ngOnInit not being called when Injectable class is Instantiated
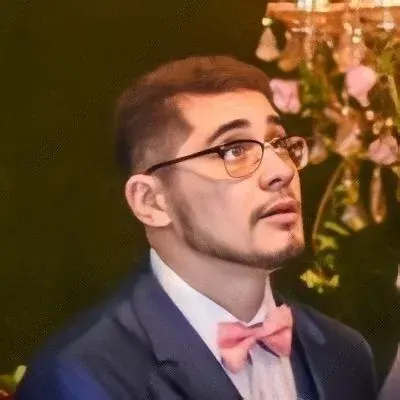
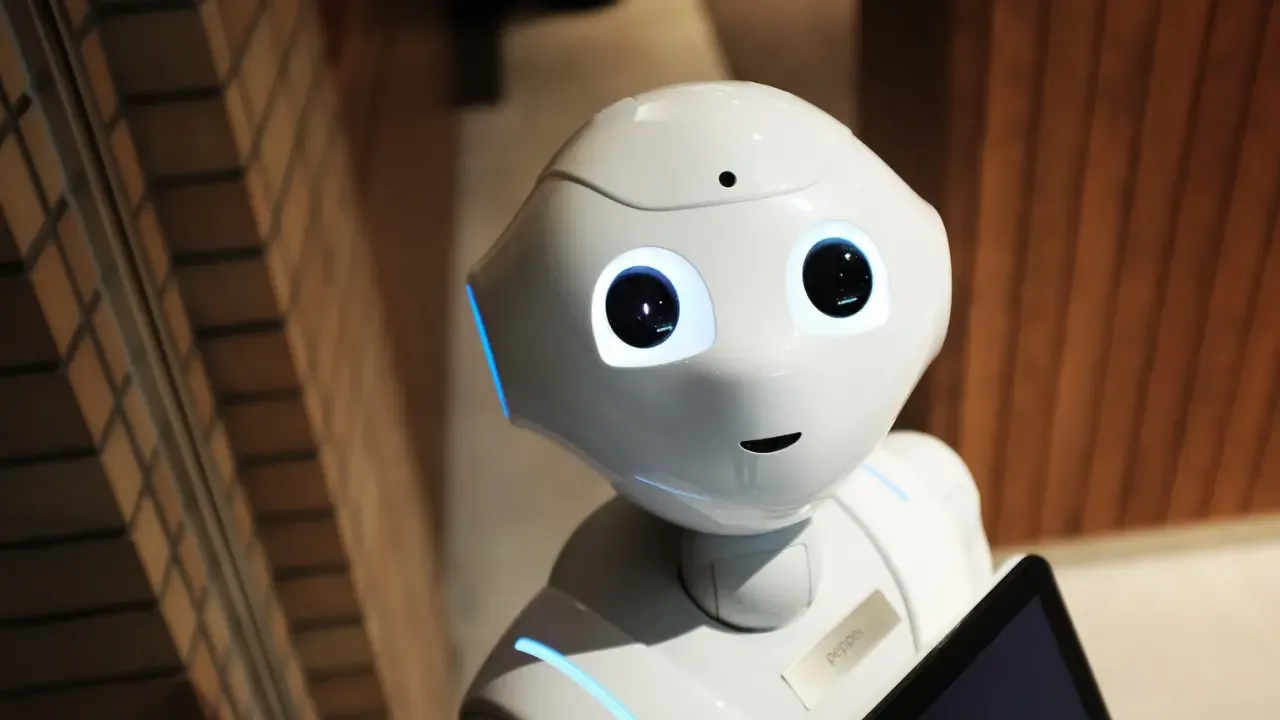
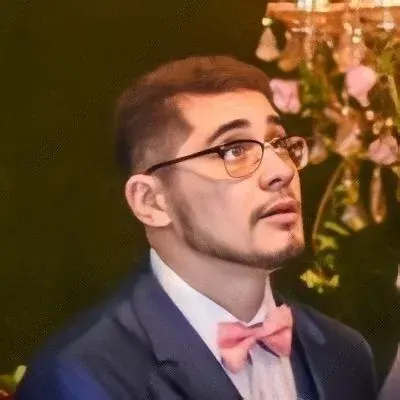
Why ngOnInit is not called when an Injectable class is Instantiated? 🤔
Have you ever encountered a situation where the ngOnInit()
function was not called even though your class implements the OnInit
interface? It can be quite frustrating, but don't worry, you're not alone! This blog post will guide you through the common issues and provide easy solutions to this problem.
Let's take a look at the code example provided:
import { Injectable, OnInit } from 'angular2/core';
import { RestApiService, RestRequest } from './rest-api.service';
@Injectable()
export class MovieDbService implements OnInit {
constructor(private _movieDbRest: RestApiService) {
window.console.log('FROM constructor()');
}
ngOnInit() {
window.console.log('FROM ngOnInit()');
}
}
📜 The Problem
The issue arises because the ngOnInit()
function is not automatically called when a class implementing OnInit
is instantiated. The ngOnInit()
function will only be called if the class is provided by Angular as a component, directive, or another injectable dependency.
🛠️ Solutions
Using a Component or Directive: If you intend to use the
ngOnInit()
function inside a component or directive, make sure you're using it within the component/directive lifecycle. Angular will handle the injection and call thengOnInit()
method for you.Manual Invocation: If you need to manually invoke
ngOnInit()
for an injectable class, you can do so by creating an instance of the class yourself and explicitly callingngOnInit()
:const movieDbService = new MovieDbService(new RestApiService()); movieDbService.ngOnInit();
Keep in mind that by manually calling
ngOnInit()
, you won't have access to the usual Angular lifecycle hooks and dependencies provided by Angular.Consider a Different Lifecycle Hook: If your intention is to perform initialization logic on class instantiation, but you don't necessarily need the
ngOnInit()
hook, you can use other appropriate Angular lifecycle hooks such asconstructor()
orngAfterViewInit()
. These hooks can be used depending on your specific use case.
📣 What's Next?
Now that you have a better understanding of why ngOnInit()
may not be called when an Injectable
class is instantiated and the possible solutions, it's time to put that knowledge into action!
If you found this blog post helpful, be sure to share it with your fellow Angular developers. Let us know in the comments if you have any other Angular questions or topics you would like us to cover. Happy coding! 💻🚀