Node.js on multi-core machines
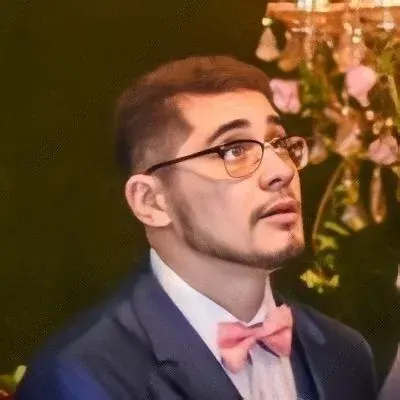
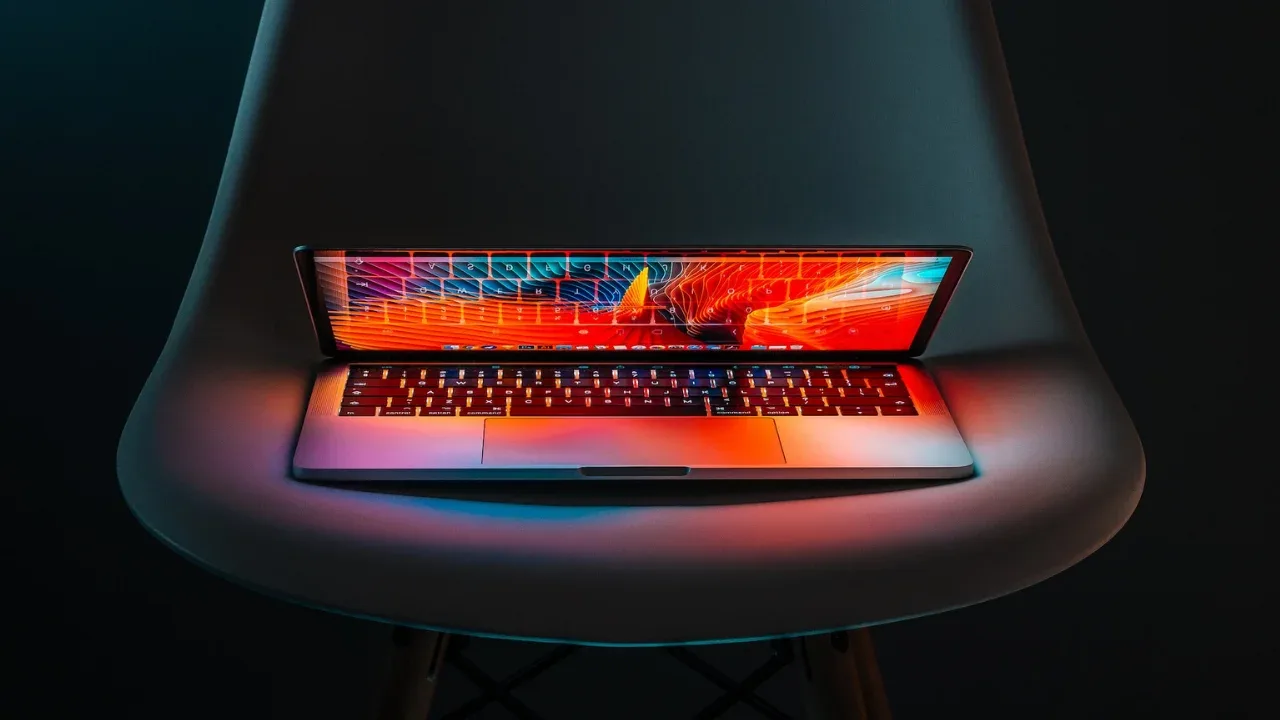
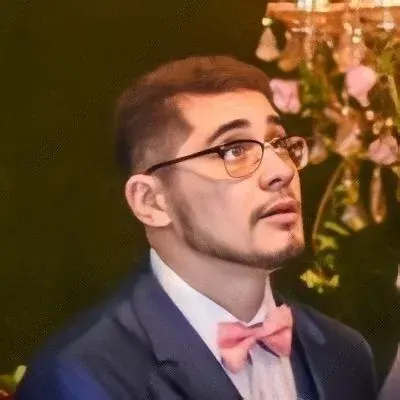
📝 BLOG POST: Node.js on Multi-Core Machines - Scaling and Parallelization Made Easy 🚀
Are you intrigued by the power and efficiency of Node.js? 🌟 But wait, isn't Node.js designed to run on a single process and thread? You might think it's not suitable for multi-core CPUs and servers, where parallelization is crucial. 🤔
Don't worry, you're not alone in wondering how Node.js handles scalability on multi-core machines. In this blog post, we'll address this common concern and provide you with easy solutions to make the most of Node.js in a multi-core environment. 💪
Understanding Node.js' Approach to Scalability 🌐
Let's set the record straight: by default, Node.js runs on a single thread. However, it doesn't mean you can't harness the power of multi-core machines. 😎
Node.js takes a unique approach called event-driven architecture, which optimizes the utilization of resources in a single thread. It utilizes non-blocking I/O operations and asynchronous programming, allowing it to handle high concurrency and large numbers of concurrent connections efficiently. 🏃♀️
Meeting the Challenge of Multi-Core Performance 🚀
So, how can we make Node.js work seamlessly on multi-core processors? 🤝 Here are a few easy solutions:
1. The Cluster Module 👥
Node.js provides a built-in module called Cluster, which allows you to create child processes (workers) that share the server's port. Each worker runs on a separate core, effectively utilizing the full power of your multi-core CPU. 🧑💼
Here's a simple example of using the Cluster module:
const cluster = require('cluster');
const os = require('os');
if (cluster.isMaster) {
const numWorkers = os.cpus().length;
for (let i = 0; i < numWorkers; i++) {
cluster.fork();
}
} else {
// Your server code here...
}
2. Load Balancing 🔄
To further optimize performance, you can implement load balancing with tools like Nginx or HAProxy. These tools distribute incoming requests across multiple Node.js instances running on different CPU cores. This way, you achieve even higher throughput and handle more simultaneous connections. 🔄
3. External Libraries and Frameworks 📚🔧
There are also external libraries and frameworks, like PM2 and StrongLoop, that make it easy to deploy Node.js applications in a multi-process and multi-core environment. They provide additional features such as process monitoring, automatic restarts, and graceful scaling. 🚀
Your Call to Action - Embrace Scalability with Node.js! 💪
Now that you understand how Node.js can scale on multi-core machines, it's time to unleash its full power and potential. Start experimenting with these solutions and find what works best for your application. 🚀
Has Node.js helped you achieve impressive scalability? Do you have any cool tips to share? Let us know in the comments below! Let's continue the conversation and help each other unlock the true potential of Node.js. 🌟
Happy coding! 💻💡