Programmatically navigate using React router
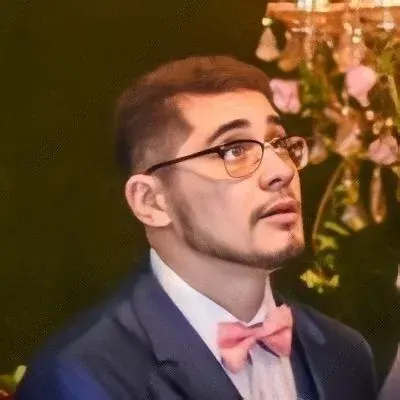
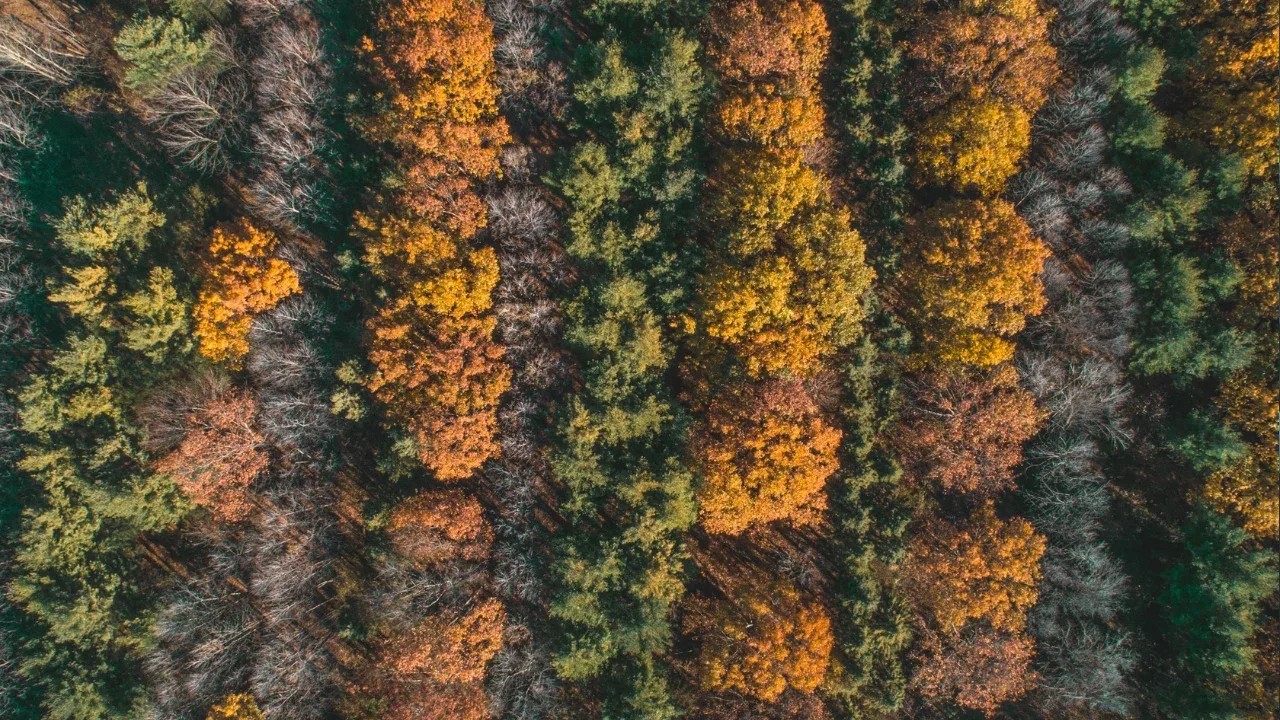
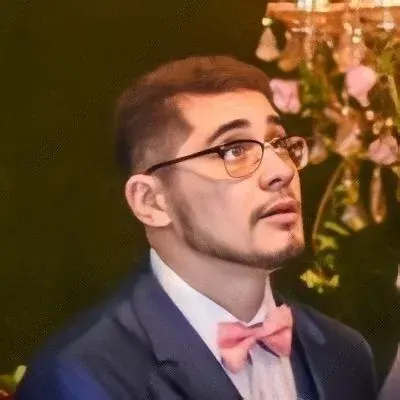
Navigating with React Router Like a Pro 🚀
Have you ever found yourself in a situation where you need to navigate programmatically using React Router but didn't know how? 🤔 Fret not, because I am here to guide you through the process and provide you with easy solutions. Let's dive right in! 💪
Understanding the Context API 🧐
Before we jump into the solution, let's take a moment to understand what this.context
refers to in the given context.
In React, the Context API allows you to pass data through the component tree without having to pass props down manually at every level. It becomes extremely useful when working with libraries like React Router, as it provides us with a way to access the router's functionality inside our components.
The Problem at Hand 🤔
In the given scenario, the user wants to perform navigation based on a dropdown selection instead of a traditional link. This requirement often arises in scenarios where you have complex UIs and need more control over the navigation flow.
The Solution 🙌
To achieve programmatically navigation using React Router, you can make use of the useHistory
hook from "react-router-dom"
. This hook gives you access to the history
object, which allows you to navigate dynamically.
Here's an example code snippet that demonstrates how to perform navigation programmatically based on a dropdown selection:
import { useHistory } from "react-router-dom";
function MyComponent() {
const history = useHistory();
const handleDropdownChange = (event) => {
const selectedOption = event.target.value;
// Perform the navigation based on the selected option
history.push(selectedOption);
}
// Rest of your component code
return (
<select onChange={handleDropdownChange}>
<option value="/option1">Option 1</option>
<option value="/option2">Option 2</option>
<option value="/option3">Option 3</option>
</select>
);
}
In this example, we import the useHistory
hook from react-router-dom
and initialize it in our component. When the dropdown value changes, we extract the selected option and use the history.push()
method to navigate to that particular route.
Avoiding Mixins 🚫
In the given context, you mentioned the Navigation
mixin but questioned whether it is possible to achieve the navigation without mixins. The good news is that with the latest versions of React Router, mixins are no longer required. You can now rely on the Context API and hooks, such as useHistory
, to achieve the desired navigation functionality.
Conclusion and Your Next Steps 🎉
Congratulations! You now have a solid understanding of how to perform programmatically navigation using React Router. Armed with the knowledge of the useHistory
hook and the power of the Context API, you can build more interactive and dynamic applications.
Feel free to experiment with different scenarios and explore the vast capabilities of React Router. Remember, understanding the problem and having the right tools at your disposal go a long way in solving any challenge.
Have you encountered similar scenarios in your React projects? How did you handle them? Share your experiences and insights with us in the comments below! Let's level up our React Router skills together. 💪🌟