React Hooks useState() with Object
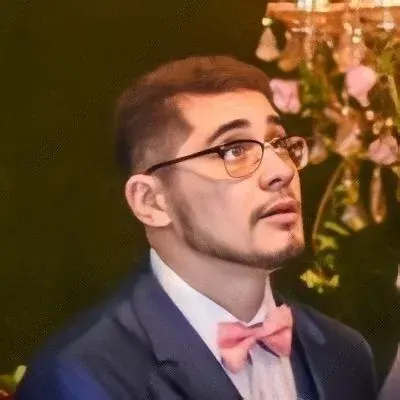
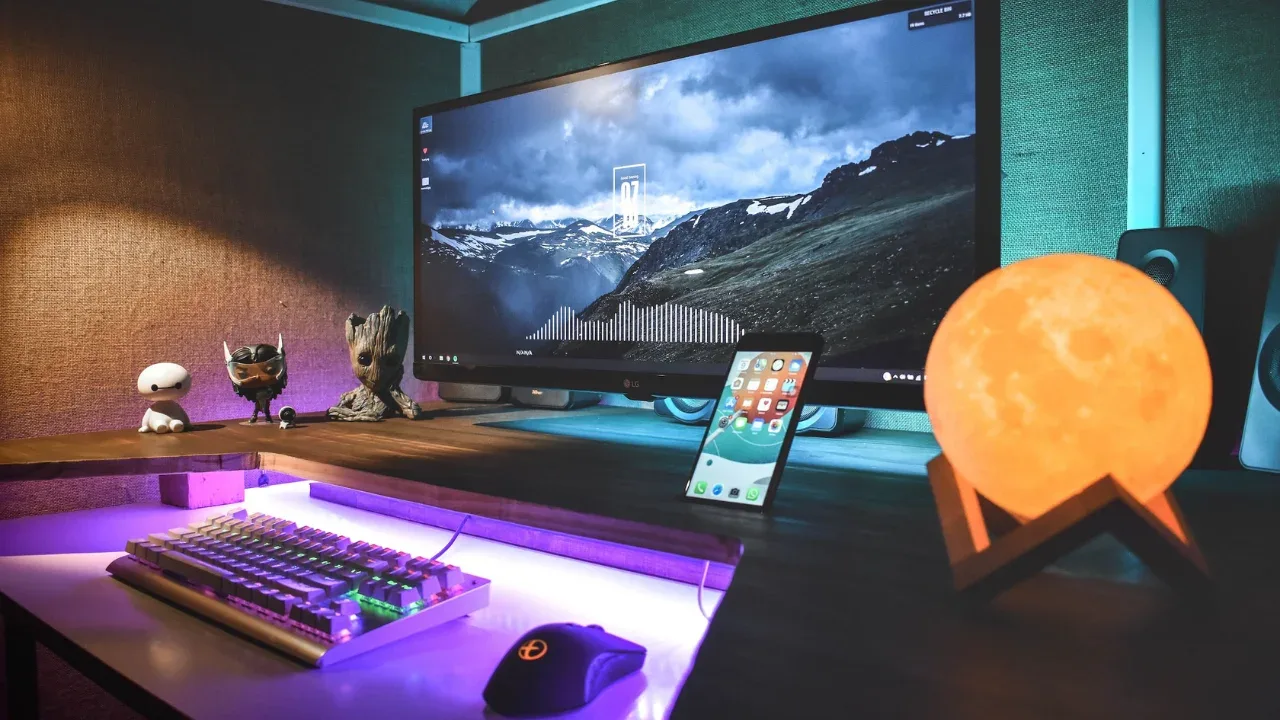
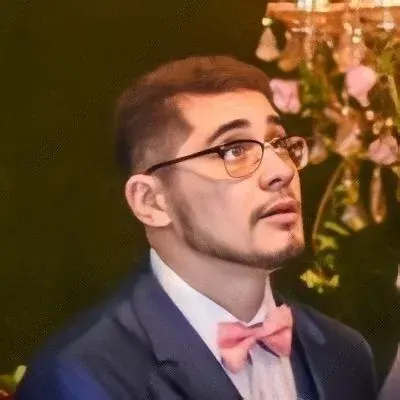
Updating Nested State in React Hooks: A Complete Guide
So, you're working with React Hooks and want to update a nested object state using the useState()
hook. 🤔 You've come to the right place! In this blog post, we'll tackle the common issues faced when modifying nested state objects in React and provide easy solutions to overcome them. Let's dive in! 💪
The Scenario
Imagine you have the following initial state defined using the useState()
hook:
const [exampleState, setExampleState] = useState({
masterField: {
fieldOne: "a",
fieldTwo: {
fieldTwoOne: "b",
fieldTwoTwo: "c"
}
}
});
And you're trying to achieve two things:
Append a new field within the
masterField
object, resulting in:
const a = {
masterField: {
fieldOne: "a",
fieldTwo: {
fieldTwoOne: "b",
fieldTwoTwo: "c"
}
},
masterField2: {
fieldOne: "c",
fieldTwo: {
fieldTwoOne: "d",
fieldTwoTwo: "e"
}
}
}
Change the values of the
masterField
object to:
const b = {
masterField: {
fieldOne: "e",
fieldTwo: {
fieldTwoOne: "f",
fieldTwoTwo: "g"
}
}
}
🛠️ Solution 1: Appending a Field
To append a field within a nested object, you need to spread the nested object and then add the new field. Here's how you can achieve it:
const updatedState = {
...exampleState,
masterField2: {
fieldOne: "c",
fieldTwo: {
fieldTwoOne: "d",
fieldTwoTwo: "e"
}
}
};
setExampleState(updatedState);
In this case, we spread the existing exampleState
and added a new property, masterField2
, with its respective nested fields. Finally, we pass the updated state to setExampleState()
to trigger a re-render with the new state.
🛠️ Solution 2: Changing Values
To change the values of the masterField
object, you can adopt a similar approach. Here's how you can accomplish it:
const updatedState = {
...exampleState,
masterField: {
fieldOne: "e",
fieldTwo: {
fieldTwoOne: "f",
fieldTwoTwo: "g"
}
}
};
setExampleState(updatedState);
Similar to Solution 1, we spread the existing exampleState
and replaced the masterField
object with a new one containing the desired values. Finally, we call setExampleState()
to trigger a re-render with the modified state.
Wrapping Up
Updating nested state in React using the useState()
hook might've seemed challenging at first, but with the solutions provided above, you now have the tools to tackle this problem with ease. 🚀
Feel free to experiment with these solutions in your own codebase and let us know how it goes! If you have any other questions or face any difficulties related to React Hooks or state management, leave a comment below, and we'll be glad to assist you. Happy coding! 💻
Remember to share this post with your fellow developers if you found it helpful! 👍
Call-to-Action
📢 Did you find this blog post useful? Have you encountered any other tricky issues while working with React Hooks? Share your experience in the comments below and let's learn together! 🌟