Preview an image before it is uploaded
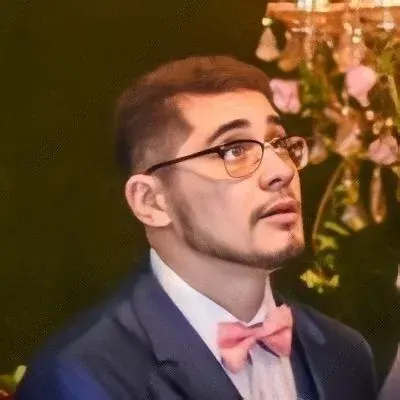
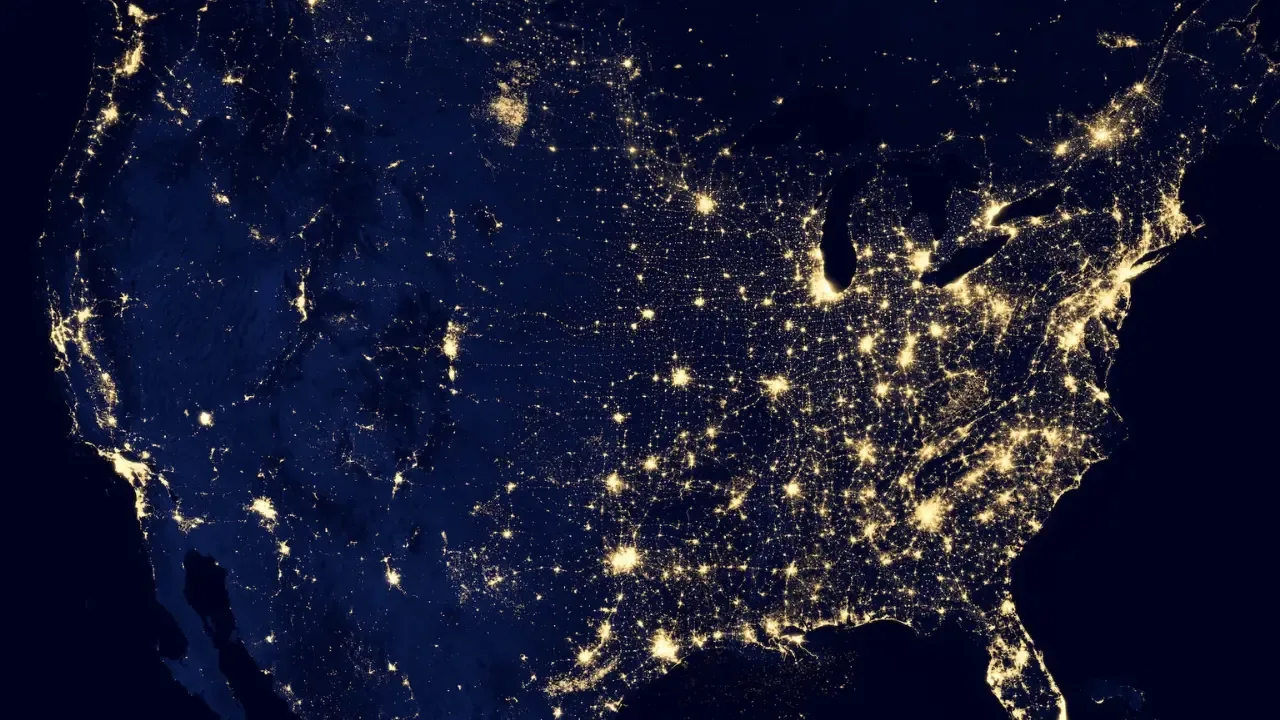
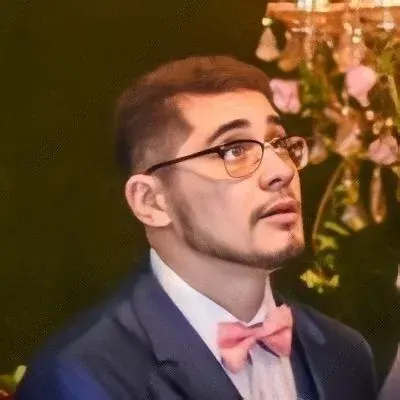
📸 Preview an Image Before Uploading: A Handy Guide 🖼️
So, you want to be able to preview an image before uploading it? No worries, my friend, I've got you covered! In this blog post, we'll address the common issues that arise when attempting to preview an image before it's uploaded, provide easy-peasy solutions, and encourage you to engage with our awesome community. Let's dive in! 💦
The Common Issue: Pre-upload Image Preview
The scenario is clear: you want to give users the ability to preview an image before they hit that famous "Upload" button. The twist? You want the entire preview action to take place right in the browser, without using Ajax to upload the image. Challenge accepted!
🏆 Solution 1: The FileReader API 📖
The FileReader API is a powerful tool that allows us to read files within the browser without uploading them to a server. Here's a simple step-by-step guide to implementing this solution:
Step 1: Handle the file input
First things first, you need an HTML input element of type "file" to let users choose the image they want to upload. Something like this:
<input type="file" id="image-input">
Step 2: Listen for changes
Next, we need to add an event listener to detect when the user selects an image. We'll then trigger our preview function. Check out this snippet:
const input = document.getElementById("image-input");
input.addEventListener("change", (event) => {
previewImage(event.target.files[0]);
});
Step 3: Preview the image
Finally, it's time to get our hands dirty and actually preview the image. Here's where the FileReader API comes into play:
function previewImage(file) {
const reader = new FileReader();
reader.onload = (event) => {
const preview = document.getElementById("image-preview");
preview.setAttribute("src", event.target.result);
};
reader.readAsDataURL(file);
}
Done! Now when a user selects an image, it will be displayed in an element with the "image-preview" ID. Magic, right? 🔮
🌟 Solution 2: The HTML5 File API 🎉
Another way to achieve the desired preview functionality is by using the HTML5 File API. This API provides us with some awesome methods and properties to interact with files. Here's how you can leverage it:
Step 1: Handle the file input (again)
You guessed it, we still need the HTML input element of type "file". We'll go with the same snippet as before:
<input type="file" id="image-input">
Step 2: Listen for changes (again)
No surprises here, we'll add the same event listener to our input element:
const input = document.getElementById("image-input");
input.addEventListener("change", (event) => {
previewImage(event.target.files[0]);
});
Step 3: Read the file
Time to dive deeper into the File API. We'll use the FileReader
class to read the contents of our image file:
function previewImage(file) {
const reader = new FileReader();
reader.onload = (event) => {
const preview = document.getElementById("image-preview");
preview.setAttribute("src", event.target.result);
};
reader.readAsDataURL(file);
}
Wait, what? Isn't this the same code as Solution 1? Yep, you're right! The FileReader API is actually part of the HTML5 File API. So, two solutions, one API, infinite possibilities! 🌈
💫 Engage with Our Community! 🗣️
Congratulations, my tech-savvy friend, you're now equipped with not one, but two brilliant solutions to preview an image before uploading. 💪 But don't stop here! Share your thoughts, experiences, and creative twists with our vibrant community below. We'd love to hear from you! 👇
Have questions? Spot a bug? Let us know, and together, we'll keep pushing the boundaries of what's possible in the tech world. Happy coding! 🚀