Object comparison in JavaScript
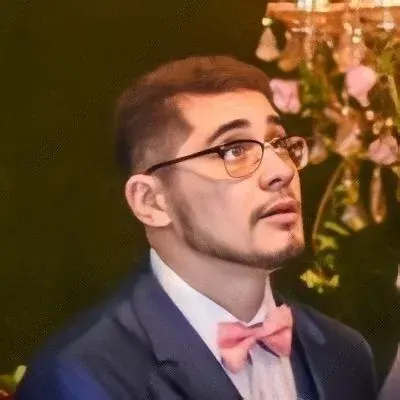
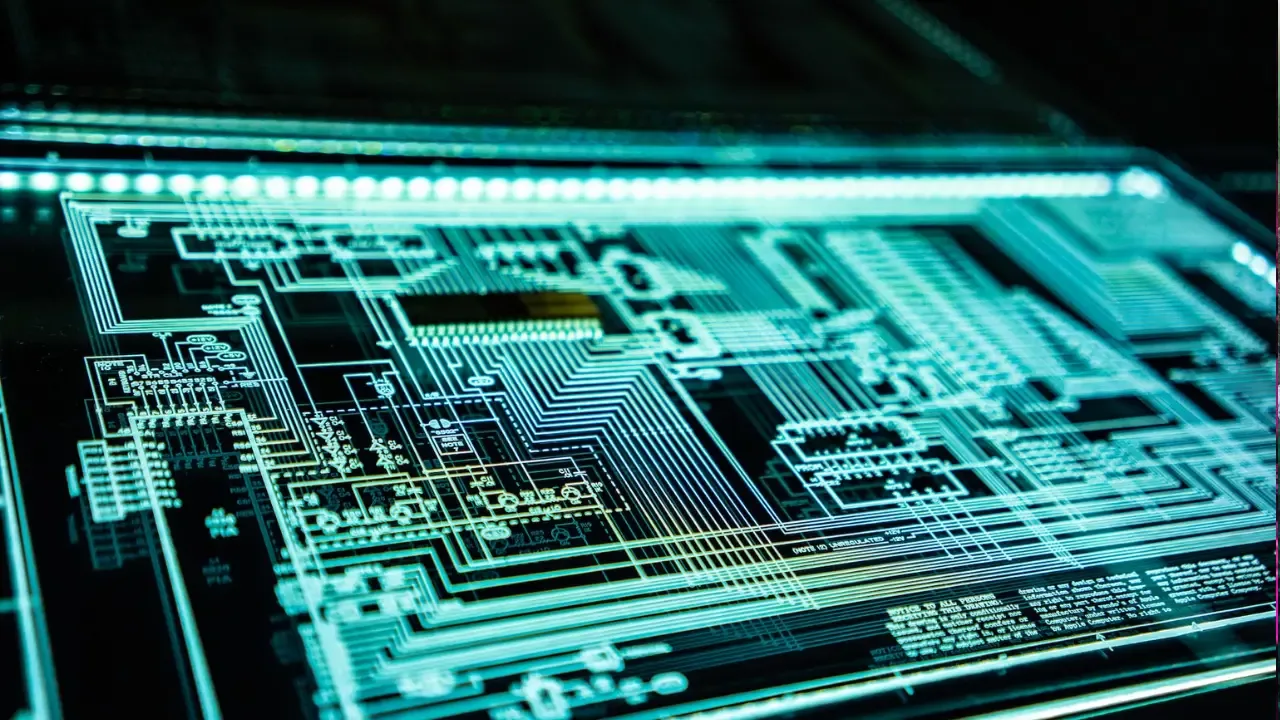
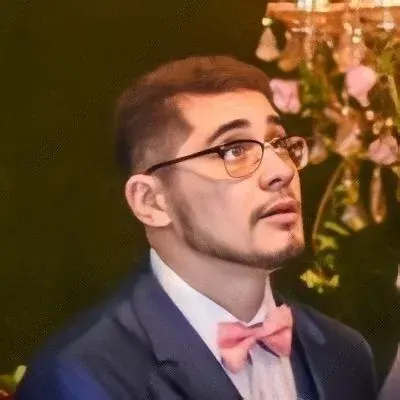
Comparing Objects in JavaScript: A Comprehensive Guide 🤝🔍
Do you find yourself struggling to compare objects in JavaScript? 🤷♂️ Are you tired of those unexpected results when comparing two objects with identical attribute values? 😫 Well, fret no more! In this blog post, we'll delve deep into the world of object comparison in JavaScript, exploring common issues and providing easy, practical solutions. 💪
Understanding the Problem 🤔
Let's start by looking at a common scenario. Say we have two objects, user1
and user2
, with identical attribute values:
var user1 = {name : "nerd", org: "dev"};
var user2 = {name : "nerd", org: "dev"};
Now, let's try comparing them using the equality operator (==
):
var eq = user1 == user2;
alert(eq); // false
Wait, what?! The equality check returns false
even though both objects have the same attribute values. 😱 This happens because objects in JavaScript are compared by reference, not by their attribute values. So, user1
and user2
are considered different objects, even though their attributes match. 😫
The Power of Object Equality 🌟
So, how can we compare objects based on their attribute values rather than their references? Here's where the power of object equality comes into play! 💥
Solution 1: JSON.stringify() Method 📝
One way to compare objects is by converting them into strings and then comparing the resultant strings. JavaScript provides us with the JSON.stringify()
method, which transforms objects into JSON strings.
Here's an example:
var eq = JSON.stringify(user1) === JSON.stringify(user2);
alert(eq); // true
By using JSON.stringify()
, we convert both user1
and user2
into JSON strings and then compare them. This comparison is based on attribute values rather than object references, as desired. 👏
Solution 2: Deep Equality Libraries 📚
While the JSON.stringify()
method works for simple object comparisons, it may fall short when dealing with complex nested objects or arrays. In such cases, using deep equality libraries can simplify the process.
Libraries like Lodash, Underscore, and Ramda provide utility functions such as _.isEqual()
and R.equals()
, which perform deep object equality checks.
// Example using Lodash
var eq = _.isEqual(user1, user2);
alert(eq); // true
// Example using Ramda
var eq = R.equals(user1, user2);
alert(eq); // true
With deep equality libraries, you can perform complex object comparisons effortlessly, regardless of the object's structure. 🙌
Embrace Object Comparison in JavaScript! ✨
Now that you've learned various approaches to compare objects in JavaScript, it's time to put your newfound knowledge into practice! 🚀 Experiment with these techniques and determine which one suits your specific requirements the best.
Additionally, if you encounter any issues while comparing objects or have any insights to share, we'd love to hear from you! Leave a comment below and join the conversation. Let's work together to make object comparison in JavaScript a breeze! 🎉
Remember, when it comes to comparing objects, don't settle for mere references – strive for attribute value equality! 💯