Pretty printing XML with javascript
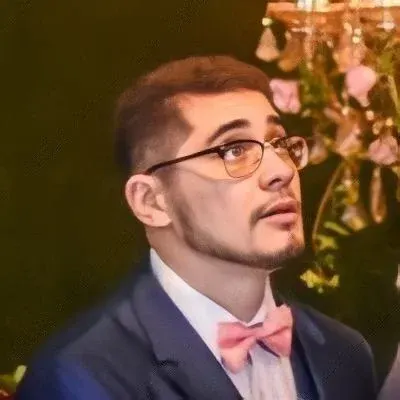
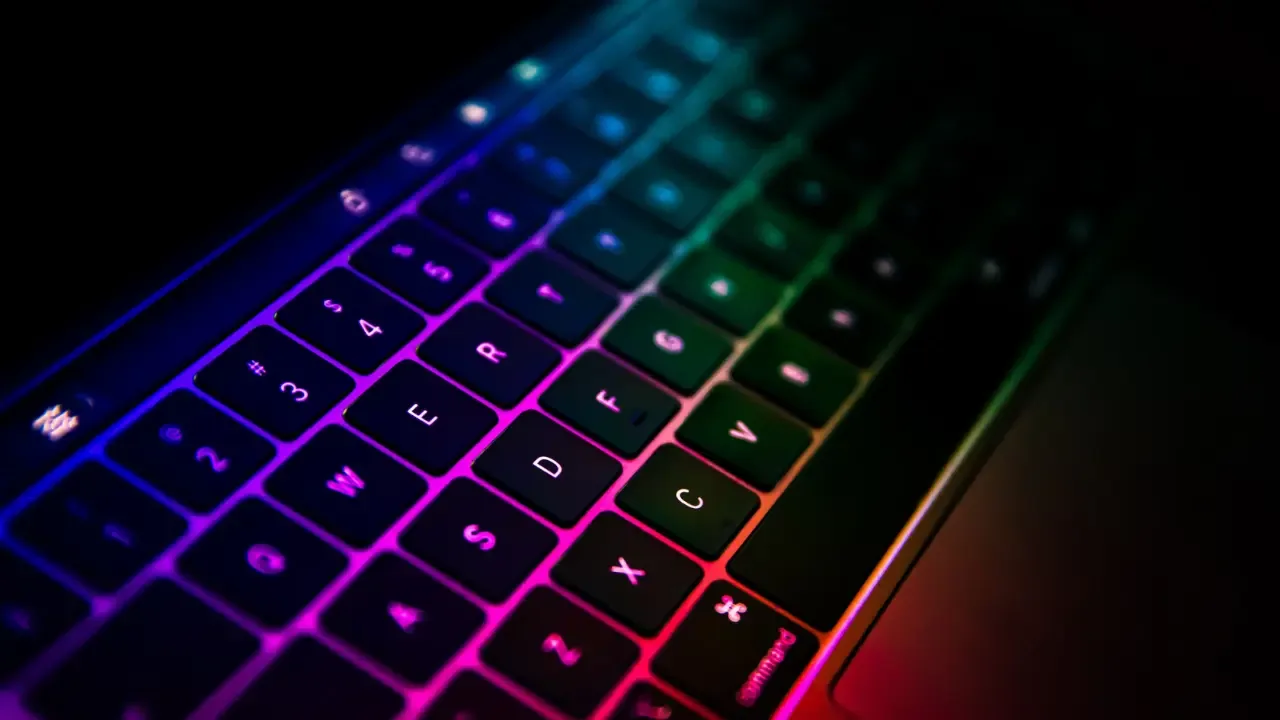
🔍 Pretty Printing XML with JavaScript: The Easy Way! 🔍
If you've ever encountered the challenge of dealing with non-indented XML and wanted to "pretty-print" it for easier readability, you're in luck! In this blog post, we'll explore a common issue faced by many developers and provide you with an easy solution using JavaScript. 🎉
The Problem: You have a string that represents non-indented XML, and you want to transform it into a nicely formatted XML structure. For example:
<root><node/></root>
should become:
<root>
<node/>
</root>
The Solution:
To tackle this problem, we'll first transform the XML to add carriage returns and white spaces. Then, we'll use the <pre>
tag to output the XML. Let's take a look at a JavaScript function that does the job:
function formatXml(xml) {
var formatted = '';
var reg = /(>)(<)(\/*)/g;
xml = xml.replace(reg, '$1\r\n$2$3');
var pad = 0;
jQuery.each(xml.split('\r\n'), function(index, node) {
var indent = 0;
if (node.match(/.+<\/\w[^>]*>$/)) {
indent = 0;
} else if (node.match(/^<\/\w/)) {
if (pad != 0) {
pad -= 1;
}
} else if (node.match(/^<\w[^>]*[^\/]>.*/)) {
indent = 1;
} else {
indent = 0;
}
var padding = '';
for (var i = 0; i < pad; i++) {
padding += ' ';
}
formatted += padding + node + '\r\n';
pad += indent;
});
return formatted;
}
To use this function, simply call it like this:
jQuery('pre.formatted-xml').text(formatXml('<root><node1/></root>'));
And voila! You will see the prettily-formatted XML printed inside the <pre>
element with the class formatted-xml
.
But wait, there's more! 🚀
While the provided solution works perfectly fine, you might be wondering if there's a better way to accomplish this task. And the answer is yes, there are JavaScript frameworks and plugins that can simplify the process for you. Let's explore a few options:
vkBeautify: A small and lightweight JavaScript library that beautifies XML, HTML, and JSON. It provides various options and customization features to fit your specific needs.
Prettier: Although primarily known for code formatting, Prettier also supports XML formatting. It is highly customizable and widely adopted in the JavaScript community.
js-beautify: Another popular JavaScript library that can beautify XML and other languages. It comes with built-in defaults but allows for extensive customization.
These frameworks and plugins can save you time and effort by providing ready-to-use solutions for pretty-printing XML. Feel free to explore them and choose the one that suits your project requirements.
Now it's your turn! 💡
Have you ever faced XML formatting challenges? How did you solve them? Share your experiences, tips, and tricks in the comments below. We would love to hear from you and learn more about your XML adventures! 🗣️💬
So go ahead, give it a try, and make your XML more readable than ever before! Happy coding! 💻🌈✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
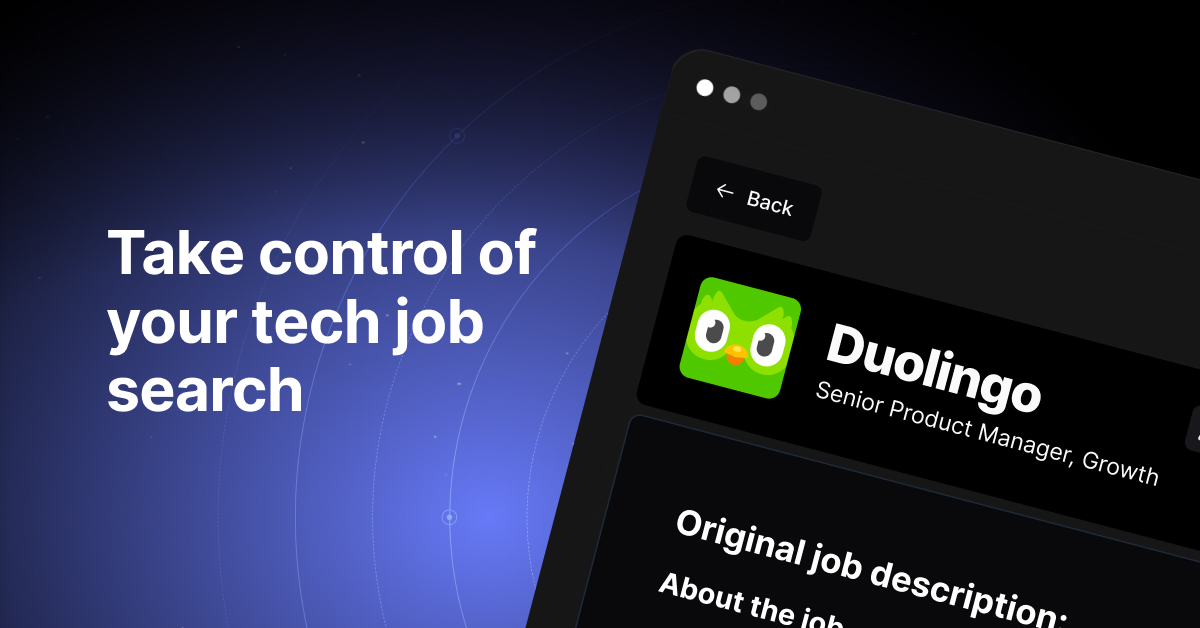