React / JSX Dynamic Component Name
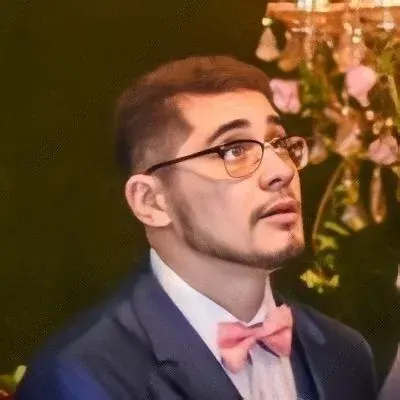
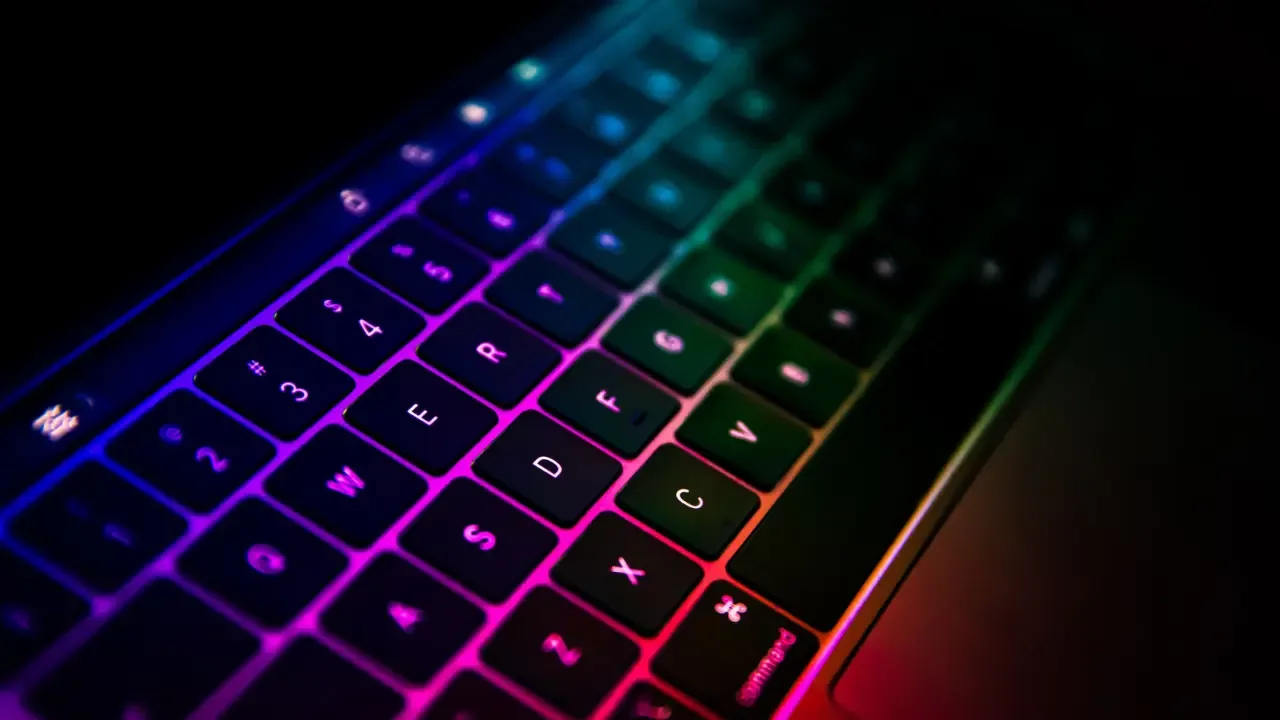
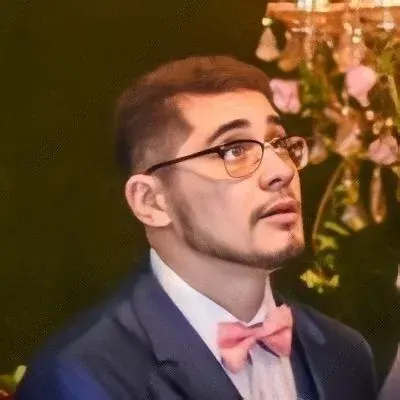
šTitle: How to Dynamically Render React Components with JSX
āØIntroduction Have you ever tried dynamically rendering React components based on their type, only to be frustrated by unexpected errors and workarounds? Fear not! In this guide, we will explore common issues and provide you with easy solutions to dynamically render React components using JSX. Let's dive in and discover a more elegant solution to this problem!
š”Understanding the Problem
The original question posed an issue where dynamically rendering a component based on its type resulted in unexpected output. For example, instead of rendering <ExampleComponent />
, it rendered <examplecomponent />
. This led to confusion and the need for a more efficient solution.
šThe Initial Approach The first attempt involved concatenating a string to dynamically generate a component's name. However, this approach failed and caused an error during compilation. It turned out that JSX expected XML syntax instead of an array-like syntax.
ā The Solution with Methods One way to overcome this roadblock is to create a method for each component. This method approach allows us to programmatically generate component names without encountering issues with JSX.
newExampleComponent() {
return <ExampleComponent />;
}
newComponent(type) {
return this["new" + type + "Component"]();
}
By using this method approach, you can dynamically render components without compromising the elegance of your code. However, the downside is that you would need to create a new method for every component you develop. This can become tedious and repetitive.
šA More Elegant Solution Luckily, there is a more elegant solution to dynamically rendering React components using JSX. React has an official documentation entry that addresses this issue: Choosing the Type at Runtime.
This official documentation provides various ways to render components dynamically, including using variables as JSX tags or using a mapping object. Let's explore a couple of these options!
šOption 1: Using Variables as JSX Tags Instead of creating separate methods, you can use a variable as the JSX tag. Let's modify the initial code snippet using this technique:
const type = "Example";
const ComponentName = type + "Component";
return <ComponentName />; // Returns <ExampleComponent />
This approach allows you to dynamically render components without the need for explicit method creation. It reduces redundancy and simplifies your codebase.
šOption 2: Using a Mapping Object Another elegant solution to dynamically render components is by using a mapping object. This approach is especially useful when you have a large number of components or types. Here's an example:
const componentMap = {
Example: ExampleComponent,
Another: AnotherComponent,
// Add more components and types here
};
const type = "Example";
const ComponentName = componentMap[type];
return <ComponentName />; // Returns <ExampleComponent />
By creating a mapping object, you can easily associate the component name with its actual component. This approach provides flexibility and scalability.
š”Conclusion When faced with the challenge of dynamically rendering React components using JSX, it's important to consider more elegant and efficient solutions. From using variables as JSX tags to creating a mapping object, you have several options to choose from. Remember to consult React's official documentation for further guidance and best practices.
āØCall-to-Action Ready to level up your React development skills? Explore more tips and tricks by visiting our blog and discovering other insightful guides. Join our community of passionate developers and stay ahead of the curve! Let's empower each other in the world of React. šš»
šAdditional Resources