React - changing an uncontrolled input
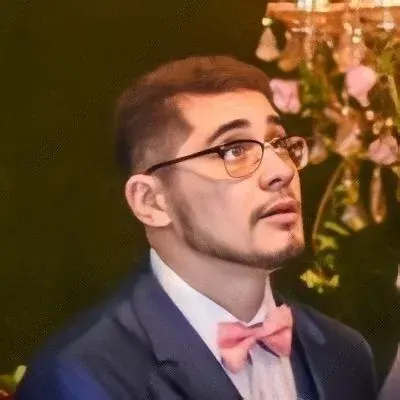
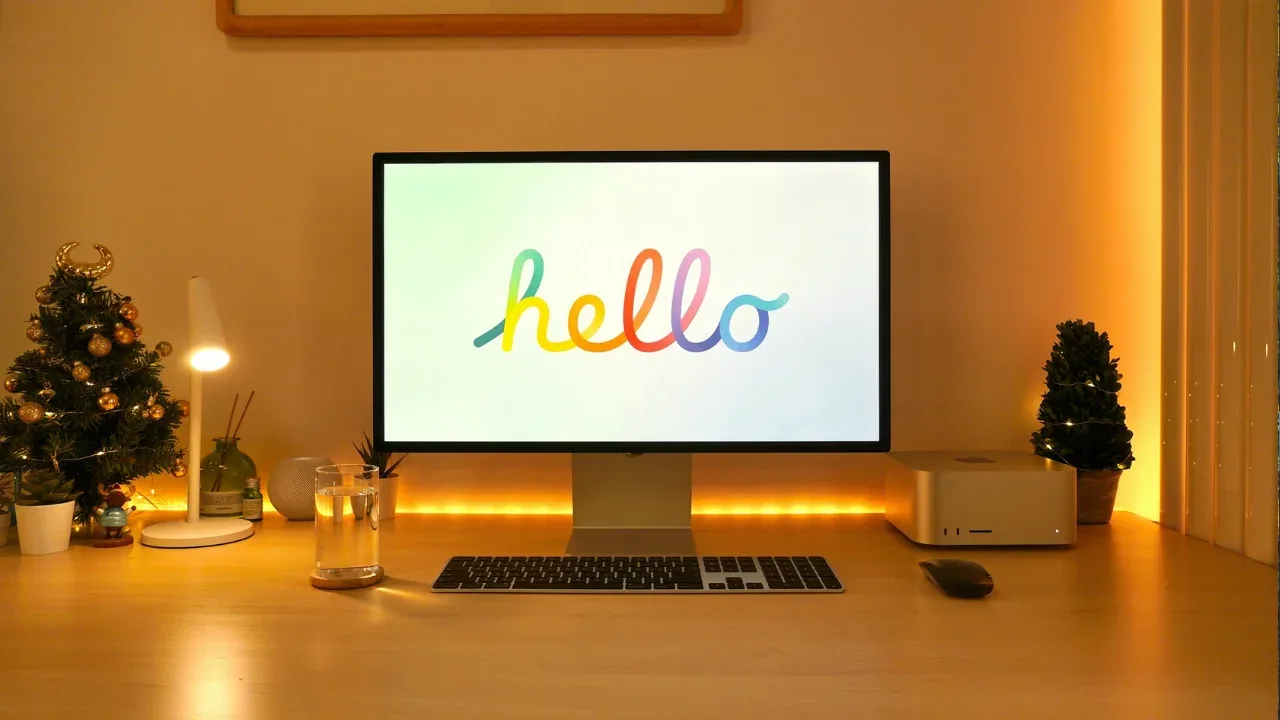
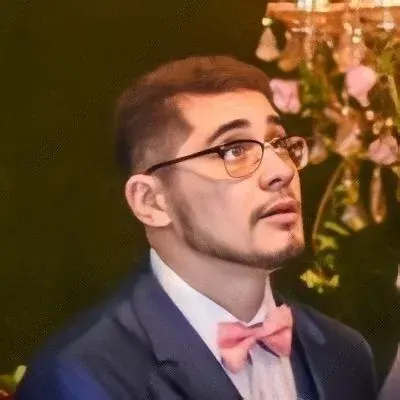
React - Changing an Uncontrolled Input: A Complete Guide
Are you confused about React's warning message that says "MyForm is changing an uncontrolled input to be controlled"? Don't worry, you're not alone! This warning can be a bit tricky to understand, but we're here to help you out.
Understanding the Error
Let's break down the error message first. React is warning you that your input element is switching from an uncontrolled state to a controlled state (or vice versa). But what does this even mean?
Controlled Input: In React, a controlled input is an input element whose value is controlled by the component's state. This means that the input's value is supplied by the component and any changes to the input's value are managed by the component as well.
Uncontrolled Input: On the other hand, an uncontrolled input is an input element whose value is managed by the DOM. In other words, the component doesn't keep track of the input's value directly.
Identifying the Issue
Based on your code snippet, it seems like you intended to have a controlled input. However, the warning suggests otherwise. To identify the issue, let's analyze your code.
import React from 'react';
export default class MyForm extends React.Component {
constructor(props) {
super(props);
this.state = {}
}
render() {
return (
<form className="add-support-staff-form">
<input name="name" type="text" value={this.state.name} onChange={this.onFieldChange('name').bind(this)}/>
</form>
)
}
onFieldChange(fieldName) {
return function (event) {
this.setState({[fieldName]: event.target.value});
}
}
}
export default MyForm;
The issue lies in the onChange
event handler of the input
element. You're invoking this.onFieldChange('name').bind(this)
to handle the event. This is where things go wrong.
Solving the Problem
To fix the error and ensure that your input remains controlled, you need to modify your event handler in two steps.
Step 1: Bind the event handler in the component's constructor
constructor(props) {
super(props);
this.state = {};
this.onFieldChange = this.onFieldChange.bind(this);
}
Step 2: Pass the input field name as a parameter to the event handler
<input name="name" type="text" value={this.state.name} onChange={this.onFieldChange('name')} />
Understanding the Solution
By binding the event handler in the constructor, you ensure that the this
keyword in the event handler refers to the component instance. Additionally, by passing the field name as a parameter, you allow the event handler to handle multiple input fields in a generic way.
Final Thoughts
React's warning message about changing an uncontrolled input can be puzzling, but once you understand the issue and implement the correct solution, you'll be able to control your inputs like a pro! Remember to bind the event handler in the constructor and pass the field name as a parameter to the event handler.
If you found this guide helpful, don't hesitate to share it with fellow developers who might be facing the same issue. And hey, if you have any other React questions or topics you'd like us to cover, let us know in the comments below! Happy coding! 💻🚀