React "after render" code?
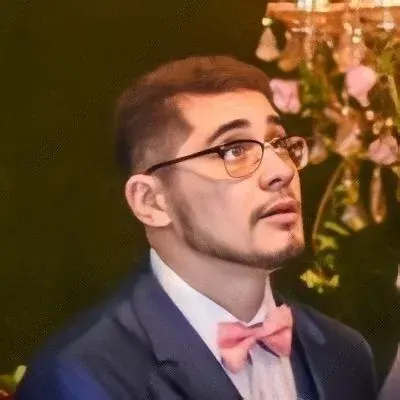
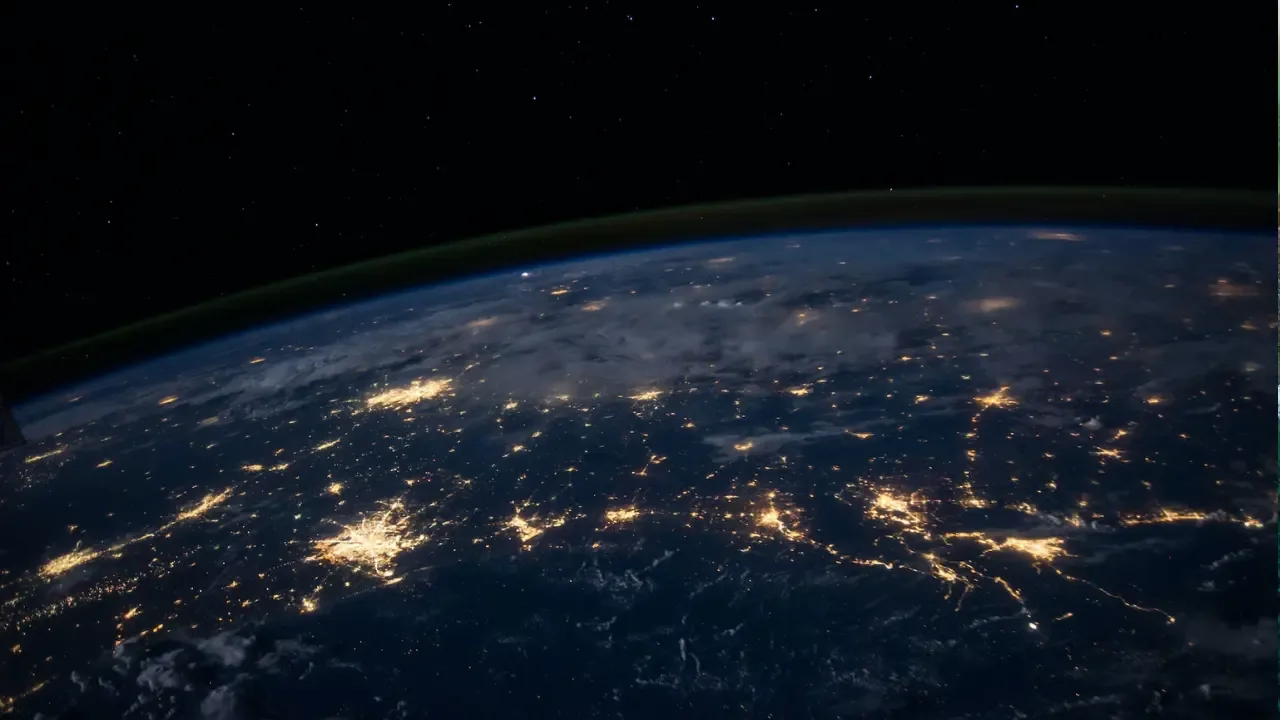
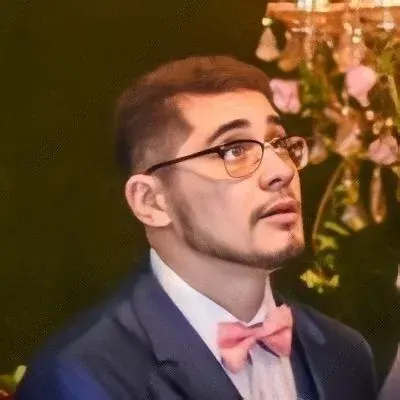
React "after render" code?
Are you struggling to find the right process for running code after rendering components in React? 🤔 Don't worry! We've got you covered! 💪
The Problem
Let's say you have an app where you need to set the height of an element dynamically, using the heights of other elements as reference. In vanilla JS, jQuery, or Backbone views, this task is relatively simple. But when it comes to React, things can get a bit trickier. So, how do you know when everything is rendered and where should you put the code to perform the height calculation? 🧐
The Solution
In React, you can leverage the component lifecycle methods to execute code after rendering. The method we're interested in is called componentDidMount
. This method gets called automatically by React after the component has been rendered and added to the DOM. It's the perfect place to perform any calculations or manipulate the DOM based on the rendered elements. 🎉
Example Implementation
In your case, to set the height of the "app-content" div based on the window size and other elements' heights, you can modify your AppBase
component like this:
var React = require('react');
var List = require('../list');
var ActionBar = require('../action-bar');
var BalanceBar = require('../balance-bar');
var Sidebar = require('../sidebar');
var AppBase = React.createClass({
componentDidMount: function() {
// Code to calculate the height of "app-content" and set it here
const appContent = document.querySelector('.app-content');
const actionBarHeight = document.querySelector('.action-bar').offsetHeight;
const balanceBarHeight = document.querySelector('.balance-bar').offsetHeight;
const windowHeight = window.innerHeight;
const newHeight = windowHeight - actionBarHeight - balanceBarHeight;
appContent.style.height = newHeight + 'px';
},
render: function () {
return (
<div className="wrapper">
<Sidebar />
<div className="inner-wrapper">
<ActionBar title="Title Here" />
<BalanceBar balance={balance} />
<div className="app-content">
<List items={items} />
</div>
</div>
</div>
);
}
});
module.exports = AppBase;
In the componentDidMount
method, we select the necessary elements, calculate the new height based on the window size and the other element heights, and then apply the new height to the "app-content" div.
Call to Action
Now that you know how to perform "after render" code in React, give it a try! Implement this solution in your project and see it in action. Don't let complicated rendering tasks hold you back! 😎
If you found this guide helpful, please share it with your fellow React developers and leave a comment with your thoughts and experiences. Let's grow together as a community! 🚀