Prevent redirection of XMLHttpRequest
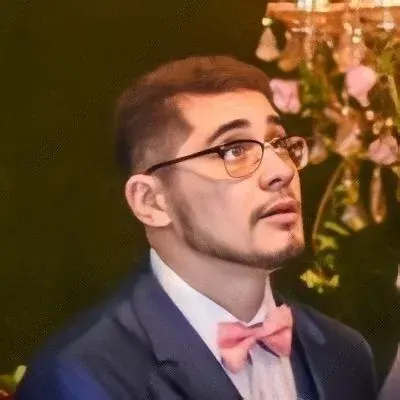
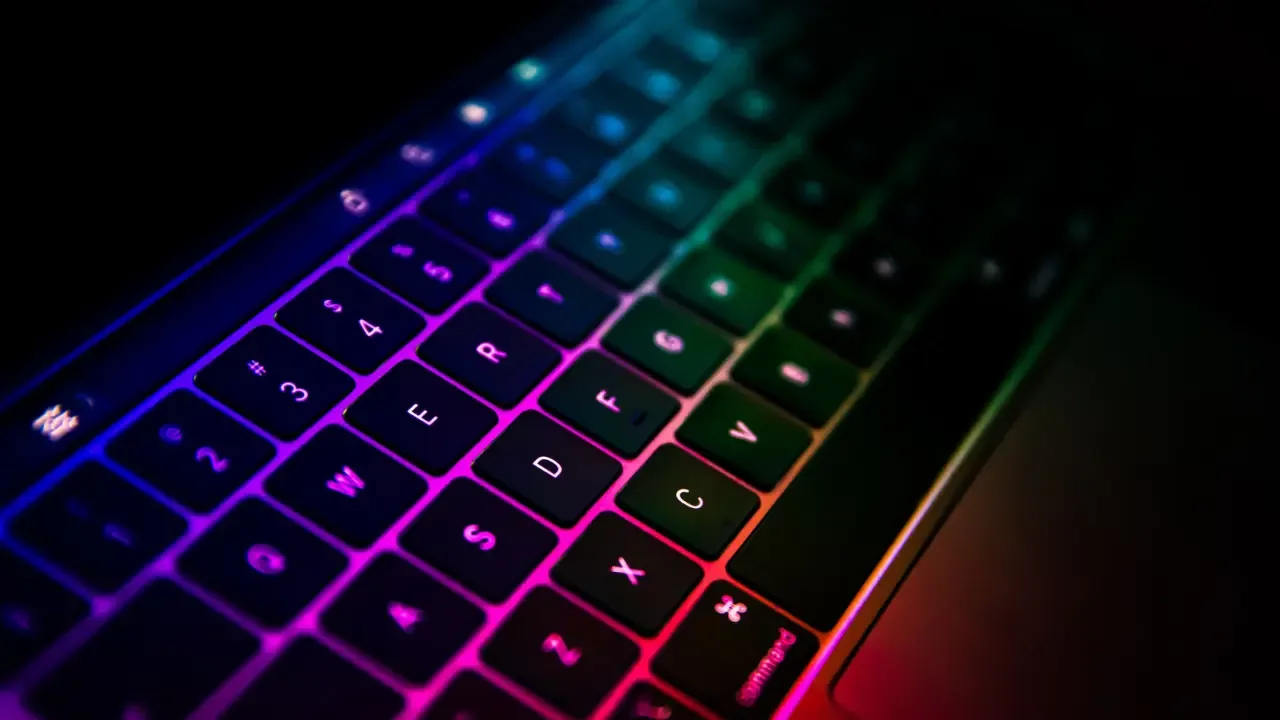
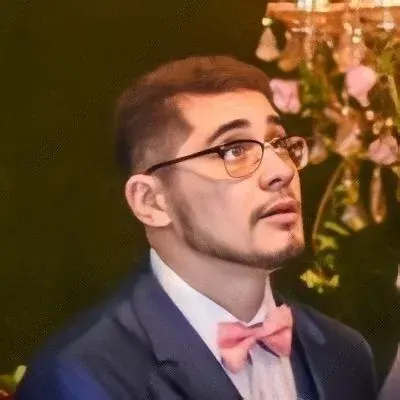
šTitle: Unleash the Power of XMLHttpRequest: Preventing Redirection with Ease!
š Hey there, tech enthusiasts! We've got an interesting topic to discuss today ā preventing redirection of XMLHttpRequest. š«š
Have you ever wondered if it's possible to stop your browser from automatically following redirects when sending XMLHttpRequests? Well, we've got the answers for you! In this guide, we'll address common issues, provide easy solutions, and empower you to take control of your redirects. Let's dive in! šŖ
š¤ Why Redirection Matters
Imagine you send an XMLHttpRequest to a server, expecting a specific response. However, instead of the desired response, your browser obediently follows a redirect and returns a completely different result. š± This can lead to confusion, incorrect data processing, and headaches. Fret not, for we have solutions to keep you in control.
š ļø Common Issue: Obtaining Redirect Status Code
One common need is to obtain the redirect status code so that you can handle it yourself. This allows you to take appropriate actions based on the redirection. Luckily, with a bit of JavaScript magic, we can quickly get the desired status code.
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (this.readyState === 4 && this.status >= 300 && this.status < 400) {
const redirectStatus = this.status;
// Handle the redirect status code as per your requirements š¦
}
};
xhr.open("GET", "https://example.com/some-api", true);
xhr.send();
By checking the status
of the XMLHttpRequest object during its onreadystatechange
event, we can grab the redirect status code. This empowers you to handle the redirection in a way that suits your needs. š
š§ Solution: Preventing Redirection
But what if you want to completely prevent the browser from following redirects? Well, we've got your back! š
By setting the XMLHttpRequest
object's followRedirects
property to false
, you can instruct your browser to bypass any redirections. This way, you can access the redirect status code and handle the situation yourself.
const xhr = new XMLHttpRequest();
xhr.followRedirects = false; // Prevent the browser from following redirects
xhr.onreadystatechange = function() {
if (this.readyState === 4 && this.status >= 300 && this.status < 400) {
const redirectStatus = this.status;
// Handle the redirect status code as per your requirements š¦
}
};
xhr.open("GET", "https://example.com/some-api", true);
xhr.send();
Now, the power is in your hands! š
š” Pro Tip: Be Mindful of Cross-Origin Requests
Keep in mind that when dealing with XMLHttpRequests, you may encounter Cross-Origin Resource Sharing (CORS) restrictions. CORS rules might prevent you from accessing the data due to security measures implemented by the server. Ensure that you have appropriate access permissions and CORS handling in place.
⨠Engage with the Community
We hope this guide has empowered you to prevent redirection and take control of your XMLHttpRequests. š
Do you have any other tips, tricks, or problems related to XMLHttpRequests and redirection? š¤ Share them in the comments below! Let's collaborate and learn from each other's experiences.
Remember, sharing is caring! If you found this guide helpful, spread the love by sharing it with your fellow techies. Together, we can master the art of preventing redirection with ease! š«šā¤ļø
Happy coding! š»āļø