orderBy multiple fields in Angular
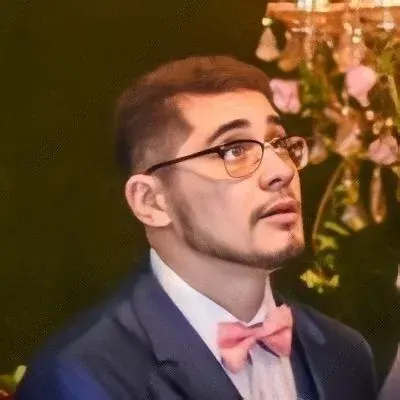
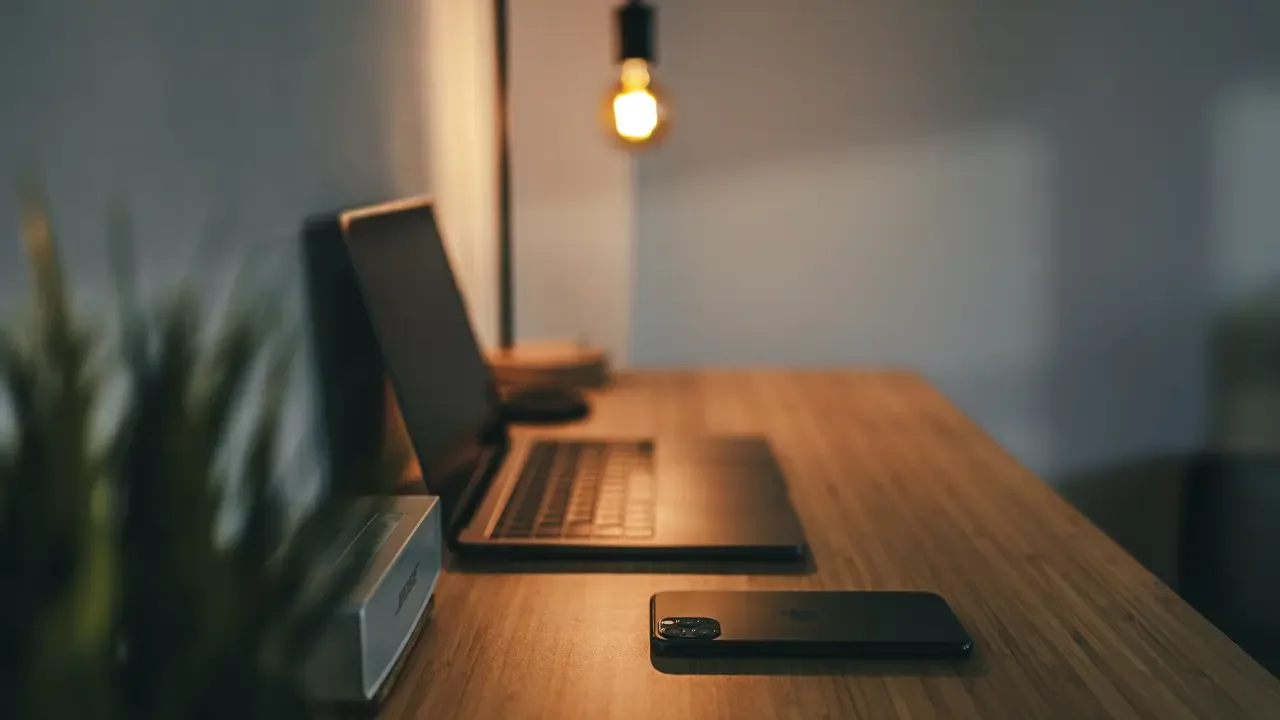
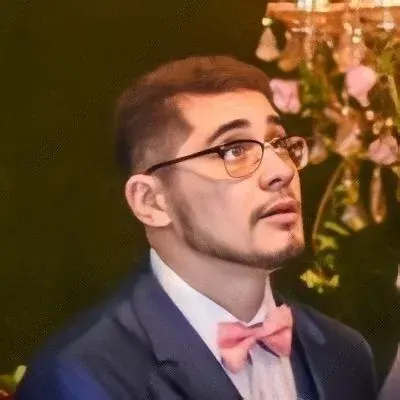
How to OrderBy Multiple Fields in Angular
Sorting data in Angular is a common operation, but what if you need to sort by multiple fields at the same time? For example, what if you have a list of divisions and you want to sort them first by group and then by sub-group? In this blog post, I'll show you a simple solution to this problem using the orderBy
filter in Angular.
The Problem
Let's consider the following example, where we have an array of divisions:
$scope.divisions = [{'group':1,'sub':1}, {'group':2,'sub':10}, {'group':1,'sub':2}, {'group':1,'sub':20}, {'group':2,'sub':1},
{'group':2,'sub':11}];
We want to display these divisions in the following format:
group : sub-group
1 - 1
1 - 2
1 - 20
2 - 1
2 - 10
2 - 11
The Solution
To achieve the desired sorting, we can use the orderBy
filter in Angular. The orderBy
filter allows us to sort an array based on the values of one or more fields.
To sort the divisions array by group and then by sub-group, we can use the following code:
<select ng-model="divs" ng-options="(d.group+' - '+d.sub) for d in divisions | orderBy:['group', 'sub']" />
In this code, we use the orderBy
filter with an array of fields to sort by. The divisions array is then passed to the ng-options
directive to populate the dropdown list. The result is a sorted list of divisions, first by group and then by sub-group, displayed in the desired format.
Conclusion
Sorting data by multiple fields in Angular doesn't have to be complicated. By using the orderBy
filter and specifying the fields to sort by, you can easily achieve the desired sorting order. Next time you need to sort data in your Angular application, remember this simple solution.
Share this blog post with your fellow Angular developers to help them solve the problem of ordering by multiple fields. And don't forget to leave a comment below if you have any questions or other Angular topics you'd like us to cover. Happy sorting! 😄🔀
Sources: