Possible to extend types in Typescript?
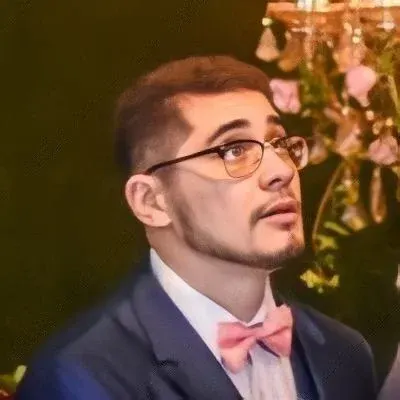
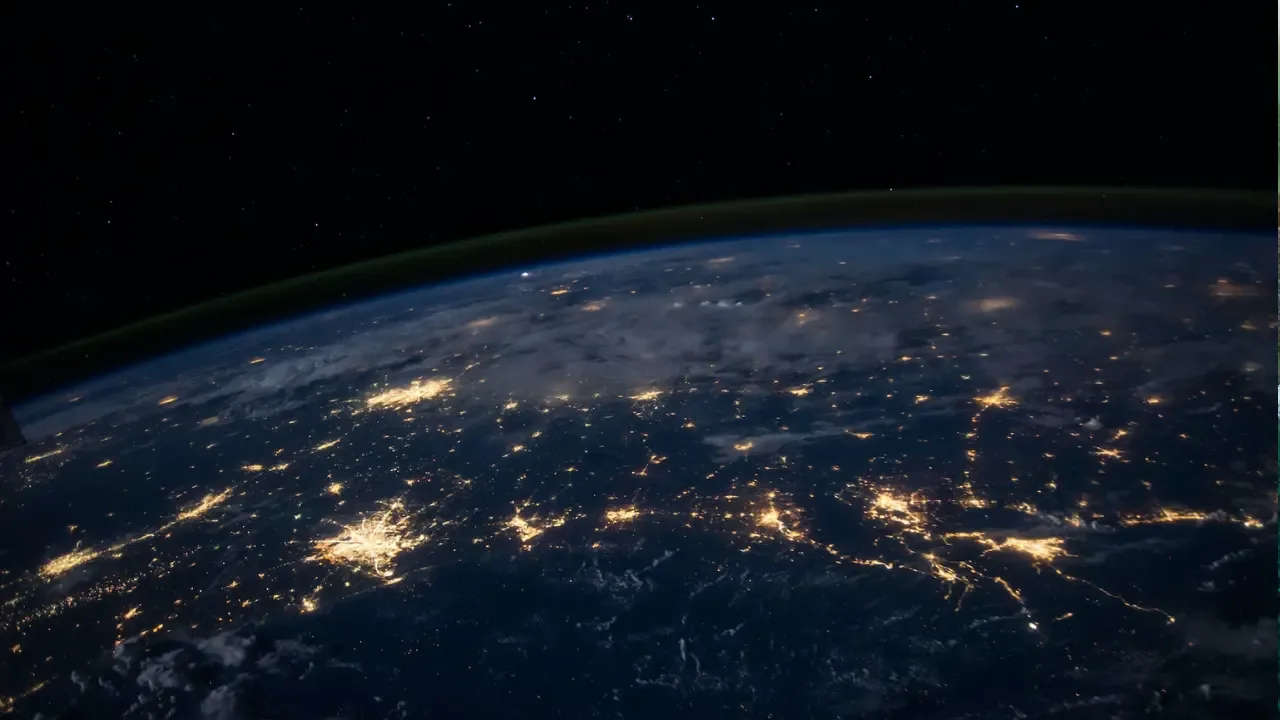
Can We Extend Types in TypeScript? 🤔
Hey there, tech-savvy folks! 👋 Today, we're going to dive into a common question among TypeScript enthusiasts: Is it possible to extend types in TypeScript? 🧐 We're here to provide you with easy solutions, examples, and answers that will make your coding life a whole lot easier! So, let's roll up our sleeves and get started! 💪
Understanding the Problem 🤔
Let's set the stage with a common scenario. Say we have a juicy Event
type that looks like this:
type Event = {
name: string;
dateCreated: string;
type: string;
}
But now, we want to spruce things up and extend this type to include some user-specific information. We might naturally try something like the following code snippet:
type UserEvent extends Event = {
userId: string;
}
However, our initial excitement quickly fades away when we realize that this code doesn't work. 😩 So, what's the deal here?
The Limitations of Extending Types ✋
In TypeScript, type extension at the level you might be expecting is not directly supported. While TypeScript provides us with powerful mechanisms to create complex types, extending one type directly from another is not one of them. But don't worry! TypeScript got your back, and we have some alternative solutions for you! 😉
Alternative Solutions 💡
Solution 1: Intersection Types 🌍
To achieve the desired extension effect, we can use intersection types. Instead of extending Event
, we can create a new type that combines both Event
and any additional properties we want to include. Here's an example:
type UserEvent = Event & {
userId: string;
}
By using the &
operator, we can merge the properties of the Event
type with our custom userId
property, effectively "extending" the Event
type.
Solution 2: Type Composition 👯♂️
Another way to tackle this is through type composition. Rather than extending an existing type, we can create a new type that combines the properties of both Event
and our custom type. Here's an example:
type UserEvent = {
userId: string;
} & Event;
By reversing the order of the types and combining them with the &
operator, we achieve a similar outcome. This type composition approach allows us to keep the Event
properties separate from our additional properties while still achieving the "extension" effect.
Take Action and Level Up Your TypeScript Game! 🚀
Now that you know the ins and outs of extending types in TypeScript, it's time to put this knowledge into practice! Give these alternative solutions a spin in your own projects and see the power they bring to your codebase. 🔥
Remember, the beauty of TypeScript lies in its flexibility and versatility, allowing you to conquer those complex types and build robust applications with ease!
If you found this blog post helpful, don't forget to share it with your fellow developers. Let's spread the TypeScript love! ❤️
Have any other TypeScript questions or topics you'd like us to explore? Leave a comment below and let's keep the conversation going! 👇
Happy coding, TypeScript enthusiasts! ⌨️💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
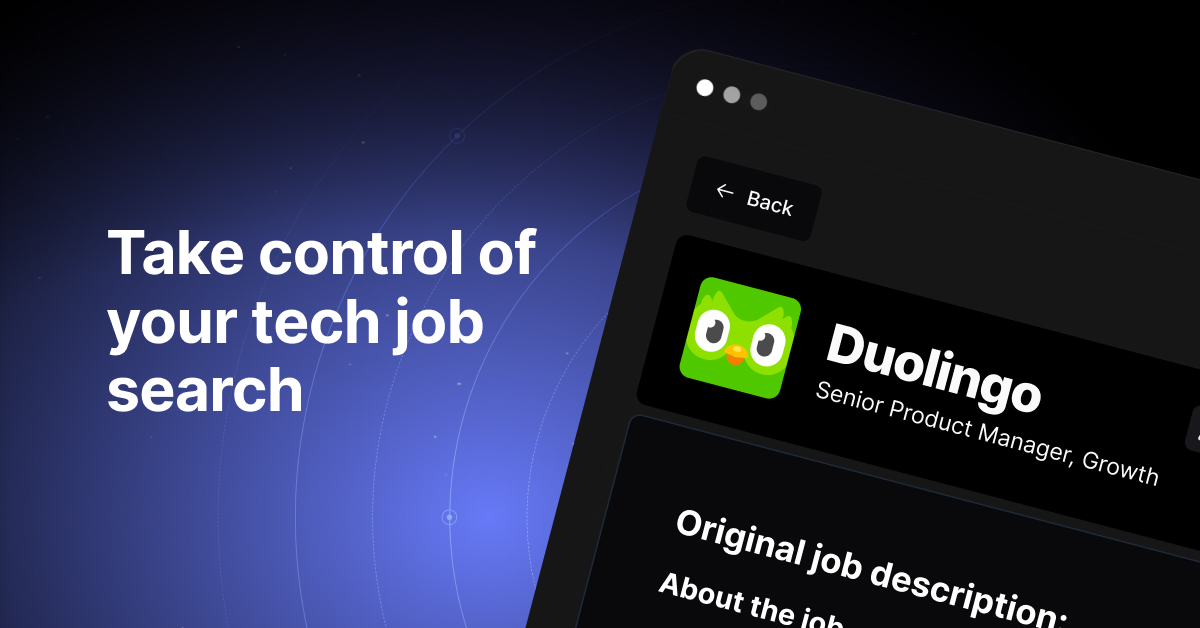