Pros/cons of using redux-saga with ES6 generators vs redux-thunk with ES2017 async/await
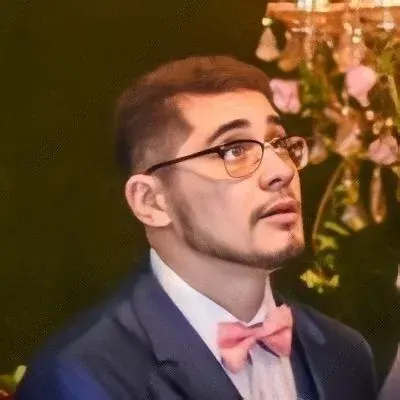
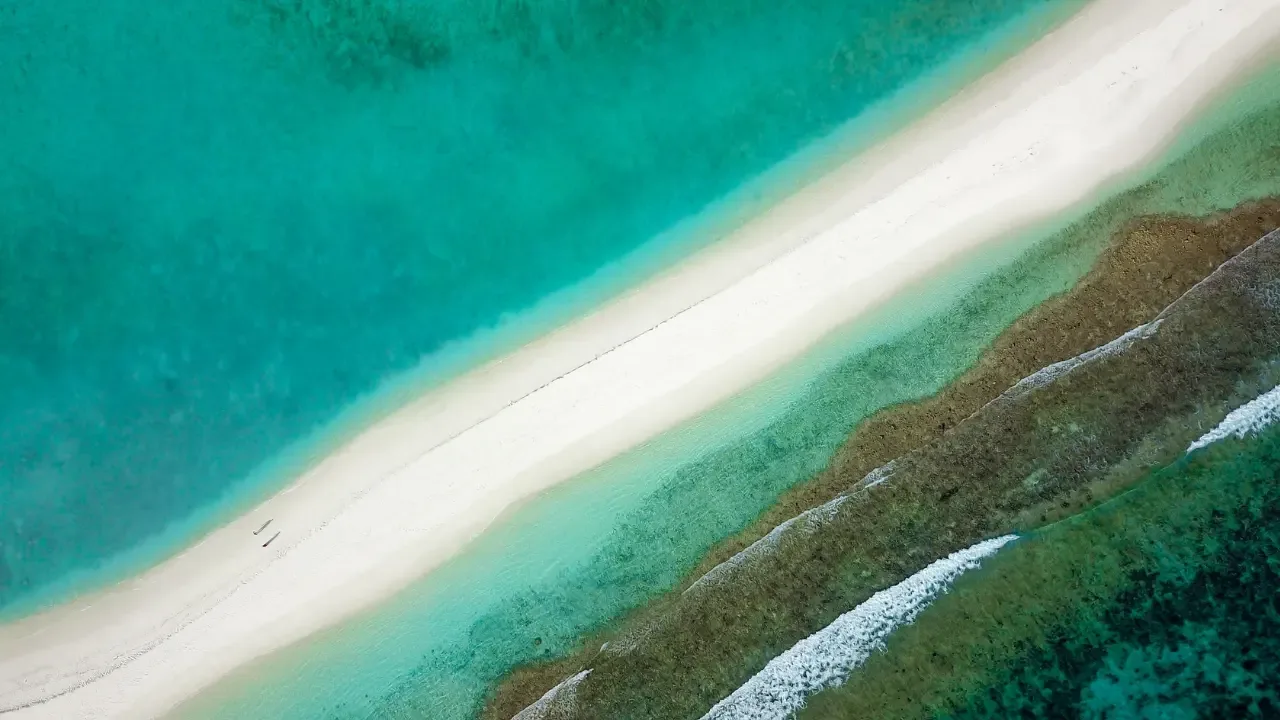
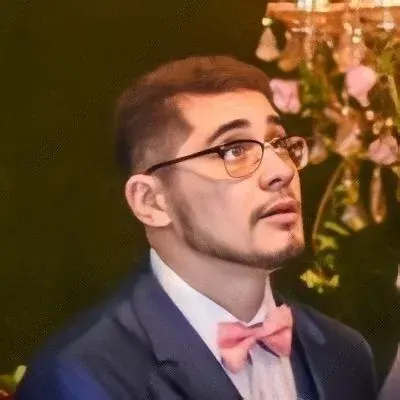
Should You Use redux-saga with ES6 Generators or redux-thunk with ES2017 async/await?
There's been a lot of buzz around redux-saga lately - it's the newest addition to the Redux family and it uses generator functions for listening to and dispatching actions. But before you jump into the saga world, let's first explore the pros and cons of using redux-saga with ES6 generators compared to the alternative approach of using redux-thunk with ES2017 async/await.
The Setup
To provide you with a better understanding of the comparison, let's take a look at an example component and its corresponding action creators using redux-thunk:
import { login } from 'redux/auth';
class LoginForm extends Component {
onClick(e) {
e.preventDefault();
const { user, pass } = this.refs;
this.props.dispatch(login(user.value, pass.value));
}
render() {
return (
<div>
<input type="text" ref="user" />
<input type="password" ref="pass" />
<button onClick={::this.onClick}>Sign In</button>
</div>
);
}
}
export default connect(state => ({}))(LoginForm);
And here are the actions using redux-thunk:
// auth.js
import request from 'axios';
import { loadUserData } from './user';
export const login = (user, pass) => async (dispatch) => {
try {
dispatch({ type: LOGIN_REQUEST });
let { data } = await request.post('/login', { user, pass });
await dispatch(loadUserData(data.uid));
dispatch({ type: LOGIN_SUCCESS, data });
} catch(error) {
dispatch({ type: LOGIN_ERROR, error });
}
}
// user.js
import request from 'axios';
export const loadUserData = (uid) => async (dispatch) => {
try {
dispatch({ type: USERDATA_REQUEST });
let { data } = await request.get(`/users/${uid}`);
dispatch({ type: USERDATA_SUCCESS, data });
} catch(error) {
dispatch({ type: USERDATA_ERROR, error });
}
}
// more actions...
The Pros and Cons
redux-saga with ES6 Generators
Pros:
Allows you to write structured and testable code by decoupling action creators (generators) from reducers.
Enables you to handle complex asynchronous flows, such as handling multiple actions in a specific order and pausing and resuming asynchronous operations.
Provides fine-grained control over side effects by using sagas, which are defined as separate functions.
Cons:
Requires a bit of a learning curve to understand generator functions and how they work with sagas.
Adds an extra layer of complexity to your codebase compared to redux-thunk.
Might be an overkill for smaller projects or simpler asynchronous flows.
redux-thunk with ES2017 async/await
Pros:
Simple and straightforward to use, especially if you're already familiar with ES2017 async/await.
Easy to understand and integrate into your existing Redux setup.
Works well for simpler asynchronous actions.
Cons:
Can lead to "callback hell" if you have nested async actions.
Limited control over asynchronous flows compared to redux-saga.
Could introduce potential performance issues if not used properly.
The Verdict
Both redux-saga with ES6 generators and redux-thunk with ES2017 async/await have their own advantages and disadvantages. The choice ultimately depends on the complexity of your application and the specific requirements of your asynchronous flows.
If you have a large, complex application and need more control over your side effects, redux-saga with ES6 generators might be the better choice. However, if you're working on a smaller project or dealing with simpler asynchronous actions, redux-thunk with ES2017 async/await could be a more practical option.
Remember, there's no one-size-fits-all solution. It's important to evaluate your project's needs and consider the trade-offs before making a decision.
Your Input Matters!
Have you used redux-saga or redux-thunk in your projects? What are your thoughts and experiences? Let's start a conversation in the comments section below and share your insights!
👇👇👇