Prevent browser caching of AJAX call result
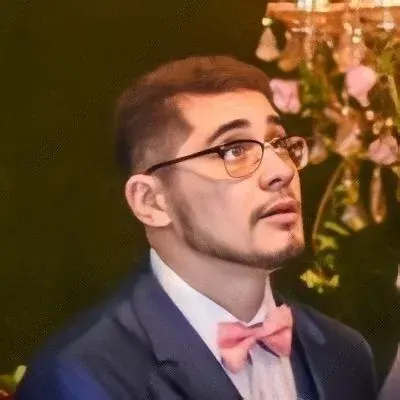
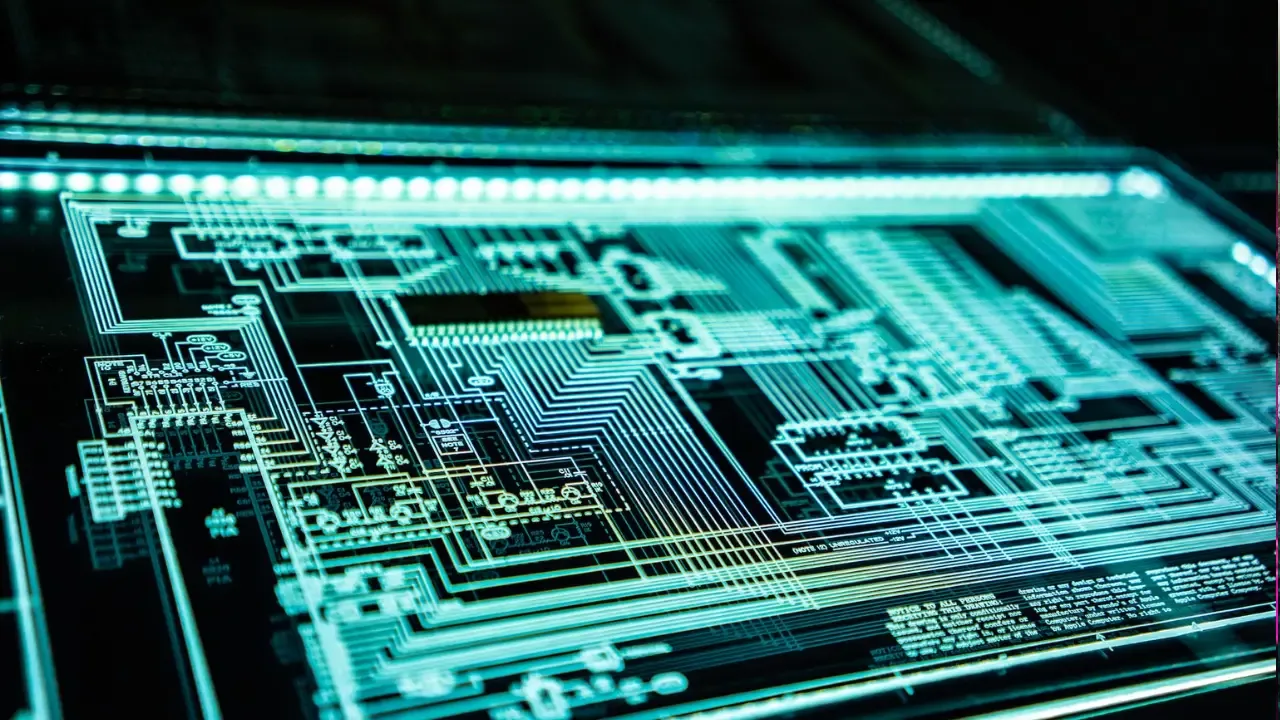
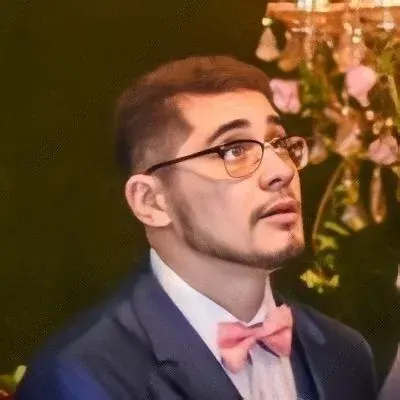
Prevent Browser Caching of AJAX Call Result: A Simple Guide 🚫🔄
Do you often find yourself frustrated with cached AJAX call results in your browser? 🤦♂️ You're not alone! Many developers face this common issue when loading dynamic content using the $.get()
function. 😩 But fear not, we've got you covered with easy solutions to prevent browser caching and streamline your development workflow! 🙌
The Problem 🤔
So, what's the deal with cached AJAX call results? When you make an AJAX request, the browser sometimes stores the response in its cache. Subsequent requests to the same URL might fetch the cached response instead of making a fresh request to the server. While this can sometimes be beneficial for performance, it can also cause issues when you want real-time updates or dynamic content. 😕
The Hacky Solution 🎭
One common workaround is to append a random string to the URL, forcing the browser to treat each request as unique. In the context of the original question, this hack is achieved by adding new Date().toString()
in the QueryString. However, this approach might feel like a temporary fix and lacks elegance. 🙈
A More Elegant Solution 💡
Fortunately, there are better alternatives to prevent browser caching while maintaining cleaner code. Let's explore a few options:
1. Disable Caching Globally 🌍
To prevent caching across your entire application, you can set appropriate headers in the server's response. By including these headers, you instruct the browser to fetch a fresh copy of the content on every request. Here's an example in PHP:
header("Cache-Control: no-cache, must-revalidate");
header("Expires: Sat, 26 Jul 1997 05:00:00 GMT");
Similarly, other server-side languages provide ways to control caching using headers. You can consult the documentation for your specific server technology to implement the equivalent solution.
2. Set Caching Headers for Specific AJAX Calls 🎛️
If you only want to prevent caching for specific AJAX requests, you can modify the server response headers accordingly. By doing so, you maintain control over caching behavior on a more granular level. Here's an example in Node.js using Express.js:
app.get("/your-ajax-endpoint", (req, res) => {
res.setHeader("Cache-Control", "no-cache, no-store, must-revalidate");
res.setHeader("Pragma", "no-cache");
res.setHeader("Expires", "0");
// your response logic here
});
Again, similar techniques can be applied in other server-side frameworks or languages.
3. Use jQuery $.ajaxSetup()
📦
If you're using jQuery, which seems to be the case here, you can leverage the $.ajaxSetup()
function to set default options for all your AJAX requests. By disabling caching globally, you ensure consistent behavior throughout your application. Here's an example:
$.ajaxSetup({
cache: false
});
Choose Your Path, Fellow Developer! 🛤️
Now that you have some elegant solutions to prevent browser caching of AJAX call results, it's time to choose the path that suits your needs best. Whether you disable caching globally, set caching headers selectively, or utilize jQuery's $.ajaxSetup()
, you are well-equipped to conquer this issue! 💪
If you have any other tips or suggestions on preventing browser caching, be sure to drop them in the comments below. Let's build a caching-free web together! 🌐❌🔄
Stay updated with more awesome tech guides and follow us on Twitter for daily tips and tricks!
Happy coding! 💻🔥⌨️