Objects are not valid as a React child. If you meant to render a collection of children, use an array instead
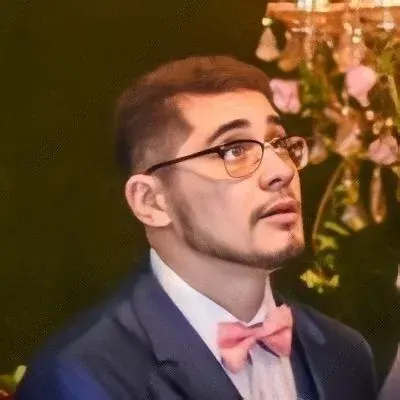
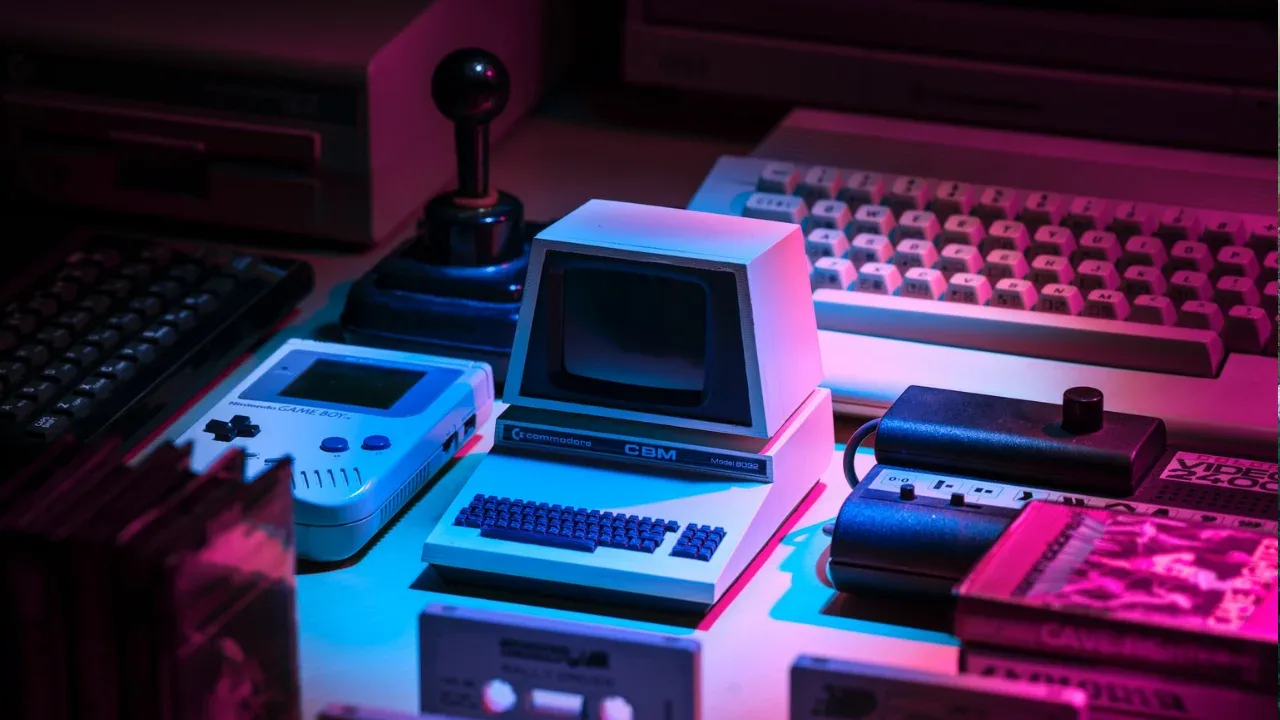
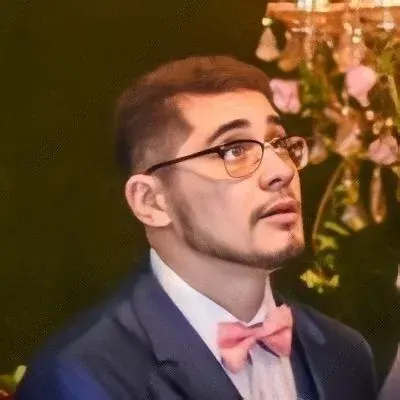
Objects are not valid as a React child: A Guide to Common Issues and Easy Solutions
If you're encountering the error "Objects are not valid as a React child," don't worry! You're not alone. This error commonly occurs when you try to directly render an object as a child in your React component. But fear not, I'm here to help you understand why this error occurs and provide you with easy solutions.
Understanding the Error
React expects components to accept and render certain types of values as children. However, objects are not one of those valid types. React's virtual DOM manipulates and updates components efficiently, and handling objects as children can lead to unexpected behavior or performance issues.
In the context of your problem, the error message specifically states that an object with specific keys (such as id
, name
, info
, created_at
, updated_at
) is being encountered. React is telling you that you need to treat this object as part of a collection and render it using an array instead.
Analyzing the Code
Let's dive into your code to understand where the error is originating from:
...
return (
<div className="col">
<h1>Mi Casa</h1>
<p>This is my house y'all!</p>
<p>Stuff: {homes}</p>
</div>
);
...
In the code snippet above, you're trying to render the homes
object directly as part of the JSX. This is causing React to throw the error because it requires an array of children, not an object.
Easy Solutions
Now that we understand the problem, let's explore some easy solutions!
Solution 1: Map the Object to JSX
One way to solve this issue is by mapping the homes
object to JSX elements within an array. This will transform each object into a separate component that React can handle. Here's an example of how you can achieve this:
return (
<div className="col">
<h1>Mi Casa</h1>
<p>This is my house y'all!</p>
<p>
Stuff:{" "}
{homes.map(home => (
<div key={home.id}>
<p>{home.name}</p>
<p>{home.info}</p>
</div>
))}
</p>
</div>
);
In the code above, we're using the map
function to iterate over each object within the homes
array. We create a separate <div>
for each home object and render the desired properties (name
and info
in this case).
Solution 2: Handle the Data Before Rendering
Alternatively, you can handle the data fetch and transformation before rendering it in the JSX. Here's an example of how you can achieve this:
...
.then(result => {
const modifiedHomes = result.map(home => ({
id: home.id,
name: home.name,
info: home.info,
created_at: home.created_at,
updated_at: home.updated_at
}));
this.setState({
isLoaded: true,
homes: modifiedHomes
});
})
...
In this code snippet, we're mapping the received result
from the API call to a modified homes
array. This ensures that each object only contains the properties required for rendering. By doing this transformation in the componentDidMount
lifecycle method, the error should be resolved and React will render the data correctly.
Conclusion
Now that you understand why the error "Objects are not valid as a React child" occurs and have easy solutions at your disposal, you're ready to tackle this issue head-on. Remember to treat objects as part of a collection by using an array and either map the objects to JSX or handle the data before rendering.
If you found this guide helpful, feel free to share it with your fellow developers who may also be struggling with this error. And if you have any questions or suggestions, let me know in the comments below. Happy coding! 😊🚀