Parse Error: Adjacent JSX elements must be wrapped in an enclosing tag
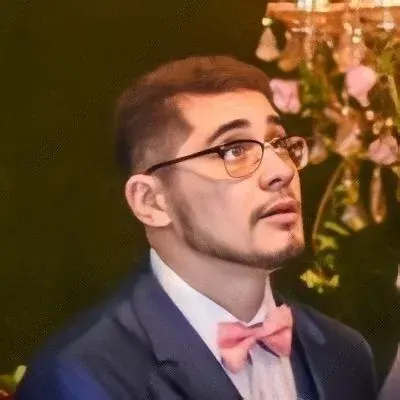
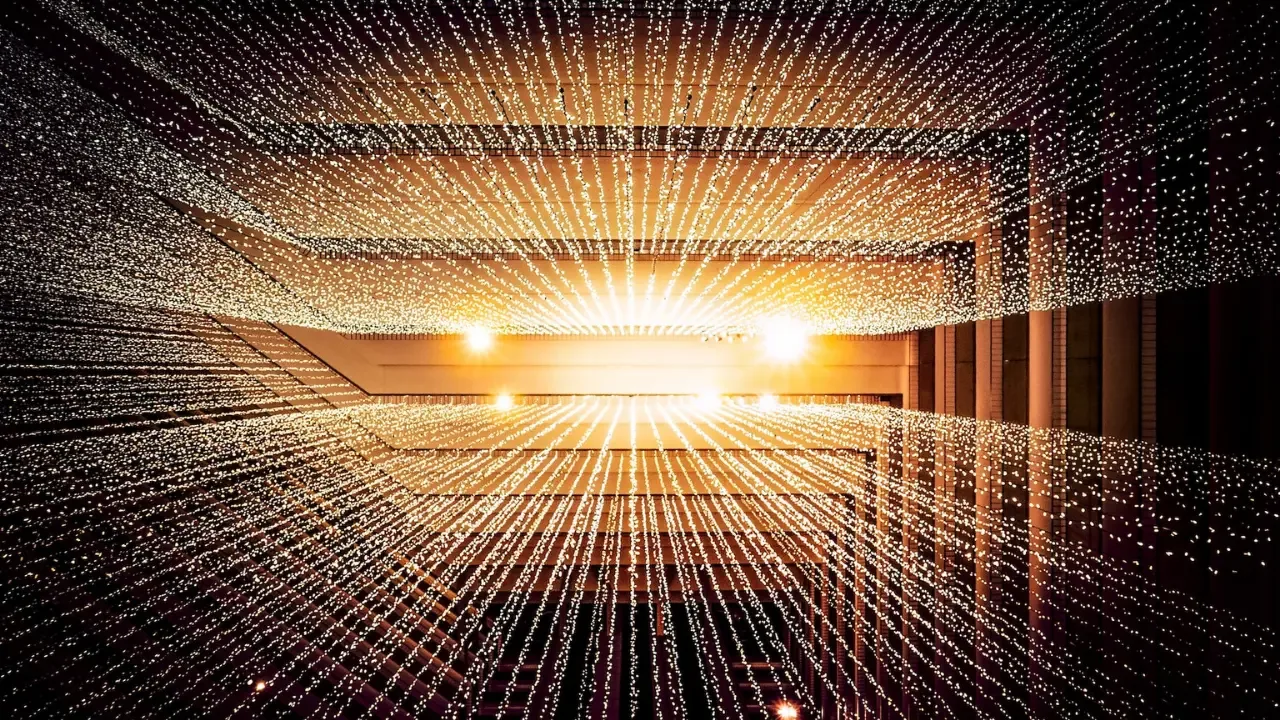
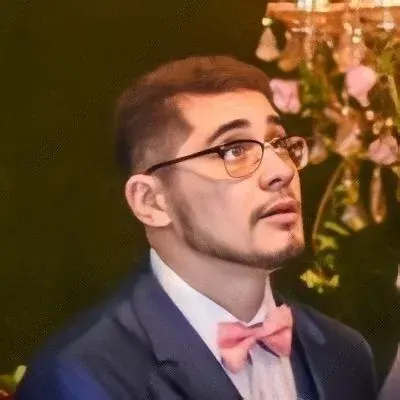
How to Fix the "Parse Error: Adjacent JSX elements must be wrapped in an enclosing tag" Issue in React.js
If you've encountered the "Parse Error: Adjacent JSX elements must be wrapped in an enclosing tag" error while working with React.js, don't worry! This error occurs when you have multiple JSX elements that are not wrapped within a common parent element. In this guide, we'll explain the issue and provide easy solutions to help you resolve it.
Understanding the Problem
In the provided code snippet, the issue originates from the fact that the JSX elements within the if
statement are not enclosed within a single parent element. This causes a conflict because React requires adjacent JSX elements to have a common parent element.
To illustrate the problem, let's take a closer look at the code snippet:
render: function() {
var text = this.state.submitted ? 'Thank you! Expect a follow up at ' + email + ' soon!' : 'Enter your email to request early access:';
var style = this.state.submitted ? {"backgroundColor": "rgba(26, 188, 156, 0.4)"} : {};
return (
<div>
if (this.state.submitted == false) {
<input type="email" className="input_field" onChange={this._updateInputValue} ref="email" value={this.state.email} />
<ReactCSSTransitionGroup transitionName="example" transitionAppear={true}>
<div className="button-row">
<a href="#" className="button" onClick={this.saveAndContinue}>Request Invite</a>
</div>
</ReactCSSTransitionGroup>
}
</div>
)
},
As indicated in the comment, the important portion causing the issue is the if (this.state.submitted == false)
statement. The subsequent JSX elements within the if
block, such as the <input>
and <div>
, need to be enclosed within a common parent element.
Solution: Wrapping JSX Elements in an Enclosing Tag
To fix the "Parse Error: Adjacent JSX elements must be wrapped in an enclosing tag" issue, you need to wrap the JSX elements within the if
block in a single parent element. Here's an updated version of the code with the issue resolved:
render: function() {
var text = this.state.submitted ? 'Thank you! Expect a follow up at ' + email + ' soon!' : 'Enter your email to request early access:';
var style = this.state.submitted ? {"backgroundColor": "rgba(26, 188, 156, 0.4)"} : {};
return (
<div>
{this.state.submitted == false && (
<>
<input type="email" className="input_field" onChange={this._updateInputValue} ref="email" value={this.state.email} />
<ReactCSSTransitionGroup transitionName="example" transitionAppear={true}>
<div className="button-row">
<a href="#" className="button" onClick={this.saveAndContinue}>Request Invite</a>
</div>
</ReactCSSTransitionGroup>
</>
)}
</div>
)
},
In the updated code, we have introduced a React Fragment (<>...</>
) as the enclosing tag for the JSX elements within the if
block. This allows multiple adjacent JSX elements to be wrapped without introducing additional DOM elements.
Conclusion
The "Parse Error: Adjacent JSX elements must be wrapped in an enclosing tag" error is common when working with React.js. By ensuring that your JSX elements have a common parent element, you can resolve this issue easily.
Hopefully, this guide has helped you understand the problem and provided a clear solution using React Fragments. Start implementing this fix and say goodbye to the pesky parse error!
Have you encountered any other React.js errors in your projects? Share your experiences and let's troubleshoot them together! 😊