Preloading images with jQuery
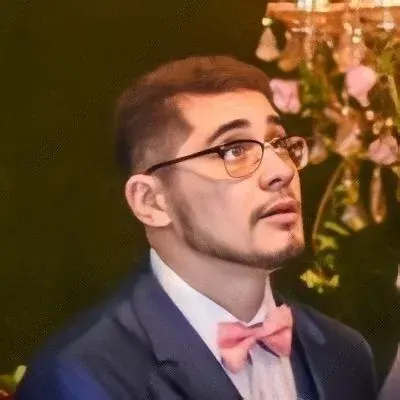
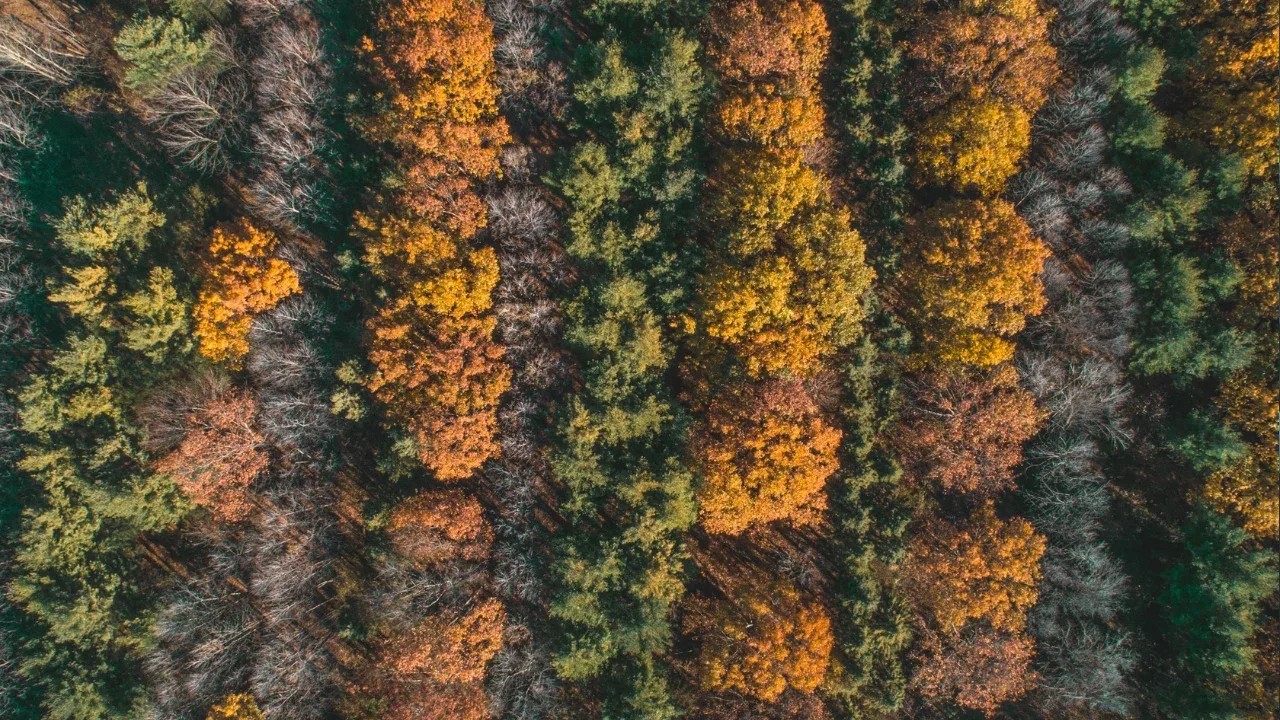
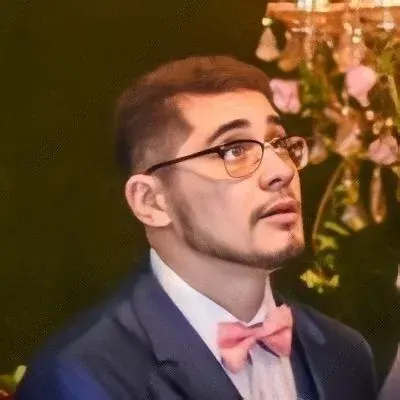
Preloading Images with jQuery Made Easy! 😎
Are you looking for a quick and easy way to preload images with JavaScript, specifically using jQuery? You're in the right place! Preloading images is essential to improve the user experience on your website by reducing flickering or delays when images are loaded dynamically. In this blog post, we'll explore a simple solution to preload images using jQuery and provide you with an easy-to-use code snippet. Let's dive in! 💪
The Over-the-Top Solution 😰
The example you found may seem a bit complex for your needs. Don't worry; we've got you covered! Let's break it down and find a simpler solution. The first code snippet you shared looks like this:
function complexLoad(config, fileNames) {
for (var x = 0; x < fileNames.length; x++) {
$("<img>").attr({
id: fileNames[x],
src: config.imgDir + fileNames[x] + config.imgFormat,
title: `The ${fileNames[x]} nebula`
}).appendTo(`#${config.imgContainer}`).css({ display: "none" });
}
};
While this solution is functional, it might be a bit overwhelming if you only need a quick and easy way to preload images. Let's explore a simpler alternative! 🙌
The Quick, Easy, and Short Solution ⚡
To preload images using jQuery in a simpler way, you can make use of the $.ajax
function. Here's a streamlined code snippet that will do the job:
function preloadImages(images) {
const promises = [];
images.forEach((image) => {
const promise = $.ajax({ url: image, type: "GET" });
promises.push(promise);
});
return Promise.all(promises);
}
In this code snippet, we define a function called preloadImages
that takes an array of image URLs as its parameter. We create an empty array called promises
to store each AJAX request promise. Then, using a loop, we iterate over the images
array and send an AJAX request for each image URL. The resulting promise is added to the promises
array.
Finally, we return a Promise.all(promises)
, which enables us to wait for all the image preloading requests to complete before executing any further code. By using this approach, you can easily preload multiple images with just a few lines of code!
Time to Take Action! 🚀
Now that you have a simple solution to preload images with jQuery, it's time to take action and implement it on your website. Follow these steps to get started:
Identify the images you want to preload.
Create an array of image URLs.
Call the
preloadImages
function, passing the array of image URLs as an argument.Handle the resolved promise returned by
preloadImages
to execute further actions, such as displaying the preloaded images.
With this easy-to-use code snippet, you can achieve image preloading efficiently and enhance the user experience on your website.
Share Your Success Story! 🌟
We hope this blog post helped you find a quick and easy solution to preload images with jQuery. Now, we want to hear from you! Did this solution work for you? How did it impact your website's performance? Share your success story in the comments below and let's engage in a conversation about improving the user experience through image preloading.
Remember, the power of preloading images is in your hands! Go ahead and implement this simple technique to make your website blazing fast and ensure your visitors have a seamless experience.
Happy preloading! 🖼️🚀