditionally set the "selected" attribute. Let's take a look at an example:
render: function() {
var options = [];
var optionState = this.props.optionState;
this.props.options.forEach(function(option) {
var selected = (optionState === option.value) ? 'selected' : '';
options.push(
<option value={option.value} selected={selected}>{option.label}</option>
);
});
return (
<select>{options}</select>
);
}
In this solution, we check if optionState
matches the value of each option. If there's a match, we set the selected
variable to 'selected'
, indicating that this option should be selected. Otherwise, selected
remains an empty string.
💡 Note: Make sure to include the return
statement to render the <select>
element containing the options.
💥 Solution 2: Using a boolean value Alternatively, you can directly assign a boolean value to the "selected" attribute. Here's an example:
render: function() {
var options = [];
var optionState = this.props.optionState;
this.props.options.forEach(function(option) {
var selected = (optionState === option.value);
options.push(
<option value={option.value} selected={selected}>{option.label}</option>
);
});
return (
<select>{options}</select>
);
}
In this approach, selected
is a boolean value (true
or false
). If optionState
matches the option's value, selected
will be true
, and the "selected" attribute will be present in the rendered HTML.
🎉 Problem solved! With either of these solutions, you should no longer encounter a syntax error, and the correct option will be marked as selected based on the application state. 🙌
👉 However, when using React, it's generally recommended to manage form-related state using the value
prop on the <select>
element, rather than relying on the "selected" attribute. This ensures that React has full control over the form value and makes it easier to handle user interactions. But for simple cases, the solutions provided above should work fine!
🌟 Don't stop here! Have you encountered any other challenges when working with React or JSX? Let me know in the comments below! Let's keep learning and solving problems together. 💪
That's all for today's blog post! Thanks for joining me. See you next time! Happy coding! 😄👩💻👨💻