Nested routes with react router v4 / v5
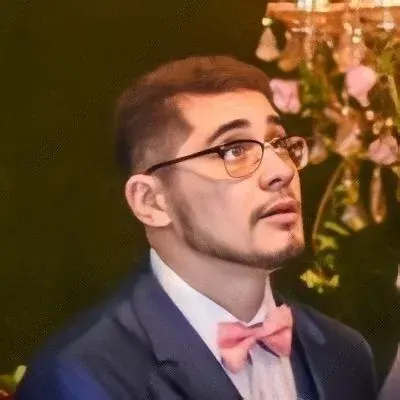
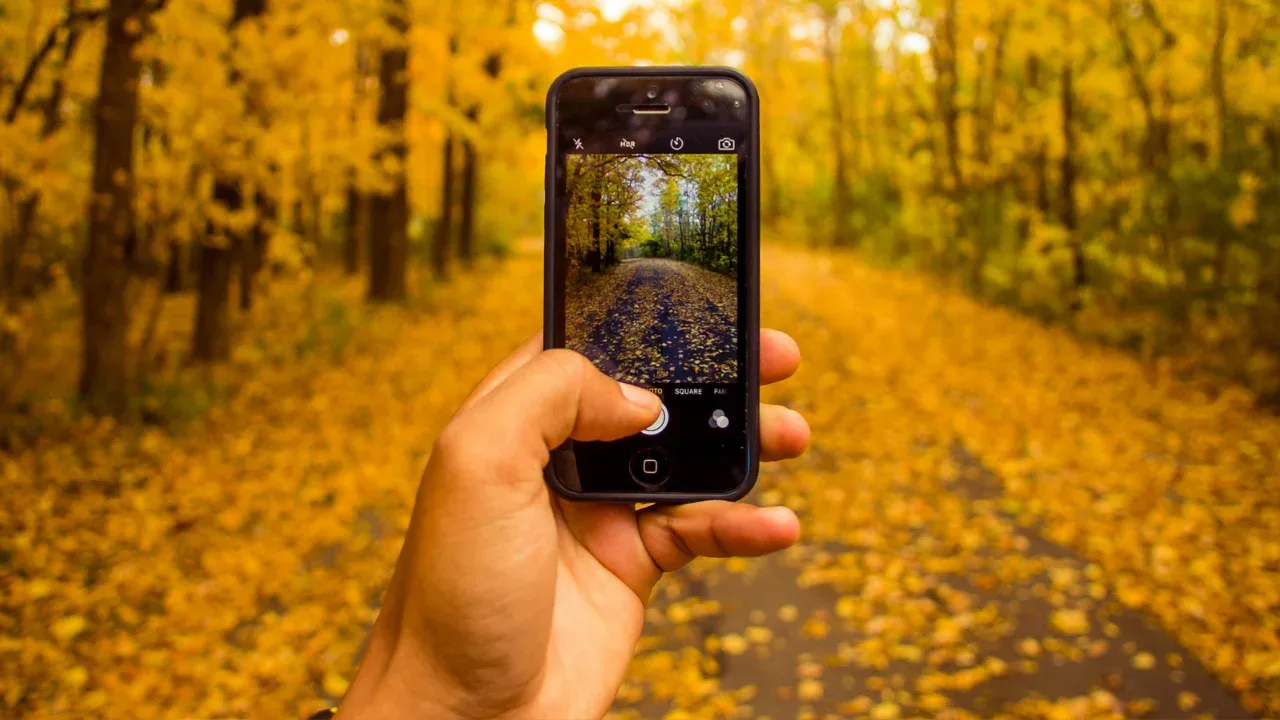
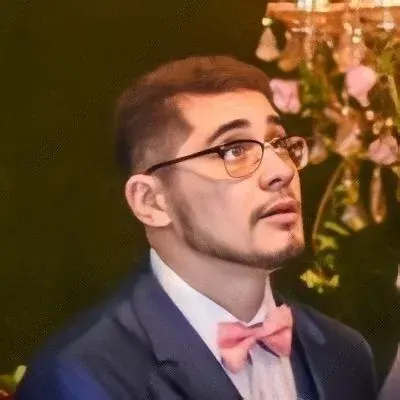
📝 Easy peasy way to nest routes in React Router v4/v5
So you're working on a React app, and you want to organize your routes by nesting them. You've checked out the React Router v4 documentation, but you can't seem to get it working the way you want. Don't worry, we've got you covered! In this guide, we'll walk you through the process of nesting routes using React Router v4/v5 and provide easy solutions to common issues.
🐣 Nested Routes Explained
Nested routes allow you to define routes within routes, creating a hierarchical structure. This is often useful when you have different sections or areas in your application that require separate layouts or functionality.
In your case, you want to split your app into two parts: a frontend and an admin area. Each part will have its own set of routes. Your desired route structure looks something like this:
<Match pattern="/" component={Frontpage}>
<Match pattern="/home" component={HomePage} />
<Match pattern="/about" component={AboutPage} />
</Match>
<Match pattern="/admin" component={Backend}>
<Match pattern="/home" component={Dashboard} />
<Match pattern="/users" component={UserPage} />
</Match>
<Miss component={NotFoundPage} />
Here's what's happening:
The
Frontpage
component is the parent component for the frontend routes.The
Backend
component is the parent component for the admin routes.The child routes are defined within the parent components using the
Match
component.When you navigate to
/home
, it should be rendered within theFrontpage
component.When you navigate to
/admin/home
, it should be rendered within theBackend
component.
🛠️ Nesting Routes Step by Step
To achieve the desired nested routes functionality, follow these steps:
Step 1: Install React Router
Make sure you have React Router installed in your project. You can install it using npm or yarn:
npm install react-router-dom
# or
yarn add react-router-dom
Step 2: Configure Your Routes
Create a file (e.g., Routes.js
) and define your routes using the Switch
component from React Router.
import { Switch, Route } from 'react-router-dom';
const Routes = () => {
return (
<Switch>
<Route exact path="/" component={Frontpage} />
<Route path="/home" component={HomePage} />
<Route path="/about" component={AboutPage} />
<Route exact path="/admin" component={Backend} />
<Route path="/admin/home" component={Dashboard} />
<Route path="/admin/users" component={UserPage} />
<Route component={NotFoundPage} /> // Put the 404 route at the end
</Switch>
);
};
export default Routes;
Step 3: Integrate Routes into App Component
In your App
component, import the Routes
component and render it.
import React from 'react';
import { BrowserRouter as Router } from 'react-router-dom';
import Routes from './Routes';
const App = () => {
return (
<Router>
<div className="app">
<Routes />
</div>
</Router>
);
};
export default App;
That's it! You've successfully nested your routes using React Router v4/v5. Now, when you navigate to /home
, it will be rendered within the Frontpage
component, and when you navigate to /admin/home
, it will be rendered within the Backend
component.
🚀 Call-to-Action: Share Your Experience!
We hope this guide helped you tackle the challenge of nesting routes using React Router v4/v5. If you found it useful, share it with your fellow developers and spread the knowledge! Let us know in the comments if you have any questions, or if you have any other topics you'd like us to cover. Happy coding! 😄👩💻👨💻
</br>