offsetting an html anchor to adjust for fixed header
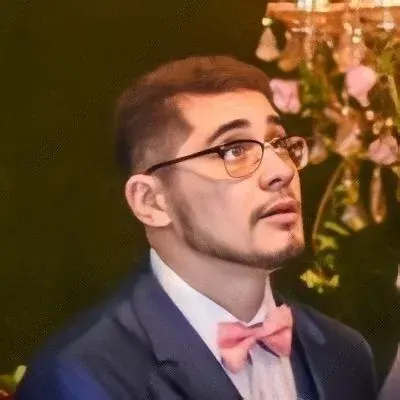
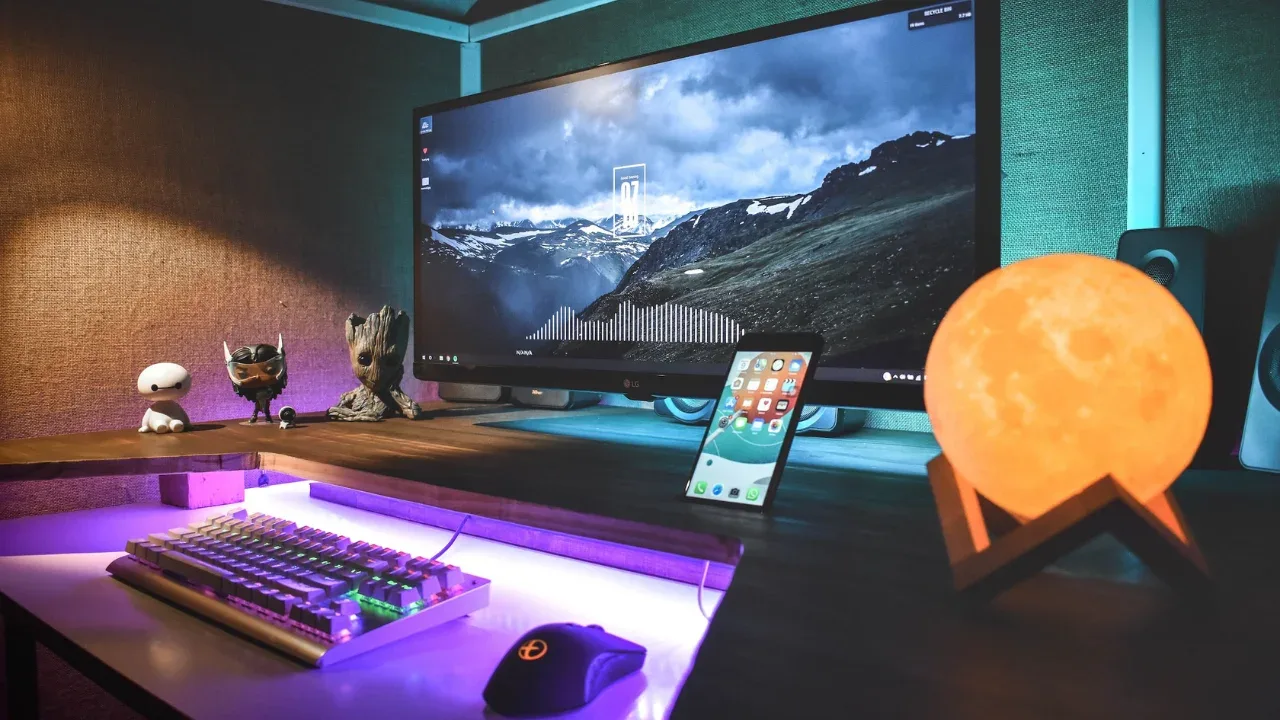
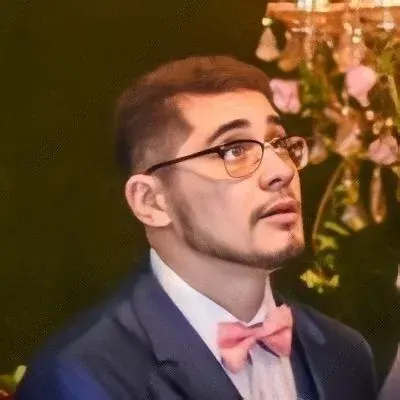
📝 Adjusting Anchors to Account for a Fixed Header
You have a beautiful website with a fixed header at the top, but when users click on an anchor link, the content is hidden behind the header. Frustrating, right? 🤔
No worries! In this blog post, we'll dive into the common issue of offsetting an HTML anchor to adjust for a fixed header. We'll explore easy-to-implement solutions using HTML, CSS, and even Javascript. Let's get started! 💪
The Problem:
You've got a fixed header, and anchor links (or internal links) within your page that, when clicked, take users to specific sections. However, due to the fixed header, the content scrolls behind it, making it difficult to read. 😞
The Solution:
To offset the anchor by the height of the fixed header (let's say 25px), we can leverage a combination of HTML, CSS, and/or Javascript. Let's explore a few approaches:
Solution 1: CSS Margin
Here's a simple CSS approach that involves applying a negative margin to the linked section to offset it:
.section {
margin-top: -25px; /* Adjust this value to match the height of your fixed header */
padding-top: 25px; /* Create space for the header so content isn't hidden */
}
In your HTML, apply the .section
class to the sections you want to link to:
<section class="section">
<!-- Content goes here -->
</section>
Solution 2: HTML Anchor Tag
Another approach involves using the anchor tag itself to offset the content:
<a id="section1" class="offset-anchor"></a>
<section>
<!-- Content goes here -->
</section>
In your CSS, add the following rule to create space for the fixed header:
.offset-anchor {
display: block;
height: 25px; /* Adjust this value to match the height of your fixed header */
margin-top: -25px; /* Same value as above */
visibility: hidden;
}
Solution 3: JavaScript ScrollTo
If you prefer a more interactive solution, you can use Javascript to scroll to the desired section and offset it programmatically:
const section = document.getElementById('section1');
const scrollOffset = 25; // Adjust this value to match the height of your fixed header
function scrollAndOffset() {
const offsetPosition = section.offsetTop - scrollOffset;
window.scrollTo({
top: offsetPosition,
behavior: 'smooth' // Add a smooth scroll effect for a better user experience
});
}
// Call the scrollAndOffset function when the anchor link is clicked
document.getElementById('yourAnchorLinkId').addEventListener('click', scrollAndOffset);
Remember to replace 'yourAnchorLinkId'
with the actual ID of your anchor link.
Conclusion:
Now you have three practical solutions to offset your HTML anchors and ensure that your content is displayed correctly, even with a fixed header. 💯
Feel free to mix and match these solutions depending on your specific needs. Apply the CSS margin solution for a quick fix, the HTML anchor tag solution for semantic markup, or the JavaScript approach for a more dynamic behavior.
If you found this guide helpful, why not share it with your developer friends? Let's solve common web development problems and create better user experiences together! 🌐💻
Got any questions, suggestions, or other anchor-related issues? Let us know in the comments below! 👇
Happy coding! 💪✨