Pass props to parent component in React.js
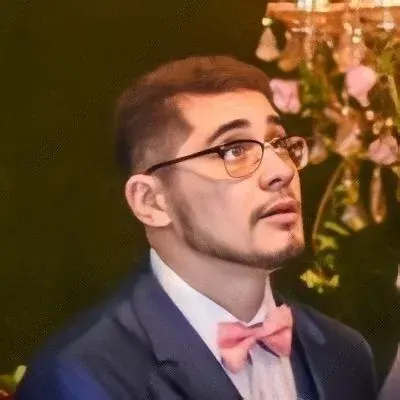
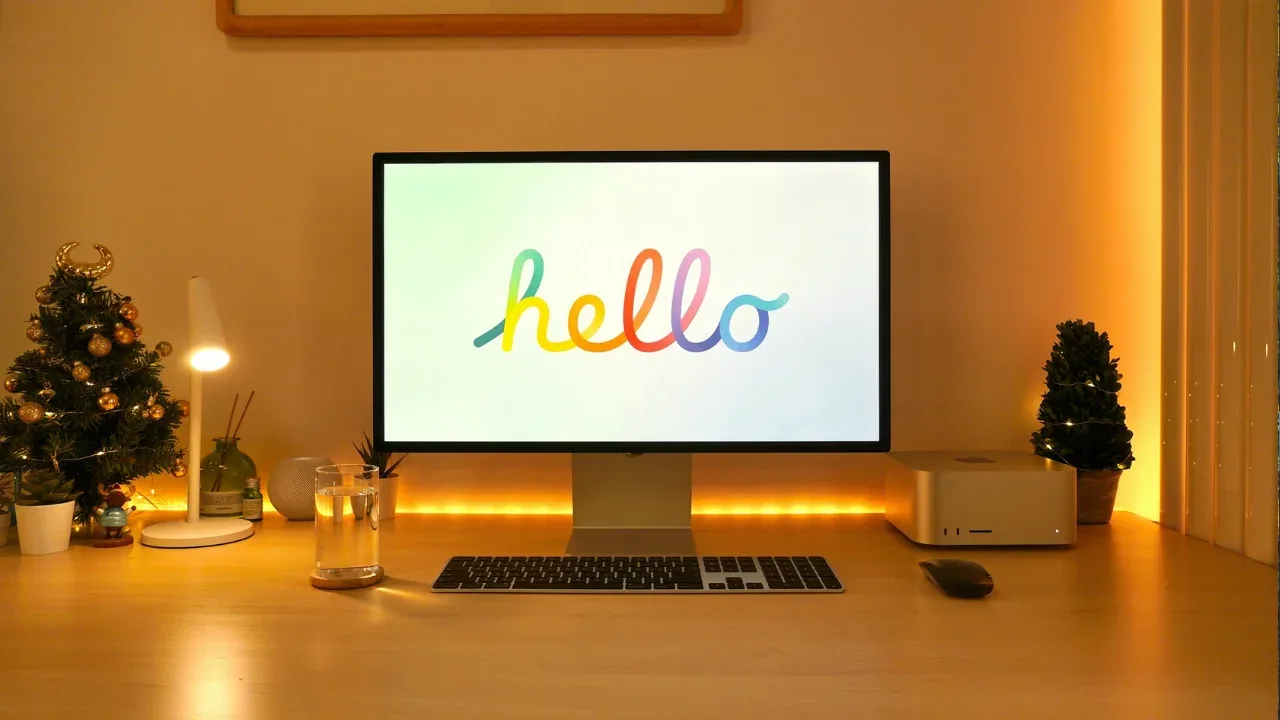
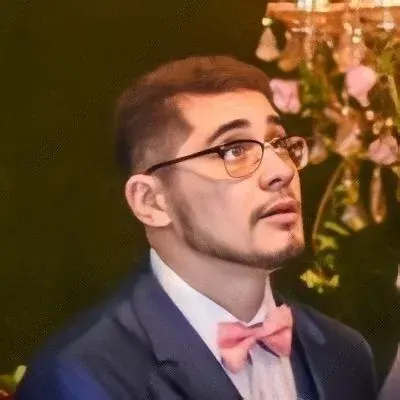
How to Pass Props to Parent Component in React.js
Have you ever found yourself in a situation where you wanted to pass a child component's props to its parent in React.js? 🤔 It may seem like a tricky problem at first, but fear not! We have easy solutions for you. Let's dive into it! 💪
The Context Behind the Question
The code snippet you provided showcases a common scenario. The Child component has an onClick event that needs to pass information to its parent, the Parent component. However, accessing the child's props directly from the event is not as straightforward as we may initially expect. 😕
var Child = React.createClass({
render: function() {
<a onClick={this.props.onClick}>Click me</a>
}
});
var Parent = React.createClass({
onClick: function(event) {
// event.component.props ?why is this not available?
},
render: function() {
<Child onClick={this.onClick} />
}
});
The React Way: Lifting State Up
Before we dive into solutions, it's important to understand the React way of handling data flow. In React, the recommended approach is to lift state up. 🚀 This means that the state and relevant logic should reside in the closest common ancestor of the components that need to communicate.
Solution 1: Callback Functions
One common solution to pass props from a child to its parent is by using callback functions. 👥 You can define a callback function in the parent component and pass it as a prop to the child component. The child component can then invoke the callback function, passing the desired props as arguments.
Let's see how we can implement this approach:
// Child.js
function Child(props) {
return (
<a onClick={() => props.onClick(props.someProp)}>Click me</a>
);
}
// Parent.js
function Parent() {
const handleChildClick = (someProp) => {
// Do something with someProp
};
return (
<Child onClick={handleChildClick} someProp="Hello, World!" />
);
}
In this example, we define the handleChildClick
function in the parent component and pass it as a prop named onClick
to the child component. When the child component is clicked, it invokes the onClick
callback with the desired prop as an argument.
Solution 2: Context API
Starting from React version 16.3, the Context API was introduced as a built-in solution for sharing state between components that are not directly connected. 🎉
The Context API allows you to create a context object in a common ancestor component and then provide and consume the values of that context in any descendant component.
Here's how you can use the Context API to pass props from a child to its parent:
// Parent.js
const ChildContext = React.createContext();
function Parent() {
return (
<ChildContext.Provider value={/* value you want to pass */}>
<Child />
</ChildContext.Provider>
);
}
// Child.js
function Child() {
return (
<ChildContext.Consumer>
{(value) => (
<a onClick={() => handleChildClick(value)}>Click me</a>
)}
</ChildContext.Consumer>
);
}
In this example, we create a context object using the React.createContext()
function in the parent component. We then provide the value we want to pass from the child component to the parent by wrapping the child component with the ChildContext.Provider
component.
In the child component, we consume the context value by using the ChildContext.Consumer
component. We can access the context value in the rendering function of the consumer.
The React Way Prevails
After using React for a while, it's important to acknowledge that passing child components as arguments to functions in parents is not the recommended approach. As stated before, the React way is to lift state up and maintain the relevant logic in the closest common ancestor. It promotes code organization, reusability, and scalability. 🌟
Spread the Knowledge!
Now that you have learned two easy solutions to pass props from a child component to its parent in React.js, why not share your newfound knowledge with others? 🌐 If you found this blog post helpful, make sure to share it on your favorite social media platforms and encourage others to engage with it. Let's spread the React love and help everyone become better developers together! 🚀✨