Origin <origin> is not allowed by Access-Control-Allow-Origin
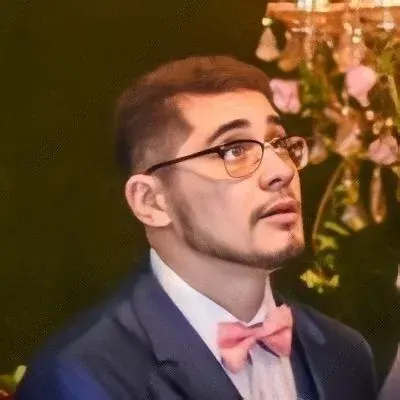
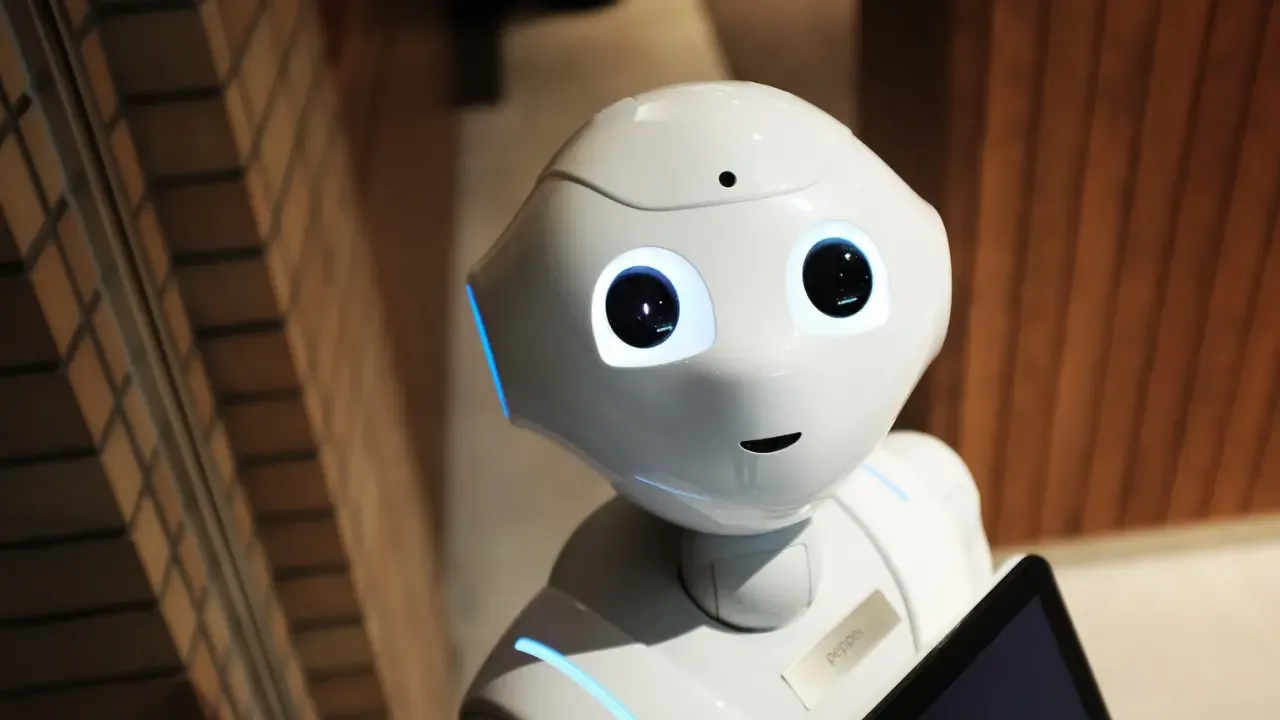
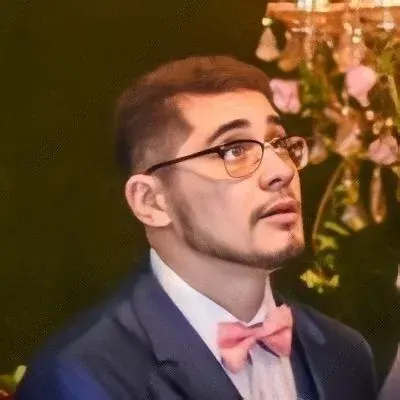
Easy Solutions for the "Origin is not allowed by Access-Control-Allow-Origin" Issue šš
š Ever encountered the pesky error message "Origin is not allowed by Access-Control-Allow-Origin"? We feel you! This error often arises when making cross-domain AJAX requests, and while it serves an essential security purpose, it can be frustrating when you're just trying to test things locally. But fear not! We've got your back with some easy solutions to fix this issue without compromising safety. š”ļø
Understanding the Problem šµļøāāļøš
š© The "Origin is not allowed by Access-Control-Allow-Origin" error occurs when a request is made from one domain (or origin) to another, and the server receiving the request doesn't have the proper CORS (Cross-Origin Resource Sharing) headers in place. CORS headers act as gatekeepers, ensuring that only trusted domains can access resources on a server.
š In your case, you're trying to send an AJAX request from your Node.js server (localhost:3000) to your Google App Engine (GAE) server (localhost:8080). However, since these two servers have different origins, the request is being blocked unless proper CORS headers are set on the GAE server.
š” keep in mind that the solutions provided here are suitable for local testing purposes. For production scenarios, it's essential to configure proper CORS policies and secure your applications.
Solution 1: Configure CORS Headers on the Server Side š„ļøš
š ļø The first solution involves configuring the CORS headers on your GAE server. By doing so, you explicitly allow requests from your Node.js server, thereby bypassing the "Origin is not allowed" error. Here's how you can do it:
Identify your server technology: Depending on your GAE server's technology (e.g., Python, Java, Node.js), the process may vary. You'll typically need to add specific headers to the server's responses.
Add the CORS headers: In your GAE server's response headers, include the following:
Access-Control-Allow-Origin: http://localhost:3000 Access-Control-Allow-Methods: GET, POST, PUT, DELETE, OPTIONS Access-Control-Allow-Headers: Content-Type
This allows requests from your Node.js server (localhost:3000) and specifies the allowed HTTP methods and headers.
Solution 2: Use a Proxy Server šš
š Another solution to overcome the "Origin is not allowed by Access-Control-Allow-Origin" error is by setting up a proxy server. This approach involves redirecting your AJAX requests through a proxy server that doesn't face CORS limitations. The proxy server, in turn, forwards the request to the destination server and returns the response to your client.
š ļø To set up a proxy server:
Install a proxy server package: You can use packages like
http-proxy-middleware
orhttp-proxy
(for Node.js) that allow you to set up a proxy server effortlessly.Configure the proxy server: Set up the proxy server to listen on your Node.js server and forward requests to your GAE server. Here's an example using
http-proxy-middleware
:const { createProxyMiddleware } = require('http-proxy-middleware'); const proxy = createProxyMiddleware({ target: 'http://localhost:8080', changeOrigin: true, pathRewrite: { '^/api': '', // remove the /api prefix from the URL }, }); app.use('/api', proxy);
This code sets up a proxy server that intercepts requests starting with "/api" and forwards them to your GAE server.
Solution 3: Use a Browser Extension šš¦
š If you're looking for a quick solution during development, consider using a browser extension that temporarily allows cross-origin requests. These extensions modify the browser's security policies to allow AJAX requests from any domain, saving you from the "Origin is not allowed" headache. Some popular extensions include Allow CORS: Access-Control-Allow-Origin for Google Chrome and CORS Everywhere for Mozilla Firefox.
š±ļø Click on the extension's icon to activate it before testing your AJAX requests. Remember, these extensions should be used carefully and only during development to avoid potential security risks.
Let's Get Your Code Working! šš»
šŖ Armed with these solutions, you'll be able to bypass the "Origin is not allowed by Access-Control-Allow-Origin" error and get your code running smoothly. Remember to consider the security implications when implementing these solutions in a production environment.
Now it's your turn! Have you encountered this error before, or do you have any other solutions to share? Let us know in the comments below, and don't forget to smash that share button to help your fellow developers debugging their CORS issues! šš