OnChange event using React JS for drop down
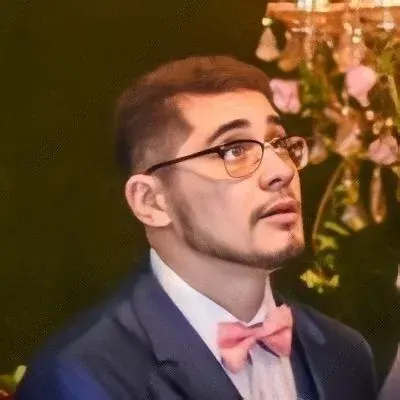
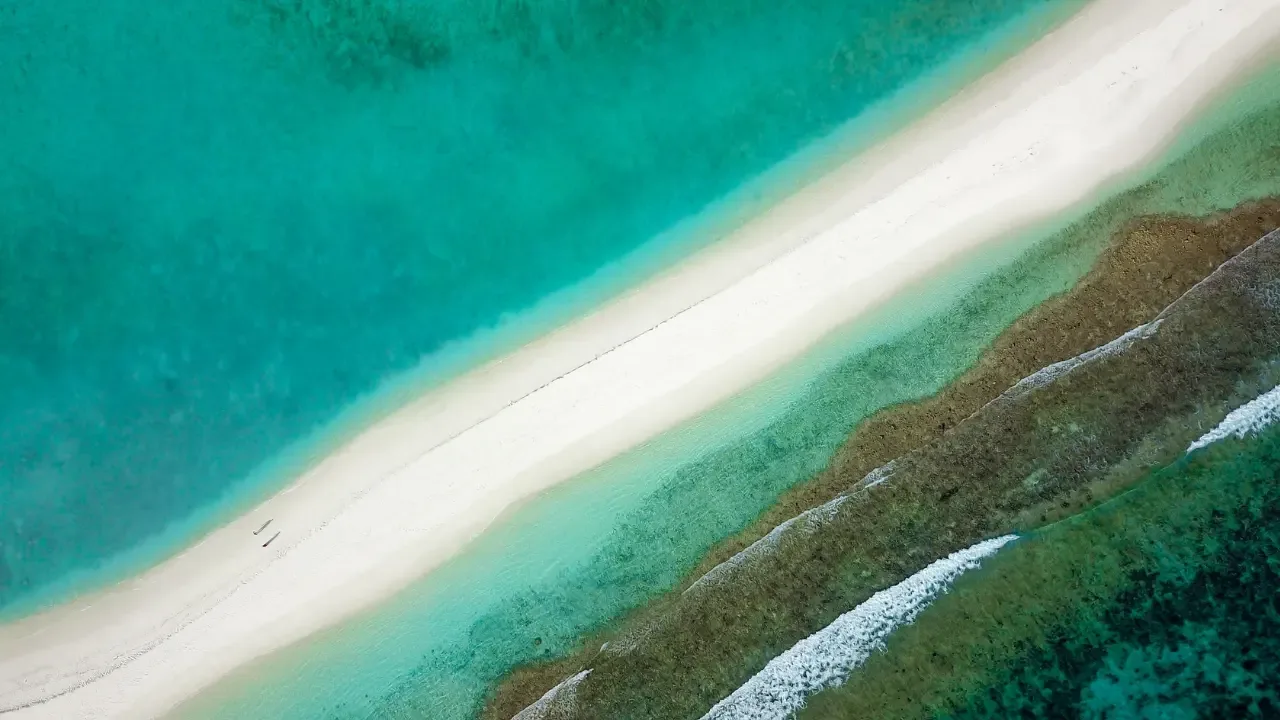
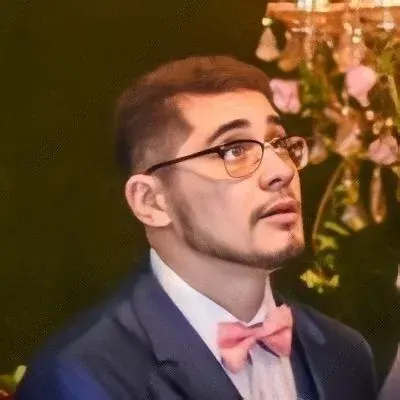
🎉 Solving the onChange Event Issue with React JS for Dropdown 🎉
So you're facing an issue with the onChange
event for a dropdown in React JS? Don't worry, we've got your back! In this blog post, we're going to address this common problem and provide easy solutions so you can get your dropdown working seamlessly. Let's dive right in! 💪
Understanding the Problem 🤔
You might have noticed that the onChange
event is not firing as expected when a selection is made in the dropdown. In the given example code, the onChange
event is placed on each option inside the <select>
element. However, this approach does not work because the onChange
event should be placed on the <select>
element itself.
The Solution 🚀
To fix the issue and make the onChange
event work for a dropdown in React JS, follow these steps:
Remove the
onChange
prop from each<option>
element and place it on the<select>
element.<select id="lang" onChange={this.change}>
Update the
change
function implementation to access the selected value from the<select>
element instead of querying it from the DOM directly.change: function(event){ return event.target.value; },
In the
<p>
tag that displays the selected value, update thevalue
prop to call thechange
function instead of accessing its value directly.<p value={this.change()}></p>
That's it! Your onChange
event for the dropdown should now be working as expected. 🎉
Example Code 💻
Here's the updated code with the necessary changes highlighted:
var MySelect = React.createClass({
change: function(event){
return event.target.value;
},
render: function(){
return(
<div>
<select id="lang" onChange={this.change}>
<option value="select">Select</option>
<option value="Java">Java</option>
<option value="C++">C++</option>
</select>
<p value={this.change()}></p>
</div>
);
}
});
React.render(<MySelect />, document.body);
Let's Get Interactive! 💬
We hope this guide helped you solve the onChange
event issue for your dropdown in React JS. If you have any further questions or face any other issues, feel free to leave a comment below. We'd love to hear from you and help you out! 😊
Now it's your turn! Share your experience with the onChange
event in React JS by commenting below. Don't forget to hit the share button and spread the knowledge with your fellow developers. Happy coding! 💻🚀✨