React Hook Warnings for async function in useEffect: useEffect function must return a cleanup function or nothing
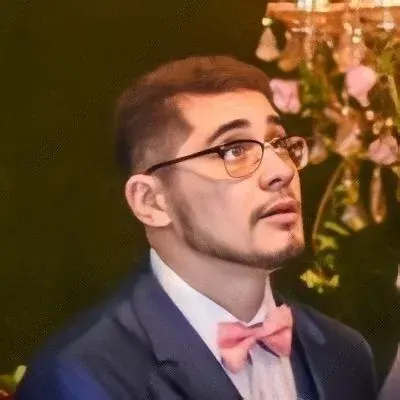
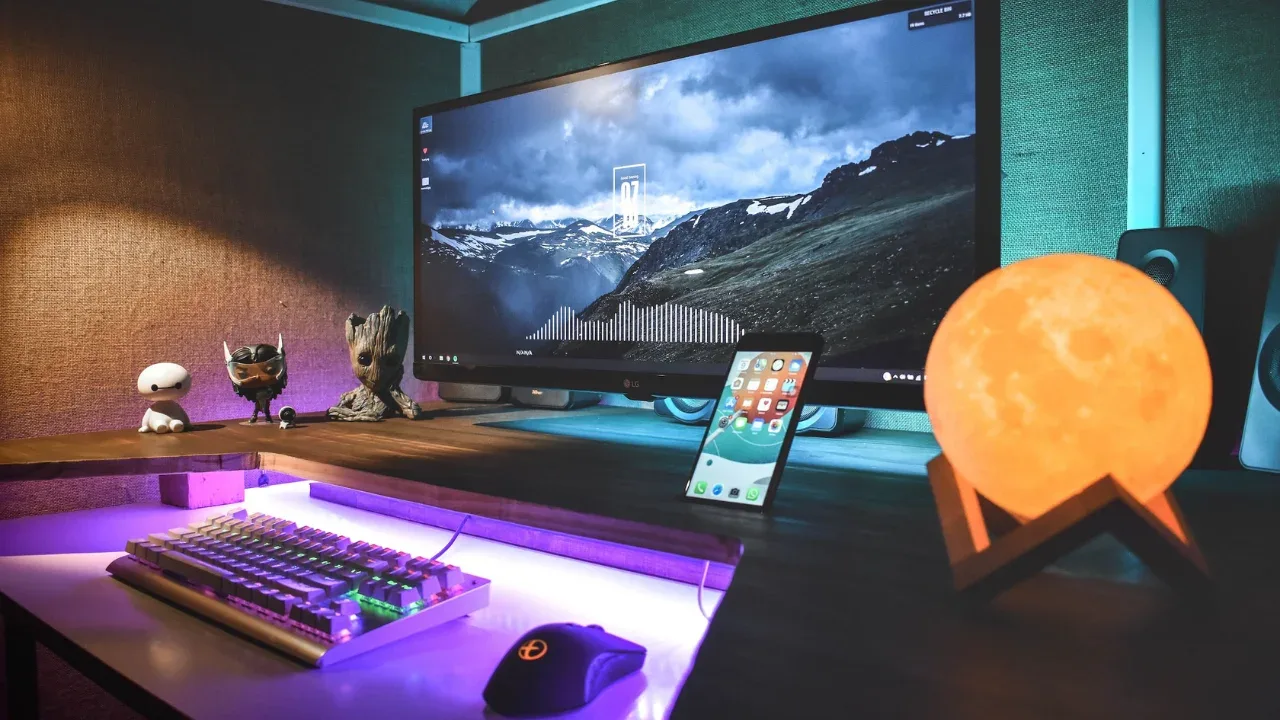
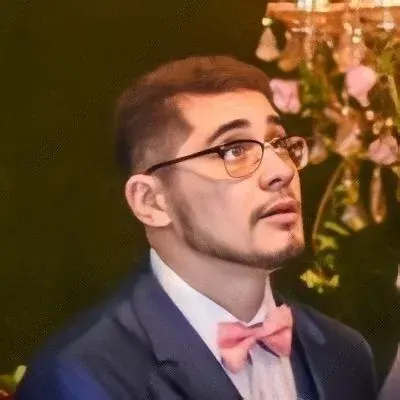
π Title: Getting Rid of React Hook Warnings for Async Functions in useEffect
π Hey there, tech enthusiasts! Are you facing React Hook warnings when using async functions in useEffect? π€ Don't worry, because I'm here to help you understand and solve this common issue effectively. Let's dive right in! π»π
π€ The Issue: Why am I getting a React Hook warning for async functions in useEffect?
The warning message you see in your console, "useEffect function must return a cleanup function or nothing," might seem confusing at first. However, you're correct in thinking that the cleanup function is optional for async calls. So, why is React throwing this warning at you? π€·ββοΈ
π§ The Solution: Understanding the root of the warning
To understand the warning, let's take a close look at the useEffect function you're using:
useEffect(async () => {
try {
// Your async code...
} catch (e) {
console.error(e);
}
}, []);
The issue lies in the fact that the useEffect hook expects a "cleanup" function to handle any necessary cleanup actions when unmounting the component or before the next useEffect run. However, when using an async function as the effect, React assumes that the returned value (i.e., the promise) is the cleanup function. This creates a mismatch between React's expectation and the async function behavior, leading to the warning message you see.
π The Solution: Handling the warning and resolving the issue
To solve this problem, we have a couple of options:
1. Option 1: Removing the async keyword You can remove the async keyword from the useEffect callback function and use a traditional promise chain instead. Here's how your code would look:
useEffect(() => {
fetch(`https://www.reddit.com/r/${subreddit}.json`)
.then(response => response.json())
.then(json => setPosts(json.data.children.map(it => it.data)))
.catch(error => console.error(error));
// No cleanup function needed; async effect resolved with promise chain
}, []);
π‘Although removing the async keyword works fine in most cases, keep in mind that it changes the behavior of your code. If you prefer to keep the async/await approach, we have an alternative solution for you. π
2. Option 2: Using a separate async function In this approach, we'll define a separate async function inside useEffect and immediately invoke it. Here's your updated code:
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(`https://www.reddit.com/r/${subreddit}.json`);
const json = await response.json();
setPosts(json.data.children.map(it => it.data));
} catch (error) {
console.error(error);
}
};
fetchData();
// No cleanup function needed; the separate async function handles everything
}, []);
π Great job! By extracting the async code to a separate function and invoking it immediately, we bypass the issue of React expecting the async function to return a cleanup function.
π£ Call to Action: Share your experience and engage with us!
I hope this guide helped you understand and resolve the React Hook warnings for async functions in useEffect. Now it's your turn! Share your thoughts, experiences, or any other React issues you've encountered in the comments below. Let's build a vibrant tech community together! ππ
π Remember, understanding the root cause and exploring different solutions is essential to mastering any development framework. Keep experimenting, sharing knowledge, and happy coding! πͺπ»
Sources:
Stack Overflow: React Warning for Empty Promises
Codesandbox: Async Function in useEffect