Passing data between controllers in Angular JS?
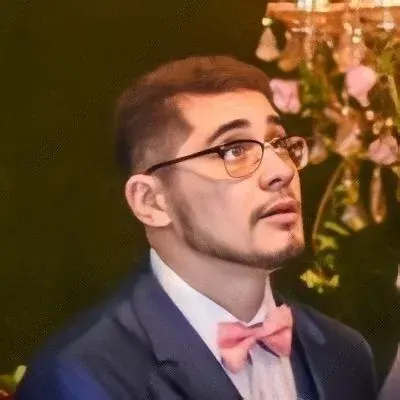
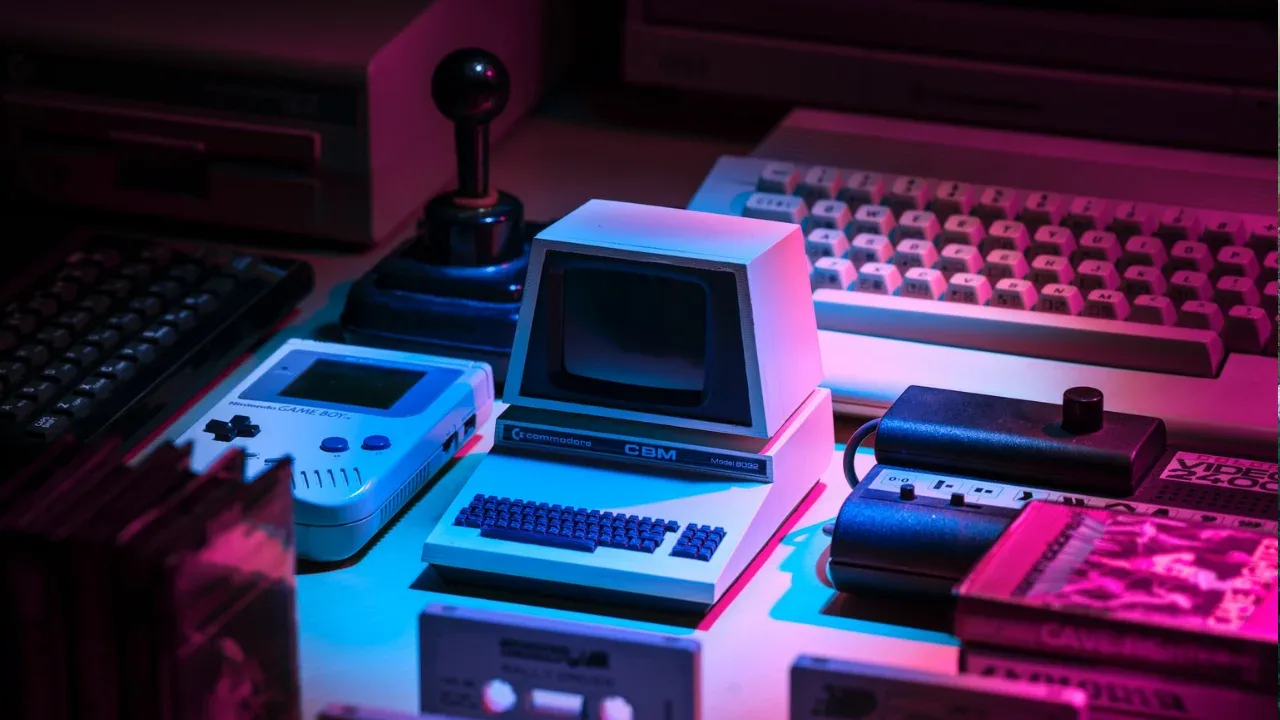
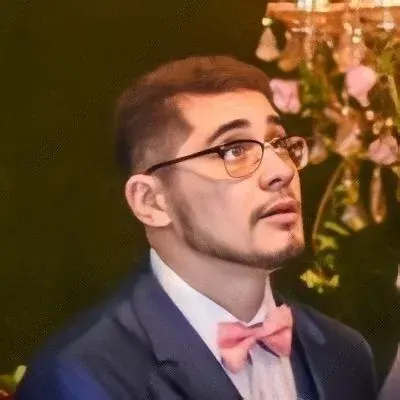
Passing Data Between Controllers in Angular JS: A Complete Guide ๐จโ๐ป๐ก
Are you struggling with passing data between controllers in your Angular JS application? ๐ค Don't worry, we've got you covered! In this blog post, we'll address common issues and provide easy solutions to help you pass data seamlessly. Let's dive in! ๐โโ๏ธ
The Setup โ
Let's start with the context. You have a basic controller, ProductCtrl
, that fetches and displays products using a factory called $productFactory
. Your main challenge is passing the clicked products to another controller, for example, the CartCtrl
, where the added products will be displayed. Here's an example of the code you provided:
App.controller('ProductCtrl', function($scope, $productFactory) {
$productFactory.get().success(function(data) {
$scope.products = data;
});
});
// HTML code
<ul>
<li ng-repeat="product as products">
{{product.name}}
</li>
</ul>
<ul class="cart">
<li>
<!-- Clicked product should be added here -->
</li>
<li>
<!-- Clicked product should be added here -->
</li>
</ul>
Solution: Using Services and $broadcast ๐กโจ
To solve this problem, we'll use services and the $broadcast
method to send the clicked product data from the ProductCtrl
to the CartCtrl
. Here are the steps:
Step 1: Create a Service ๐ ๏ธ
First, we need to create a service that will handle the communication between controllers. In this case, let's call it CartService
. The service will have a variable to store the clicked products and a method to broadcast the data to all interested listeners (controllers). Here's an example of how to create the service:
App.service('CartService', function() {
var clickedProducts = [];
this.addClickedProduct = function(product) {
clickedProducts.push(product);
};
this.getClickedProducts = function() {
return clickedProducts;
};
this.clearClickedProducts = function() {
clickedProducts = [];
};
});
Step 2: Update the Click Event Handling ๐ฑ๏ธ
To handle click events on the products, we'll use a directive. Here's an example of how to update the HTML code to incorporate the directive:
<ul>
<li ng-repeat="product in products" ng-click="addToCart(product)">
{{product.name}}
</li>
</ul>
Step 3: Update the Product Controller ๐ฉโ๐ป๐
Now, let's update the ProductCtrl
to utilize the CartService
and $broadcast
method. Here's an example of how to modify the controller:
App.controller('ProductCtrl', function($scope, $productFactory, CartService) {
$productFactory.get().success(function(data) {
$scope.products = data;
});
$scope.addToCart = function(product) {
CartService.addClickedProduct(product);
$scope.$broadcast('productAddedToCart');
};
});
Step 4: Update the Cart Controller ๐๐
Finally, let's update the CartCtrl
to listen to the broadcasted event and update the view accordingly. Here's an example of how to update the controller:
App.controller('CartCtrl', function($scope, CartService) {
$scope.cartItems = [];
$scope.$on('productAddedToCart', function() {
$scope.cartItems = CartService.getClickedProducts();
});
// Other functionalities of CartCtrl
});
Step 5: Update the DOM ๐ฅ๏ธ๐ฅ
To display the clicked products dynamically in the cart, we can use the ng-repeat
directive. Here's an example of how to update the HTML code for the cart:
<ul class="cart">
<li ng-repeat="item in cartItems">
{{item.name}}
</li>
</ul>
Conclusion and Call-to-Action ๐๐
And that's it! By following these steps, you can easily pass data between controllers in Angular JS using a service and the $broadcast
method. Now you can display the clicked products dynamically in the cart view. ๐
We hope this guide has been helpful to you! If you have any questions or faced any issues during the process, feel free to leave a comment below. Happy coding! ๐ปโจ
PS: Did you find this guide useful? Share it with your fellow developers who might be struggling with passing data between Angular JS controllers! Let's help each other grow! ๐๐ค