ng-repeat finish event
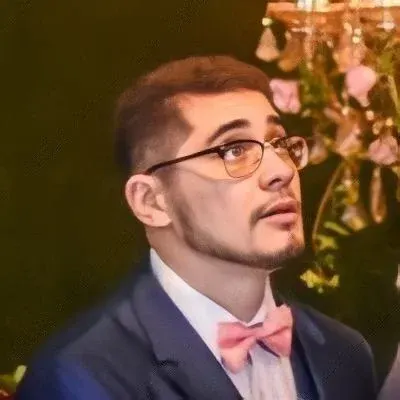
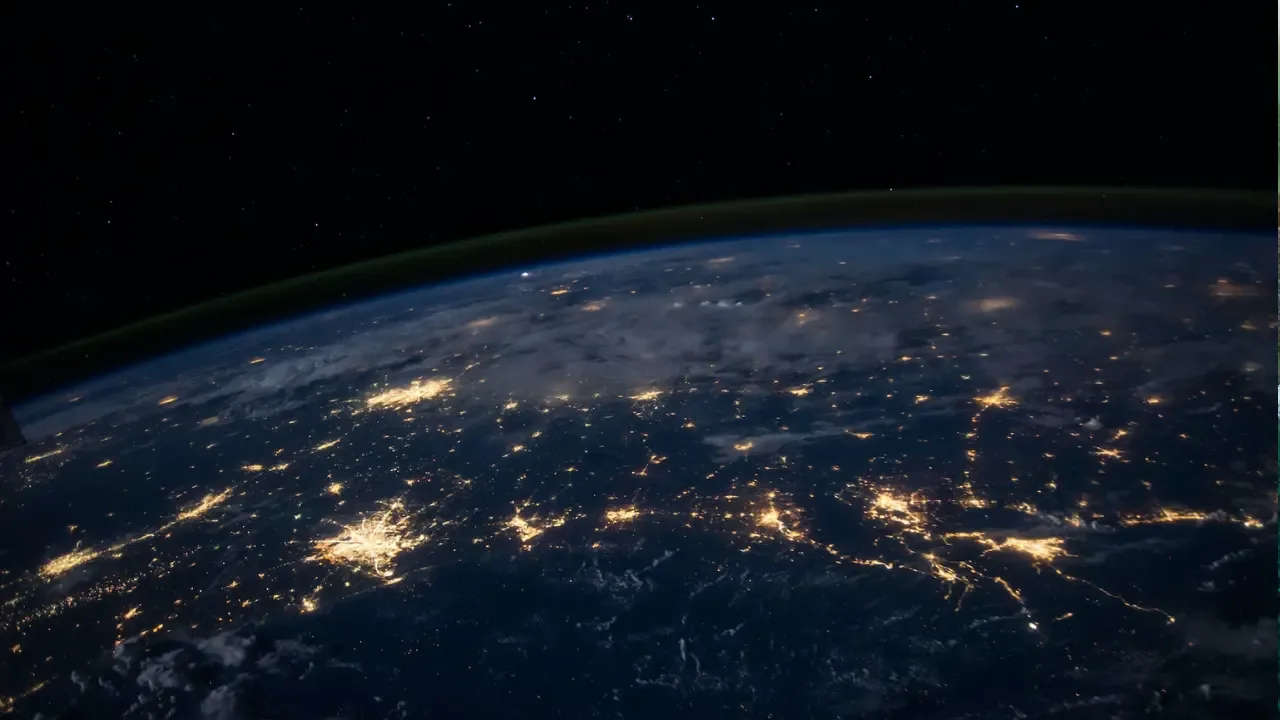
📝 Title: The Ultimate Guide to Executing a Function after ng-repeat Population Completes
Hey there tech-savvy peeps! 👋 Are you having trouble getting your jQuery function to work after populating a table using ng-repeat? 🤔 Don't fret! In this guide, we'll explore common issues, provide easy solutions, and give you a mind-blowing trick 🎩 using a custom directive so that your function runs like a charm! Let's dive in! 💻
🧩 The Common Issues
So, you've tried calling your jQuery function inside $(document).ready()
, but it's a no-go, right? 😞 And even using $scope.$on('$viewContentLoaded', myFunc)
didn't help either? 😩 We feel you! These approaches don't work because the ng-repeat population might not be finished during these events. But fear not! We've got your back with some simple solutions. 💪
💡 Easy Solutions
Solution 1: Wrapping your function call with a $timeout
A quick workaround is to use the $timeout
service provided by AngularJS. This service allows us to delay the execution of our function until the Angular digest cycle is complete, ensuring ng-repeat has finished populating your table. 🎉 Check out the code snippet below:
app.controller('MyController', function($scope, $timeout) {
$timeout(function() {
// Your function call goes here!
// Target your div with the table, and voila! 🎩✨
});
});
By wrapping your function inside $timeout
, you're guaranteed that it will be executed after ng-repeat has done its magic. Easy peasy! 🙌
Solution 2: Using the $last variable in ng-repeat
Another clever trick is to leverage the $last
variable provided by ng-repeat. This boolean variable becomes true when the current item being repeated is the last one. By checking the value of $last
, you can trigger your function call only when ng-repeat has finished. Let's take a look at an example:
<div ng-repeat="item in items" ng-init="myFunc()" ng-if="$last">
<!-- Your table and content go here! -->
</div>
In the code snippet above, we use ng-if="$last"
to ensure that the function myFunc()
is only called when ng-repeat reaches the last item in the array. This ensures that your function executes after ng-repeat finishes populating your table. Cool, huh? 😎
🎩 The Mind-Blowing Trick: Creating a Custom Directive
Now, if you're looking for a more structured and reusable solution, here's where custom directives come into play. We'll show you how to create a custom directive that executes your desired function after ng-repeat completes. Brace yourself, because it's about to get awesome! 🚀
Step 1: Define your custom directive. In this case, let's call it ngRepeatFinish
:
app.directive('ngRepeatFinish', function() {
return {
restrict: 'A',
link: function(scope, element, attrs) {
if (scope.$last === true) {
// Your function call goes here!
// Target your div with the table, and prepare to be amazed! 🌟
}
}
};
});
Step 2: Apply your custom directive in the ng-repeat element, like so:
<div ng-repeat="item in items" ng-repeat-finish>
<!-- Your table and content go here! -->
</div>
By applying the ng-repeat-finish
directive to your ng-repeat element, your function gets executed after ng-repeat has done its marvelous job. Isn't that incredible? 😲
🚀 Time to Take Action!
Now that you know all these fantastic solutions, it's time to give them a spin! Choose the one that suits you best and start executing your functions flawlessly after ng-repeat population completes. Share your success stories with us in the comments below or on social media using the hashtag #NgRepeatExecutionVictory! We can't wait to hear from you! 🎉💬
So, tech enthusiasts, let's spread the word on this ultimate guide! Share it with your friends, family, and fellow developers to help them conquer this challenge too. Together, we can make coding a breeze! 🌬️💻
Happy coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
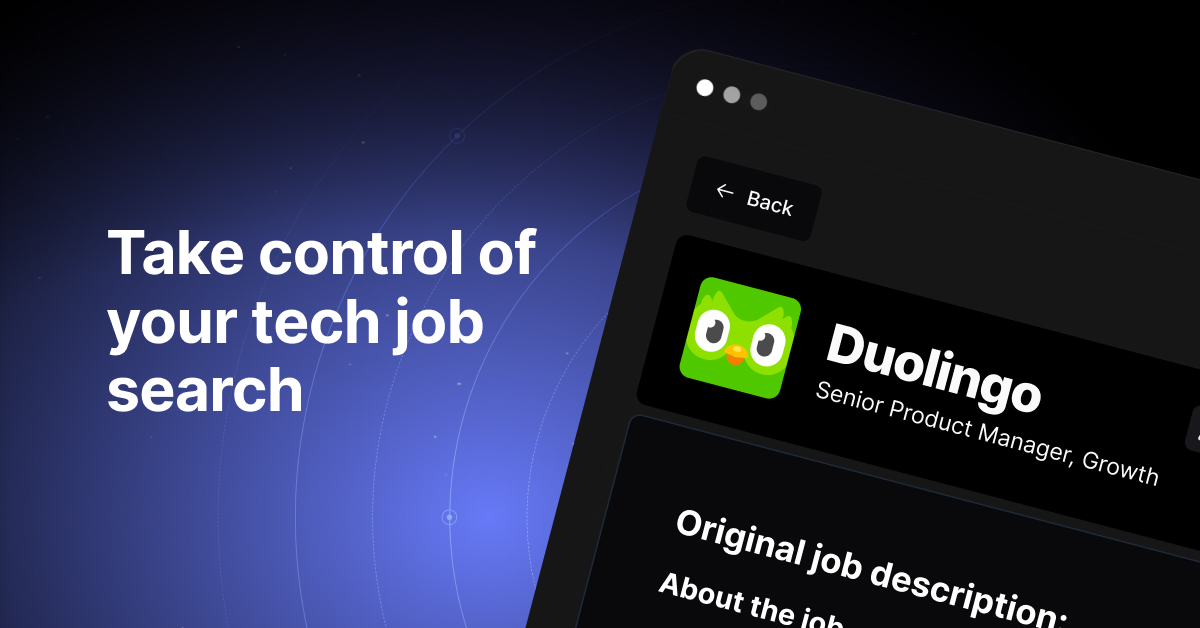