Overriding interface property type defined in Typescript d.ts file
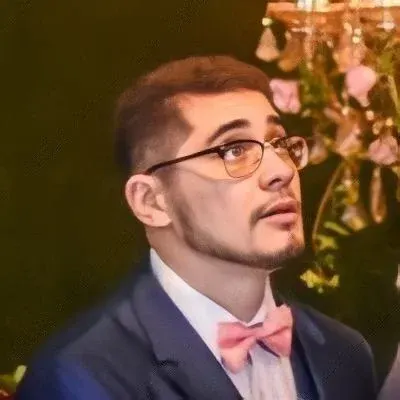

š» Overriding interface property type defined in Typescript d.ts file
Are you struggling with overriding a type defined in a Typescript .d.ts
file? Don't worry, we've got you covered! š¤
š¤ The Problem
You may find yourself in a situation where you want to change the type of a property defined in an interface from a .d.ts
file. Let's say you have the following interface defined in x.d.ts
:
interface A {
property: number;
}
But, for whatever reason, you need to change the type of property
to Object
in your Typescript files, like this:
interface A {
property: Object;
}
Or maybe you want to extend interface A
with a new interface and change the type there:
interface B extends A {
property: Object;
}
However, when you've tried these approaches, they didn't work as expected. š«
š ļø The Solution
The reason it didn't work is that .d.ts
files are declaration files, used to provide type information about external libraries or modules. They're meant for type checking, not for changing the behavior or structure of the code.
To solve this issue, you can create your own types or interfaces in your Typescript files, rather than attempting to modify existing ones defined in .d.ts
files.
Here's an example of how you can achieve what you want:
// x.d.ts file
interface A {
property: number;
}
// app.ts file
interface MyA extends A {
property: Object;
}
By creating a new interface (MyA
) that extends the original interface (A
), you can override the type of property
as desired.
Remember, when using this approach, make sure to use the new interface (MyA
) in your code wherever you need to use the modified property type.
š£ Take Action!
Now that you've learned how to override interface property types in Typescript, go ahead and give it a try in your own code! š
If you found this guide helpful, don't forget to share it with your fellow developers. And if you have any further questions or comments, we'd love to hear from you! š¬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
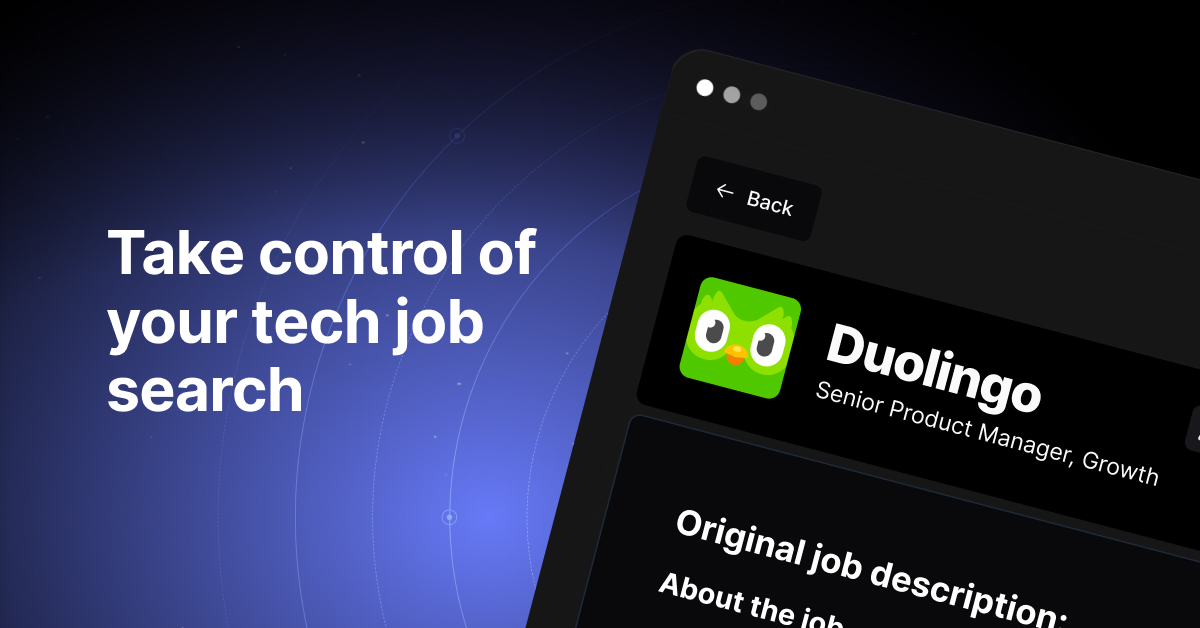