React js onClick can"t pass value to method
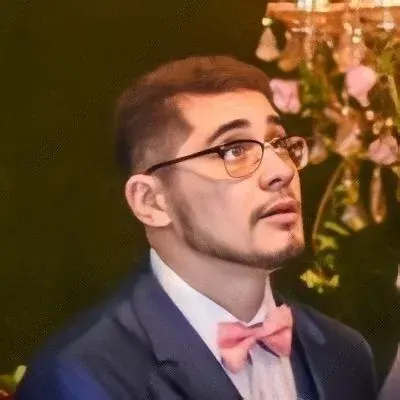
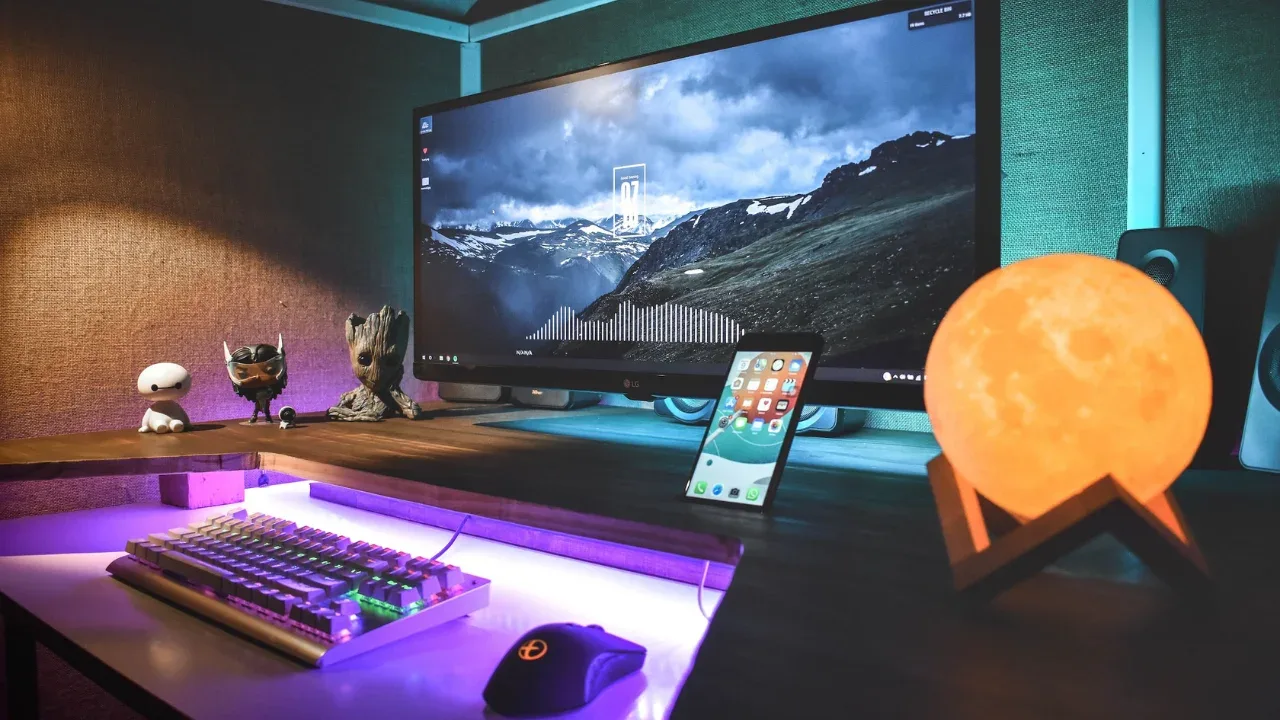
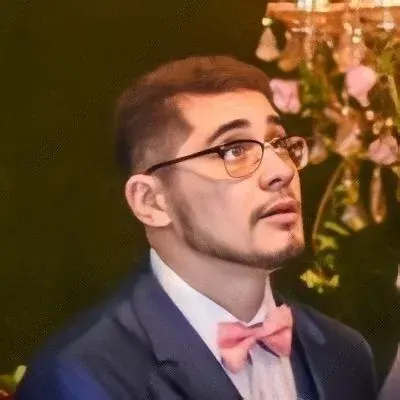
React js onClick: How to Pass Value to Method
Have you ever been puzzled by the fact that you can't pass a value to the onClick
event in React? 🤔 Don't worry, you're not alone! Many developers have encountered this common issue, but fear not, because we have easy solutions for you! 😄
Understanding the Problem
Let's first understand why passing a value to the onClick
event in React is not straightforward. In React, the onClick
event expects a function reference as its value. However, if you try to pass a value directly, it won't work as expected. Instead, React will pass an event object as the argument to the function. This event object contains information about the event that occurred, but not the value you intend to pass.
The Solution
To solve this problem, we need to find a way to pass the desired value along with the event object. In React, there are a couple of approaches you can take:
Approach 1: Using an Anonymous Function
One simple solution is to use an anonymous function as the event handler. This allows you to pass the desired value as an argument to the function. Here's how you can modify your code to achieve this:
<th onClick={() => that.handleSort(column)}>{column}</th>
In this example, we created an anonymous function that calls that.handleSort(column)
. The column
variable will contain the desired value passed from the map
function.
Approach 2: Using bind
Another approach is to use the bind
method to create a new function with the desired value bound to it. Here's how you can modify your code to achieve this:
<th onClick={that.handleSort.bind(null, column)}>{column}</th>
In this example, we use the bind
method to create a new function reference where null
is the value of this
and column
is the desired value passed as an argument to that.handleSort
.
Putting it All Together
Now, let's see how our modified code looks like:
var HeaderRows = React.createClass({
handleSort: function(value) {
console.log(value);
},
render: function () {
var that = this;
return(
<tr>
{this.props.defaultColumns.map(function (column) {
return (
<th onClick={() => that.handleSort(column)}>{column}</th>
);
})}
{this.props.externalColumns.map(function (column) {
var externalColumnName = column[0];
return (<th>{externalColumnName}</th>);
})}
</tr>
);
}
});
With these modifications, you can now pass a specific value to the handleSort
method when the onClick
event is triggered.
Your Turn to Explore
Now that you have learned how to pass a value to the onClick
event in React, why not try implementing it in your own project? 💻 Experiment with different values and see how it works for you. And remember, if you ever need help or have questions, don't hesitate to reach out to the React community.
Join the conversation and let us know your thoughts or any other issues you've encountered with React's onClick
event. We would love to hear from you! Share your experiences and insights in the comments below. Happy coding! 😊✨