Parse JSON in JavaScript?
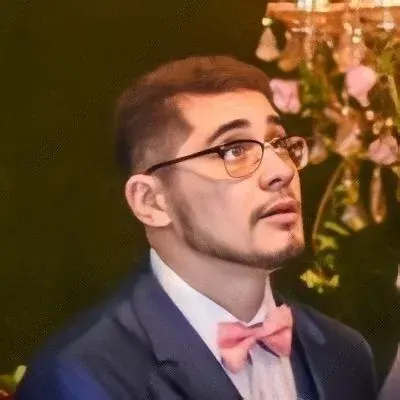
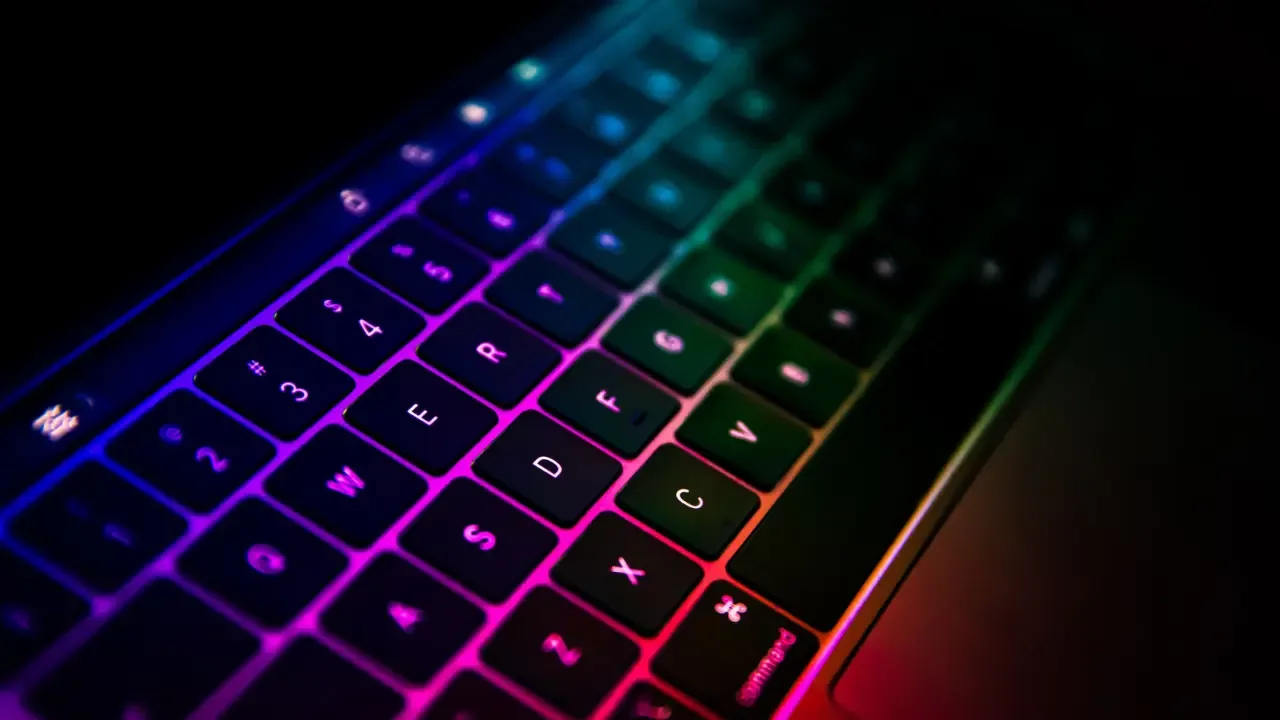
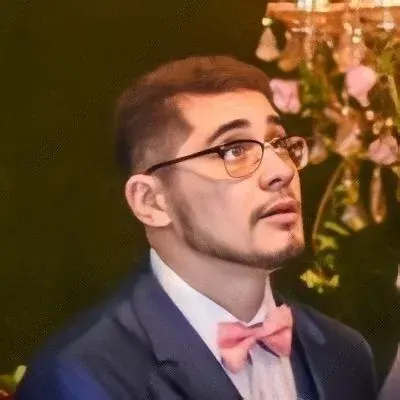
How to 🌪️ Parse JSON in JavaScript?
Are you struggling to parse a JSON string in JavaScript? 🤔 Don't worry, we've got you covered! In this guide, we'll discuss common issues faced while parsing JSON and provide you with easy solutions. So, let's dive in and decode that JSON! 🎉
The JSON String
Before we get started, let's take a look at the JSON string you're trying to parse:
var response = '{"result":true,"count":1}';
Accessing Values in JSON
To retrieve the values of result
and count
from the JSON string, you can follow these steps:
Parsing the JSON: The first step is to parse the JSON string using the
JSON.parse()
method. This method converts a JSON string into a JavaScript object, allowing you to access its properties easily.
var responseObject = JSON.parse(response);
Accessing
result
andcount
: Now that you've parsed the JSON string, you can access theresult
andcount
values using dot notation or bracket notation:
Using dot notation:
var result = responseObject.result;
var count = responseObject.count;
Using bracket notation:
var result = responseObject['result'];
var count = responseObject['count'];
Example
Let's put it all together! Here's a complete code example:
var response = '{"result":true,"count":1}';
var responseObject = JSON.parse(response);
var result = responseObject.result;
var count = responseObject.count;
console.log(result); // true
console.log(count); // 1
Troubleshooting
Encountering any issues while parsing JSON? Here are some common problems you might face and their solutions:
Invalid JSON: Ensure that your JSON string is valid. Even a small error, like a missing comma or incorrectly placed quotes, can cause parsing to fail.
Catching Errors: Wrap your parsing code in a try-catch block to handle any potential errors. This way, if an error occurs during parsing, you can gracefully handle it.
try {
var responseObject = JSON.parse(response);
} catch (error) {
console.error('Error parsing JSON:', error);
}
Share Your Thoughts! 💬
Now that you know how to parse JSON in JavaScript, give it a try! Share your experience with us in the comments below. We'd love to hear about any other JavaScript topics or issues you'd like us to cover next. Happy coding! 😄